How to Create a Port Scanner in Python
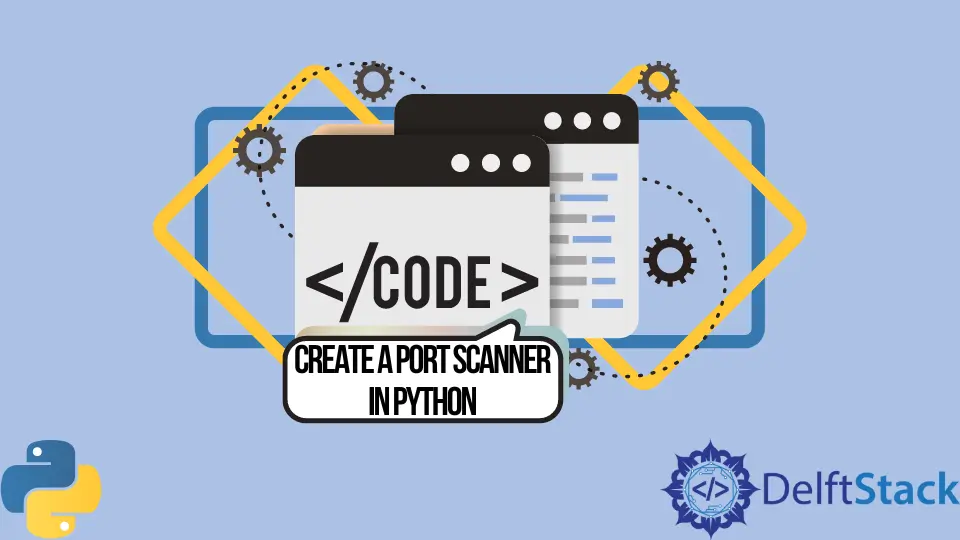
This tutorial will demonstrate how to create a port scanner in Python.
Create a Port Scanner in Python
First, let us learn the basics of a port. A port is an endpoint in a network via which some information is communicated between a server and client.
A port scanner is a simple application that allows us to determine which ports are open for communication on a given host.
Using a port scanner, we can determine which ports are open for connection and know about the ports’ running services. This type of tool helps test the security measures against potential penetration attacks.
Information is exchanged over the internet, generally using the TCP and UDP protocols. Ports range in these protocols from 0 to 65535 and are divided into three ranges.
The system ports range from 0 to 1023, user ports from 1024 to 49151, and every port above this is a dynamic port.
With a port scanner, we will individually check every port for connection and whether the connection is successful. Let us discuss how to achieve this in Python.
-
We will import the
socket
module. -
We will create a
socket
object and attempt to connect to ports for a given host server. -
We will employ a
for
loop to iterate over the range of ports and attempt a connection for each port individually. -
We will create a function to test the connection and use a
try
andexcept
block to returnTrue
andFalse
based on connection success. -
In the
try
block, we will attempt to create a connection using thesocket.connect()
function. If the connection is successful, the block will returnTrue
. -
If the connection is unsuccessful, an exception will be raised, caught in the
except
block, andFalse
will be returned.
This is implemented in the following code.
import socket
t = "https://www.hackthissite.org/"
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
def check_port(port):
try:
c = s.connect((t, port))
return True
except:
return False
for a in range(200):
if check_port(a):
print("Port", a)
The above code will check the first 200 ports to see if any ports are open for connection. Note that we should only check for hosts that permit us to do so.
We can modify our simple port scanner and add some exciting features. We can use the socket.settimeout()
function to set the timeout by providing the time in the function.
The connection is aborted if it takes more time than the one set by this function.
Another way to enhance the performance of the port scanner in Python is by using the concept of multithreading. The threads
module in Python can be used to create and manage threads that can give the application more concurrency and improve the execution time.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn