The Mixin Classes in Python
-
Use the
Mixins
to Solve the Multiple Inheritance Problem in Python -
Use the
Mixins
to Increase Methods for a Class in Python
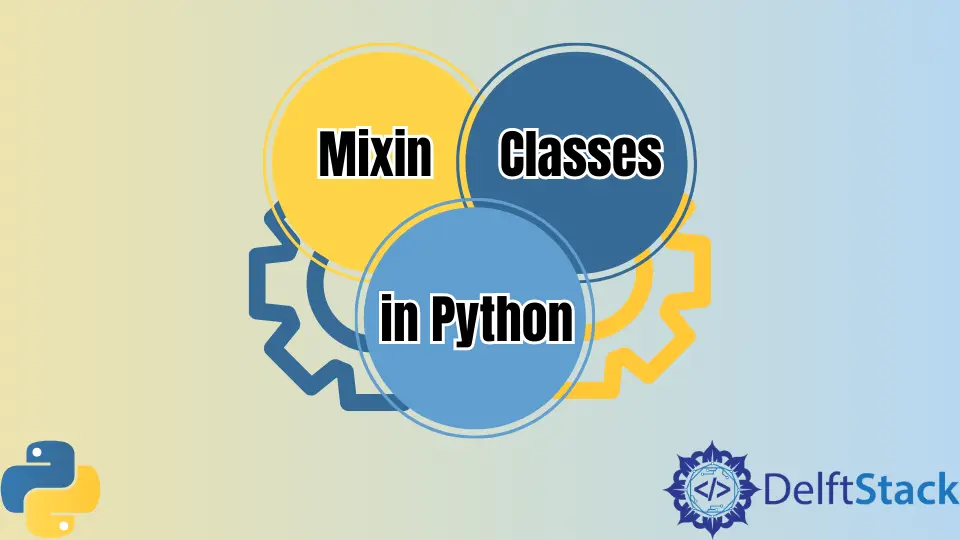
Python is an object-oriented language. Inheritance is an important feature, and it allows objects of one class to inherit variables and methods of another class.
Python supports multiple inheritance, unlike Java and C#. We can derive the features from more than one base class in multiple inheritance.
Multiple inheritance seems like a very useful feature but can cause a lot of ambiguity in the code. A common problem associated with multiple inheritance is the diamond dependency problem.
This problem is understood better with an example. Say we have two classes, X and Y inherit from A, and Z inherits from X and Y.
Some class A
methods are overridden by X and Y, not by Z, so the class does inherit X or Y. The C3 linearization algorithm in Python helps with this to some extent.
Use the Mixins
to Solve the Multiple Inheritance Problem in Python
Mixin is one such feature that helps perform efficient and understandable multiple inheritance avoiding the diamond dependency problem. We will demonstrate the use of mixins in this tutorial.
The mixin
class design pattern avoids the ambiguity associated with multiple inheritance. It is used to create a class that defines a single method.
These are only meant to be inherited and not instantiated. These are used when we use a particular class feature in many other classes.
class base(object):
def fun(self):
print("fun() base")
class mixin_1(object):
def fun(self):
print("fun() mixin 1")
class mixin_2(object):
def fun(self):
print("fun() mixin 2")
class child(mixin_2, mixin_1, base):
pass
ob = child()
ob.fun()
Output:
fun() mixin 2
In Python, the mixin
class does not override the methods from other mixins and the base class. Also, the class hierarchy is from left to right.
That is why the fun()
function from the class mixin_2
is invoked in the above example.
Use the Mixins
to Increase Methods for a Class in Python
Mixins
can also implement a method that uses some function not defined in the class.
This method does not involve any multiple inheritance but aims to increase the functionalities for a class. This class also will serve only as a base class for other classes.
class mixin_class(object):
def __ne__(self, val):
return not (self == val)
def __lt__(self, val):
return self <= val and (self != val)
def __gt__(self, val):
return not self <= val
def __ge__(self, val):
return self == val or self > val
class num_class(mixin_class):
def __init__(self, i):
self.i = i
def __le__(self, val):
return self.i <= val.i
def __eq__(self, val):
return self.i == val.i
print(num_class(10) < num_class(51))
print(num_class(3) != num_class(2))
print(num_class(5) > num_class(2))
print(num_class(5) >= num_class(3))
Output:
True
True
True
True
In the above example, we create the mixin_class
to provide functions for comparing integers even though it does not declare the __le__
and __eq__
functions.
Remember to use the magic functions with __
for comparison invoked while using the comparison operators.
Due to their ability to add new functionalities, the mixin
class works similar to decorators in Python.
However, one should remember that both have different uses as decorators mainly modify the already present methods of a class.
Mixins are also confused with other features like abstract classes and interfaces. Unlike mixins, an abstract class requires one or more abstract methods.
Interfaces contain only abstract methods. The main common ground between the above three features is that they are not instantiated, meaning they cannot exist independently.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn