How to Implement Progress Bar in Python
-
Use the
tdqm
Module in Python to Create Progress Bars -
Use the
progressbar
Module in Python to Create Progress Bars - Create Your Own Function in Python to Create Progress Bars
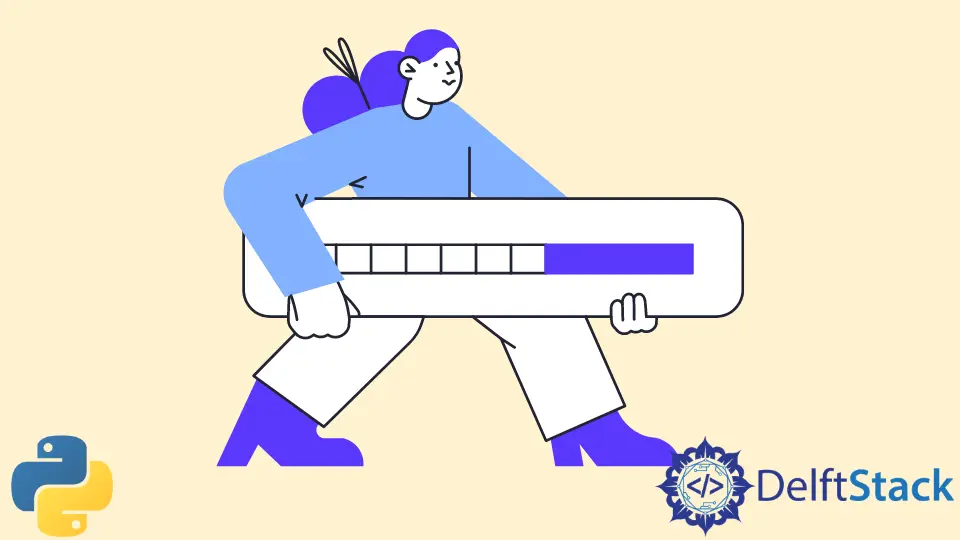
In the world of programming, we work with large volumes of code. A program might take a lot of time to compile and run. Therefore, it is a little helpful to know how much progress has been made and the estimated time of completion of the program. For such situations, we can use progress bars in Python. Moreover, they can help us identify if our program has stopped in the middle or not.
In Python, we have many libraries available which can help us to create a simple progress bar for our program to help us with this. We will discuss each method one by one in this tutorial.
Use the tdqm
Module in Python to Create Progress Bars
The tdqm
library has a swift and efficient framework for creating basic progress bars. It works well on terminal, python notebooks and is compatible with Windows, Linux, and macOS.
In the following code, we create a simple progress bar using functions from this module.
from time import sleep
from tqdm import tqdm
for i in tqdm(range(10)):
sleep(0.2)
We iterate through a loop, and on every iteration, the progress bar is also incremented. The sleep()
function is used to pause the compiler for some specified seconds. Note that by default, the bar shows the time remaining, iterations per second, and percentage.
Out of all the modules discussed in this tutorial, this one has the least overhead.
Use the progressbar
Module in Python to Create Progress Bars
The progressbar
module does almost everything like the tdqm
module. In addition to this, it has options to animate your progress bar and use widgets displayed on the progress bar.
The following code shows an example of this.
import time
import progressbar
for i in progressbar.progressbar(range(100)):
time.sleep(0.02)
The progressbar2
also exists in Python and is a fork of the progressbar
. It is extremely popular due to its powerful and customizable features. It also supports print statements with the progress bar. On the downside, this module hasn’t been maintained in years.
Note that more libraries are available like alive-progress
, progress
that can help make the progress bars, but these two are the most frequently used. The only difference between them is the animations and other customizations which these libraries offer.
Create Your Own Function in Python to Create Progress Bars
We can also create a simple function that can resemble a progress bar. The code below implements this.
import time
import sys
def progressbar(it, prefix="", size=60, file=sys.stdout):
count = len(it)
def show(j):
x = int(size * j / count)
file.write("%s[%s%s] %i/%i\r" % (prefix, "#" * x, "." * (size - x), j, count))
file.flush()
file.write("\n")
show(0)
for i, item in enumerate(it):
yield item
show(i + 1)
file.write("\n")
file.flush()
for i in progressbar(range(15), "Computing: ", 40):
time.sleep(0.1)
Output:
Computing: [........................................] 0/15
Computing: [##......................................] 1/15
Computing: [#####...................................] 2/15
Computing: [########................................] 3/15
Computing: [##########..............................] 4/15
Computing: [#############...........................] 5/15
Computing: [################........................] 6/15
Computing: [##################......................] 7/15
Computing: [#####################...................] 8/15
Computing: [########################................] 9/15
Computing: [##########################..............] 10/15
Computing: [#############################...........] 11/15
Computing: [################################........] 12/15
Computing: [##################################......] 13/15
Computing: [#####################################...] 14/15
Computing: [########################################] 15/15
We calculate the total iterations required, and over every iteration, we increment the #
symbol and print it.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn