The Pillow Package in Python
- Use the Pillow Package to Read and Display Images in Python
- Use the Pillow Package to Get the Attributes of the Image in Python
- Use the Pillow Package to Edit and Process Images in Python
- Conclusion
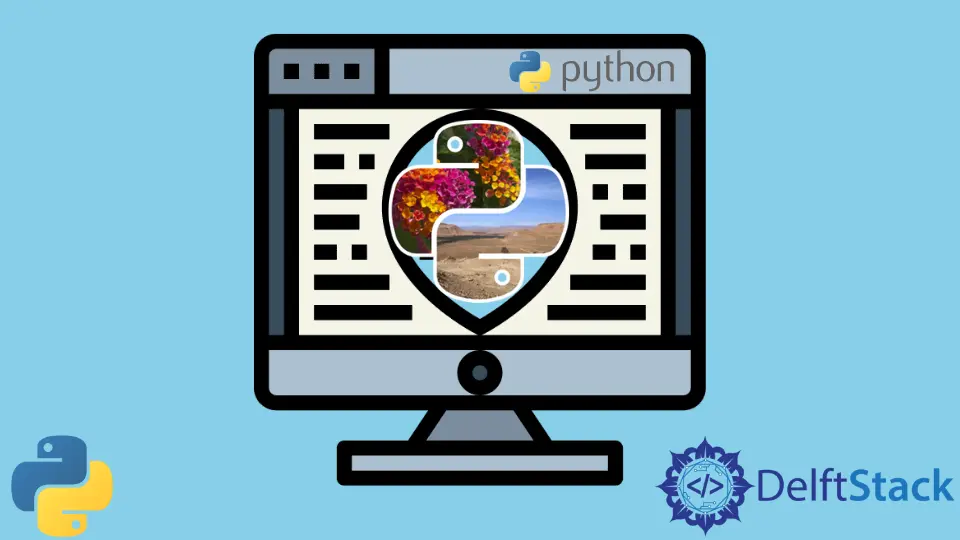
We can read, process, and display images using different libraries in Python. We had the PIL (Python Imaging Library) package, the standard image processing library, before deprecating in 2011.
After a while, a Pillow project was introduced, a fork of the original PIL package. This library included the support for all the new image formats, and new decoders were added to work with these new formats.
We install this library by executing the pip install pillow
command.
This tutorial will demonstrate the basics of the Pillow library in Python.
Use the Pillow Package to Read and Display Images in Python
We can open an image and store it in the PIL class object using the open()
function. We specify the file’s path and use it with the PIL.Image
object.
Using the show()
function, we can display the image in the PIL.Image
object. After this, the image will be stored in the buffer and converted to a PNG format to show it.
See the code below.
from PIL import Image
i = Image.open("image.png")
i.show()
Output:
In the above example, we read an image using the open()
function and displayed it with the show()
function.
Use the Pillow Package to Get the Attributes of the Image in Python
We can also get different image attributes using the PIL.Image
object.
The mode attribute tells about the depth and type of the loaded image as determined by the pixels. Modes can be L (greyscale), P (mapped to other modes), RGB, and more.
Example:
from PIL import Image
i = Image.open("image.png")
print(i.mode)
Output:
RGBA
We can use the convert()
function to convert images to different modes and specify the mode within.
We can also find the size and format of the image using the size
and format
attributes, respectively.
See the code below.
from PIL import Image
i = Image.open("image.png")
print(i.size, i.format)
Output:
(640, 640) PNG
Use the Pillow Package to Edit and Process Images in Python
We can also process the image and edit it using the Pillow package. It has a module called PIL.ImageFilter
that stores different filters.
We can apply them to images using the filter()
function and specify the filter within this.
Example:
from PIL import Image, ImageFilter
i = Image.open("image.png")
edited = i.filter(ImageFilter.BLUR)
edited.show()
Output:
In the above example, we blurred an image using the ImageFilter.BLUR
in the filter()
function.
Moreover, this package’s crop()
function can crop images to the given dimensions. And the rotate()
function can rotate the image by the provided angle.
See the code below.
from PIL import Image, ImageFilter
i = Image.open("image.png")
t = i.crop((100, 100, 350, 350))
edited = t.rotate(90)
edited.show()
Output:
We mentioned the dimensions for the cropped image and rotated it by a given angle.
In addition, the save()
function saves the edited images.
We mention the path and filename for the image in the function. We should also specify the format of the image in the filename.
Example:
from PIL import Image, ImageFilter
i = Image.open("image.png")
t = i.crop((100, 100, 350, 350))
edited = t.rotate(90)
edited.save("edited.png")
Conclusion
To wrap up, we discussed different uses of the Pillow package, its history, how it came to be after the PIL package, and some primary use in reading and processing images.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn