How to List Serial Ports Using Python
- Brief Introduction to COM Ports
- Get a List of Available Serial Ports in Python
- Get a List of Serial Ports Along With Their Details
- Search a Serial Port by Name
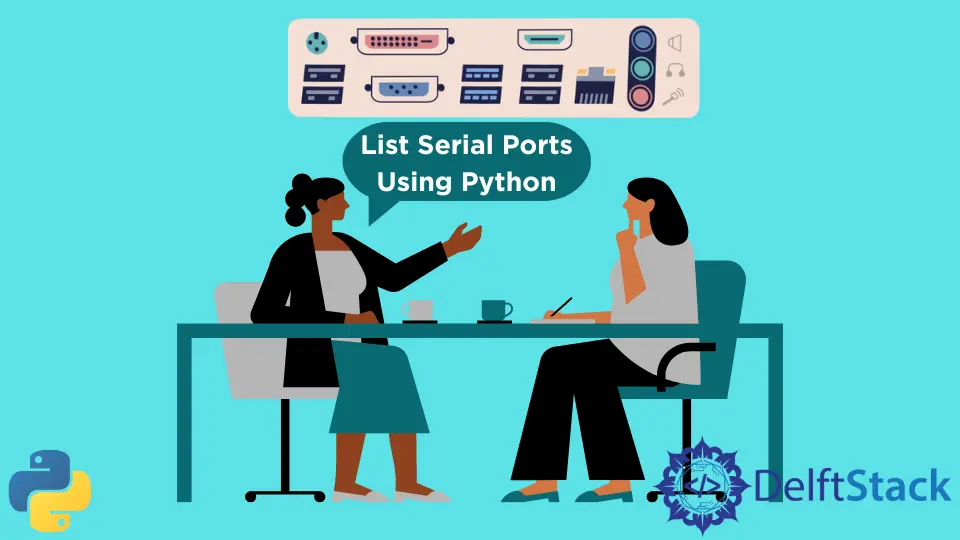
In this article, we’ll discuss communications using serial or com ports. We will explore Python packages in depth to help us get our system’s available communication ports.
Later, we’ll review a few techniques for using Python to search and get detailed information about the available serial ports.
Brief Introduction to COM Ports
A COM port is an I/O interface that makes it possible to connect a serial device to a computer. It is a short form of Communication Port.
COM ports are also sometimes referred to as serial ports. COM ports are no longer commonly used on new computers and devices, but many old serial port devices are still in use.
Therefore, we may sometimes need to do serial communication and list these ports for several serial operations.
Get a List of Available Serial Ports in Python
Sometimes while programming, we need to get information about the available communication ports in our system. We are going to discuss how to do this using Python.
Python offers the pySerial
module, which provides access to the serial ports and the related information. We first need to install the package pySerial
into our project, and then we can easily use its functions.
To install the package, we must type pip install pyserial
in the Python command terminal and press Enter. The package will be available to use.
Now, let’s see the following code listing the serial ports:
import serial.tools.list_ports
ports = []
for port in serial.tools.list_ports.comports():
ports.append(port.name)
print(ports)
Output:
['COM1']
Let’s discuss what we did in the code. We have to write import serial
to use the installed package.
The package pySerial
has a comports()
method which returns the list of available COM ports. Each object in this list is of type ListPortInfo
.
We will discuss later in the article what this ListPortInfo
object holds.
The comports()
function is in the module list_ports,
which is in tools
. So we import the whole module by writing import serial.tools.list_ports
.
Then we just run a for
loop on the list returned by the comports()
function and append the port to our list.
The above code can be written more simply by using Python list comprehension. The output will be the same for both codes. Here’s how.
import serial.tools.list_ports
print([port.device for port in serial.tools.list_ports.comports()])
Get a List of Serial Ports Along With Their Details
As we discussed, the comports()
function returns the list of ports, and each object in the list is of the type ListPortInfo
. This object holds information about the serial ports and provides indexed access to retrieve the device (full name/path), description, and hwid
of the serial port.
The index 0 will give us the device’s value, the description is at index 1, and index 2 will share the hwid
of the port.
Following is the detailed information the ListPortInfo
object can give us about the COM port:
Object | Description |
---|---|
device |
Full device name/path. This will be returned as the first element when accessed by the index. |
name |
Short device name. |
description |
Human-readable description. This will be returned as the second element when accessed by the index. |
hwid |
Hardware ID. This will be returned as the third element when accessed by the index. |
vid |
USB Vendor ID. |
pid |
USB Product ID. |
serial_number |
USB Serial number as a string. |
location |
USB device location string. |
manufacturer |
USB manufacturer string, as reported by the device. |
product |
USB product string, as reported by the device. |
interface |
Interface-specific description. |
Note: Support is limited to a few operating systems. The
description
andhwid
might not be available on all systems.
Now, let’s write a code to get a list of all available com ports along with their name
, description
, manufacturer
, and hwid
.
import serial.tools.list_ports
port_data = []
for port in serial.tools.list_ports.comports():
info = dict(
{
"Name": port.name,
"Description": port.description,
"Manufacturer": port.manufacturer,
"Hwid": port.hwid,
}
)
port_data.append(info)
print(port_data)
Output:
[{'Name': 'COM1', 'Description': 'Communications Port (COM1)', 'Manufacturer': '(Standard port types)',
'Hwid': 'ROOT\\PORTS\\0000'}]
Search a Serial Port by Name
This function is useful when we have multiple ports in our computer. If we need any specific port, we can search for it by its name or description per our choice.
Let’s create a function in Python that will return a port of our given name.
import serial.tools.list_ports
def get_port_by_name(port_name):
for port in serial.tools.list_ports.comports():
if port.name == port_name:
return port
print(get_port_by_name("COM1").description)
Output:
Communications Port (COM1)
The code is simple to grasp. In our function, a for
loop runs on the list of ports returned by the comports()
function.
We verify each port’s name inside the loop to see whether it matches the user input. If it does, we return that port.
You may construct a similar function for description
, which will return the port by checking the description.