OpenCV Webcam
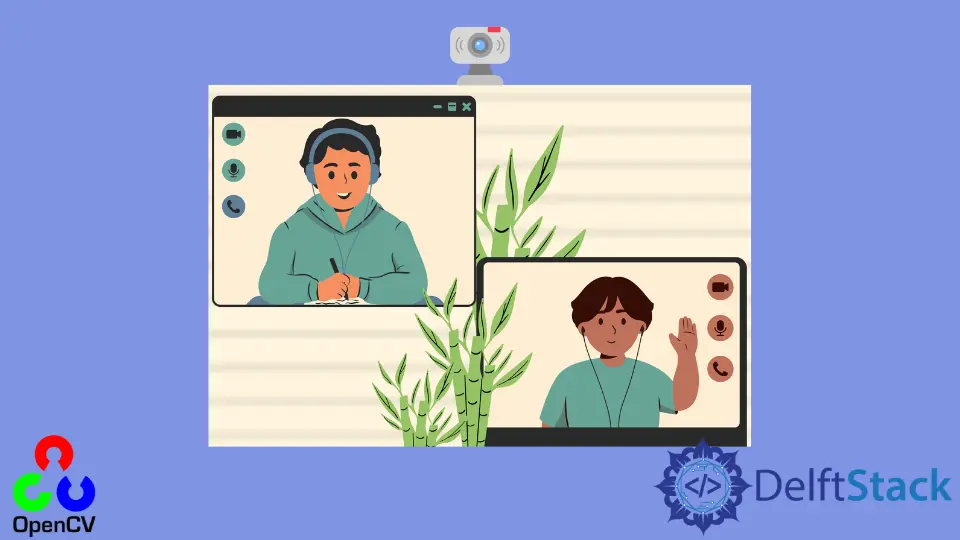
In this short demonstration, we learn how to access a webcam and display that live feed with the help of Python and OpenCV.
Access Webcam With the Help of Python and OpenCV
Whenever we are working on any computer vision project where we need to access live camera feeds, if we want to do the face mask detection, or we design a project where we want to do the social distance detection.
In that case, we have to access the live feeds of our camera, and whatever live feeds we receive from our camera, then the next instruction may be used for different purposes.
Let’s see the code to access the real-time camera feed with OpenCV. Once we import the opencv
library, we must use the VideoCapture()
method from the opencv
library.
It captures the video from different sources. It can capture a video from your local computer system or a video from your laptop’s camera, or you can capture video from any IP-based camera.
We have to provide the source of video capture in the parenthesis.
WC = cv2.VideoCapture(0)
Now you can see we provided source 0
, which means it will access our laptop’s default camera. If we want to access any video stored in the computer system, we can pass the full path of our video as a string.
If you want to access any IP-based camera, you have to provide the full path of your IP-based camera. We consider a dummy address, and it would be something like this.
WC = cv2.VideoCapture("https://3.4.5.6/cam2")
But in this article, we will try to access the default camera of our laptop, and to this, we need to put the source as 0
. It will capture the live feeds from the camera.
Once we have captured the video, we store it in an object, and after that, we will try to read each frame from the video or the live feed we are receiving from the camera.
The video is nothing but a sequence of images, and in the computer vision field, we call that frames.
Now we will write a bunch of code in the while
loop; it will infinitely keep reading the live feeds of a camera. We read the frames from the WC
object using the read()
method, and this frame is nothing but an image.
while True:
# this will read images/frames one by one
RET, F = WC.read()
cv2.imshow("Live Feeds", F)
The next instruction displays these images with the help of the imshow()
method, which has two arguments. One is the window name, which will appear in the top right corner, and the second is the image’s content, the numpy
array.
In the next instruction, we receive the feeds from a live camera, and it will keep displaying frames or images in our program. We must break this loop to stop this infinite loop or the camera’s live feeds.
We need to use v2.waitKey(1)
, which will keep the window open until our action if we mention that the key we press on our keyboard should be the exit from the while
loop.
If we press the defined key, it will automatically stop the live feeds we are accessing from a camera.
At the end of the code, we are releasing our WC
object. We call the destroyAllWindows()
method, which will destroy all windows we have opened to display our live feeds.
import numpy as np
import cv2
# Capture video from storage/laptop camera/IP based camera
WC = cv2.VideoCapture(0)
while True:
# this will read images/frames one by one
RET, F = WC.read()
cv2.imshow("Live Feeds", F)
KEY = cv2.waitKey(1) # wait for key press
if KEY == ord("q"):
break
WC.release()
cv2.destroyAllWindows()
Now we can see the camera is capturing real-time video.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn