MIMEMultipart in Python
-
Python’s
MIMEMultipart
,MIMEText
andMIMEBase
Modules - Example Script for Sending Emails With Attachments in Python
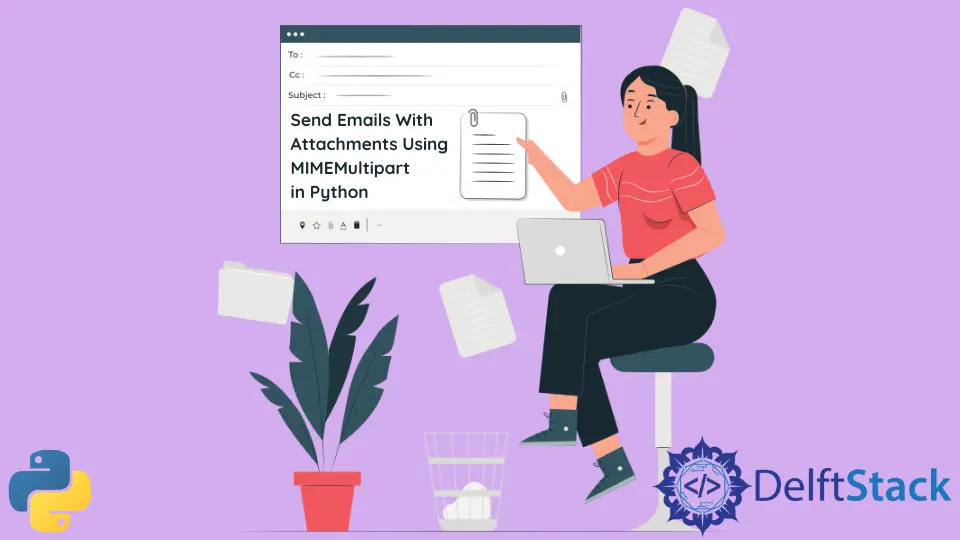
Sending emails as a prompt or reminder or for anything else has become a vital and regular part of our lives. Software developers often encounter situations during the development phase where they want the end-user to receive an email via their application.
In this article, we will learn about efficient ways to send emails with attachments with the help of Python and its MIME (Multipurpose Internet Mail Extensions) module.
Python’s MIMEMultipart
, MIMEText
and MIMEBase
Modules
Much like smtplib
in Python, the MIME modules offer advanced tools to help make the sending-receiving process of emails easier and more enhanced.
While the smtplib
module enables us to send emails without attachments, the MIME
modules are used when we have to send emails with attachments.
The three modules of MIME
have varying and unique purposes, each of which enables it to enhance our experience of sending an email via a Python script.
-
MIMEMultipart
This module is used when we want to construct a message with varying content types. This means that
MIMEMultipart
supports the use of many content types.For example, text/HTML and application/octet-stream. We can also have both HTML and an image within the HTML with the help of multipart.
-
MIMEText
This is used to send text-based emails. This module allows us to write and send a complete text-based email easily.
-
MIMEBase
The
MIMEBase
module is only used as a base class so that we can have more specific subclasses and ‘MIME aware’.
Example Script for Sending Emails With Attachments in Python
While one may think that writing a Python script to send emails with attachments may be pretty complicated as compared to one without, the MIME modules make the whole process rather straightforward.
We need to follow these steps to send an email with an attachment.
Adding an attachment requires us to import the following Python libraries.
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email import encoders
These libraries make the task at hand fairly simple. Luckily, these are native libraries and do the job nicely, so we don’t need any external libraries imported for adding an attachment.
-
First, we begin the script by creating an instance for
MIMEMultipart
, and we call itmsg
. -
Then, we describe the sender’s and receiver’s email addresses. We also describe the subject in the
From
,To
andSubject
keys of the instance we created. -
Now, we need to construct the body of the message we wish to send in a variable called
body
and attach this variable to themsg
instance we created earlier using theattach()
method. -
Now comes the fun part, where we open the file we want to attach. We open this file in the
rb
mode.Following this, we create an instance of
MIMEBase
with two parameters:maintype
andsubtype
. These define the base class for all our MIME-aware sub-classes of the message.Remember that the
maintype
here is the content typemajor
consisting mainly of texts or images, and thesubtype
is the content typeminor
, which typically consists of plains, gifs, audio and/or other media. -
Now, we use
set_payload
to change our payload into an encoded form. We encode it in base 64 and attach this file to the multipart instancemsg
we created earlier.
Here is a Python script to help us visualize and understand the MIME modules’ use.
Example code:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email import encoders
fromaddr = "EMAIL address of the sender"
toaddr = "EMAIL address of the receiver"
# instance of MIMEMultipart
msg = MIMEMultipart()
# storing the sender's email address
msg["From"] = fromaddr
# storing the receiver's email address
msg["To"] = toaddr
# storing the subject
msg["Subject"] = "Subject of the Mail"
# The string to store the body of the mail
body = "Body_of_the_mail"
# attach the body with the msg instance
msg.attach(MIMEText(body, "plain"))
# open the file to be sent
filename = "File_name_with_extension"
attachment = open("Path of the file", "rb")
# The instance of MIMEBase and named as p
p = MIMEBase("application", "octet-stream")
# To change the payload into encoded form
p.set_payload((attachment).read())
# encode into base64
encoders.encode_base64(p)
p.add_header("Content-Disposition", "attachment; filename= %s" % filename)
# attach the instance 'p' to instance 'msg'
msg.attach(p)
# creates SMTP session
s = smtplib.SMTP("smtp.gmail.com", 587)
# start TLS for security
s.starttls()
# Authentication
s.login(fromaddr, "Password_of_the_sender")
# Converts the Multipart msg into a string
text = msg.as_string()
# sending the mail
s.sendmail(fromaddr, toaddr, text)
# terminating the session
s.quit()
This is a reasonably straightforward script to send an email to one person. If we wish to send this email to multiple people, we can use loops to achieve this.
Another important thing to note here is that this script will not work if we have the two-step authentication enabled on our email accounts. For this to work, we must disable the two-step verification.
Sending an email this way also ensures that the email lands directly in the user’s inbox and never in the spam folder.
While this feature can be misused, we know that it can be helpful for businesses requiring urgency and having no room for delays because of a missed email.
We have learned everything there is to know about sending a simple email with an attachment using Python and its native MIME libraries.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn