Pointers in Python
-
Use the
id()
Function to Return the Memory Address of an Object in Python -
Use the
ctypes.pointer()
Function to Create a Pointer Variable in Python
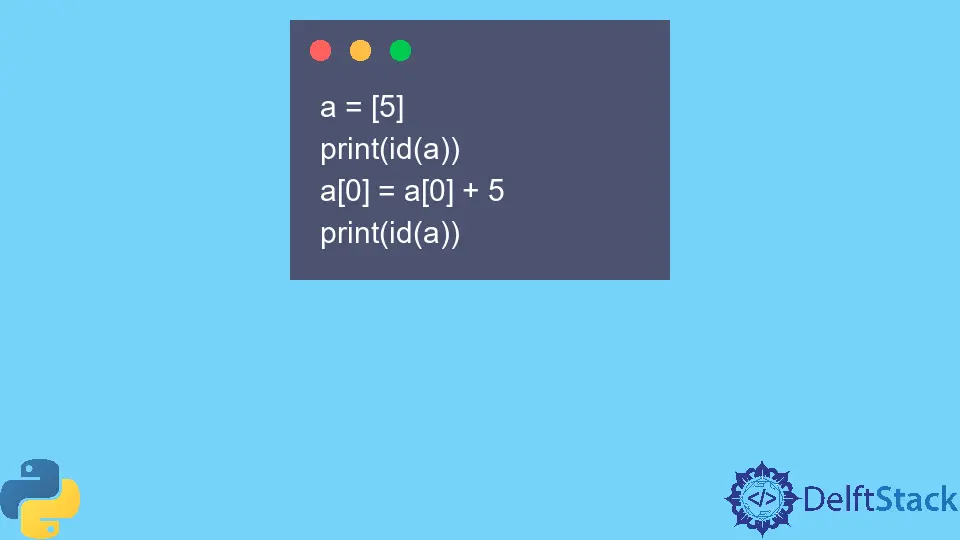
C and C++ have an interesting feature of a pointer. Pointers are variables that can store the memory address of an object.
The *
operator is used to create pointers. The same operator can be used to access the values at given addresses.
However, in Python, there is no such feature. This tutorial will discuss why this feature is not present in Python and how we can emulate them to some extent.
Although a very useful tool, pointers can be a little hard to grasp for beginner-level programmers. It can also lead to different errors related to memory management.
It can cause various issues and may lead to situations where we access something which wasn’t meant to be.
It goes against the Zen of Python, which suggests simple is better than complex, explicit is better than implicit.
Everything is an object in Python and can be immutable or mutable. Mutable objects can be changed as the code progress, whereas we cannot alter the immutable objects.
Lists, sets, dictionaries are the mutable objects in Python. Objects like int
, float
, str
, tuples
, and boolean
are immutable.
We create new objects whenever we make any changes to the immutable objects. We can verify this in the code below.
Use the id()
Function to Return the Memory Address of an Object in Python
a = 5
print(id(a))
a = a + 2
print(id(a))
Output:
140731589698064
140731589698128
In the above example, the id()
function returns the memory address of an object. You can observe the memory address before and after making the variable a
.
This is why it is not feasible to have pointers in Python. Another reason is the difference between memory allocation between variables in C/C++ and Python.
In C/C++, when we create a variable, some memory is allocated for the variable, and the value is assigned to this location.
The variable name points to this allocated memory. However, in Python, it is not so straightforward.
In Python, a new PyObject
is created, and then the typecode
for the given object is assigned. The value for this object is provided, and the variable name points to this object.
The refcount
of this object is increased by 1. On making changes to an immutable object type, a new object is created, and the refcount
of the previous object is decreased by 1
.
With the mutable objects, it is possible to replicate some easy functions of pointers.
For example, if we take our previous example, a new object is created by incrementing an integer. If we wish to avoid this, we can use a list.
A list is mutable. So we can store the value in a list and update it.
a = [5]
print(id(a))
a[0] = a[0] + 5
print(id(a))
Output:
2063886567688
2063886567688
In the above example, we store some value in a list
. Then, we modified this value by incrementing it by 5
.
However, we can observe that the memory location is the same after updating the value.
Use the ctypes.pointer()
Function to Create a Pointer Variable in Python
Another way we can emulate pointers in Python is by using the ctypes
module. This is a very complicated module but provides functionalities to load C-libraries and wrap Python around it.
import ctypes
a = ctypes.pointer(ctypes.c_int(5))
print(a)
Output:
<ctypes.wintypes.LP_c_long object at 0x000001E0894E66C8>
In the above example, you can observe that we created an integer-pointer variable using the ctype
module.
One has to load downloaded libraries with this module to use different functionalities.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn