How to Parse JSON Array of Objects in Python
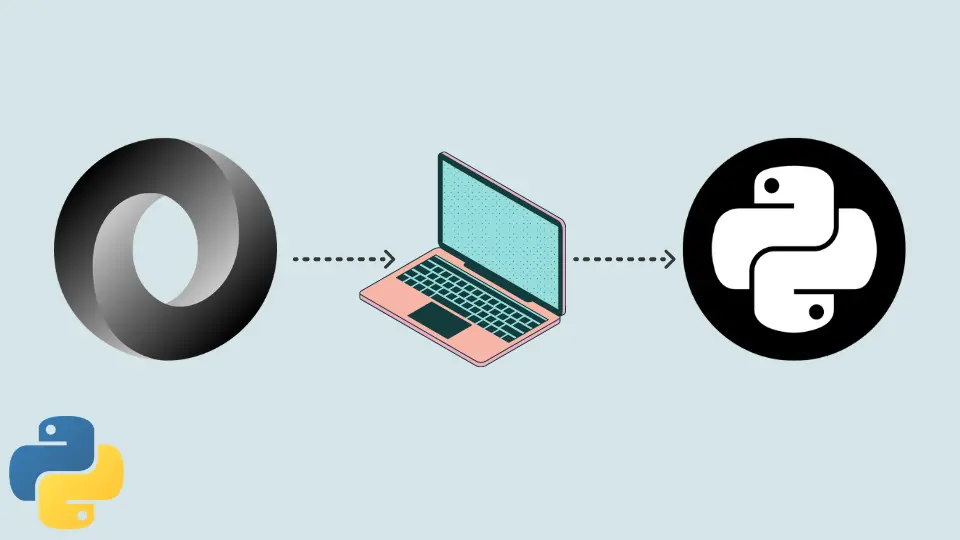
As browsers can swiftly parse JSON objects, they help transfer data between a client and a server.
This article will describe how to transmit and receive JSON data using Python’s JSON module.
JavaScript Object Notation
JSON (JavaScript Object Notation
) is a syntax for data exchange that is simple to read and write by people, simple to parse and produce by computers and store data as well.
It is a full-text format that is unbiased toward language. In addition, python includes a library named json
that we can use to interact with JSON data.
JSON’s syntax is regarded as a part of JavaScript’s syntax, which also includes name
and value
as name
is preceded by a colon (:
) in the representation of data, and name: value
pairs are split by comma.
Objects are in curly brackets, while array elements live in square brackets where a comma separates each value. Python will need some JSON to work with before you begin to parse JSON.
There are a few things we must initially set up. Make a Python file first that will contain the code for these exercises. Then, import the JSON module within the file.
JSON Objects in Python
As browsers can swiftly parse JSON objects, they help transfer data between a client and a server. Strings, integers (floats or ints), Boolean values, lists, null, or another JSON object all are acceptable values for JSON object keys.
Here in the below example, an object json_string
is created which is populated by a dictionary, the data in the object will be parsed by using the json.load()
method and then printing the data shown in the output.
Example Code:
import json
json_string = """
{
"Student": {
"ID" : "3",
"name": "kelvin",
"Group": "A",
"Program" : "BSSE"
}
}
"""
data = json.loads(json_string)
print(data)
Output:
{'Student': {'ID': '3', 'name': 'kelvin', 'Group': 'A', 'Program': 'BSSE'}}
Parse JSON Array of Objects in Python
The structure of JSON arrays is identical to that of Python bracketed lists. They may include nested arrays and the same data types as the JSON object field values. Use the json.loads()
function of the built-in package json
to transform a JSON string into a Python object.
The JSON data string is parsed by the json.loads()
function, which then provides a Python dictionary with all of the data from the JSON. You may get parsed data from this Python dictionary by using names or indexes to refer to objects.
We can also examine the dictionary for nested JSON items. Use the associated method json.load()
to parse a JSON file (without the s
). we have used json.loads
for parsing the values in the array in the below example.
Example Code:
import json
json_string = """
{
"Student": {
"ID" : "3",
"name": "kelvin",
"Group": "A",
"Program" : "BSSE",
"cars": [
{"model": "BMW 330e", "mpg": 143},
{"model": "Rolls-Royce Phantom EWB", "mpg": 18}
]
}
}
"""
data = json.loads(json_string)
print(data)
Output:
{'Student': {'ID': '3', 'name': 'kelvin', 'Group': 'A', 'Program': 'BSSE', 'cars': [{'model': 'BMW 330e', 'mpg': 143}, {'model': 'Rolls-Royce Phantom EWB', 'mpg': 18}]}}
This example transforms a JSON array into a Python array data type via the JSON Decoder. Using its index in a Python object may obtain an element from a JSON array.
Example Code:
import json
json_str = (
"""
{
"Orders": [
{"Id": 151},
{"Id": 120},
{"Id": 131},
{"Id": 114}
]
}
"""
""
)
data = json.loads(json_str)
order_1_id = data["Orders"][0]["Id"]
order_2_id = data["Orders"][1]["Id"]
order_3_id = data["Orders"][0]["Id"]
order_4_id = data["Orders"][1]["Id"]
total = len(data["Orders"])
print(f"Order #1: {order_1_id}, Order #2: {order_2_id}, Total Orders: {total}")
Output:
Order #1: 151, Order #2: 120, Total Orders: 4
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn