How to Create an IRC Bot in Python 3
- Create an IRC bot in Python
- Create Python Script Containing the Class
- Initiate Messages and Connect to a Server in Python
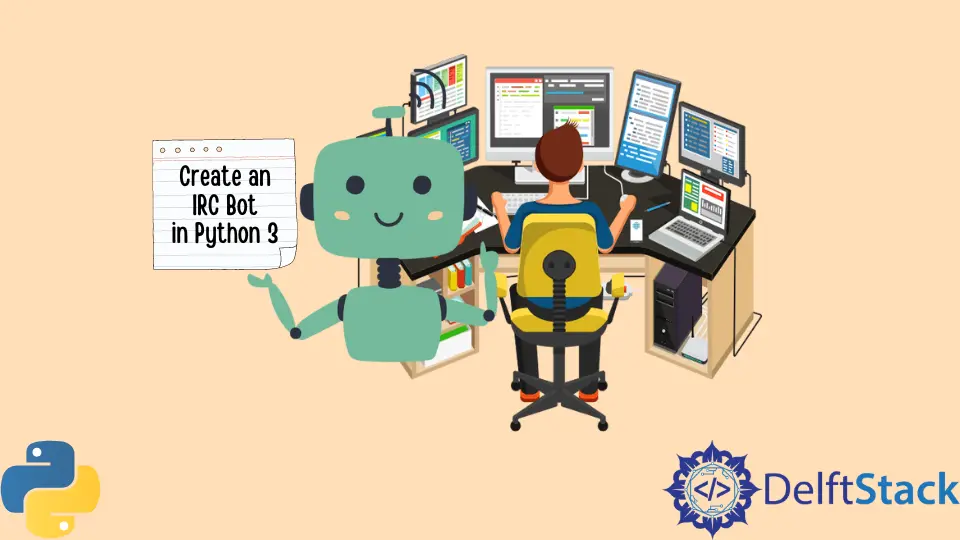
IRC bots are program scripts that provide instant user responses using the IRC protocol. These bots can act as virtual assistants to respond to active users quickly.
This tutorial will demonstrate how to create a simple IRC bot in Python.
Create an IRC bot in Python
To create an IRC bot, we need to connect the program to a server. For this, we will use sockets.
Python’s socket module implements the socket-API to connect two nodes on the same network to facilitate communication.
After connecting to the network, we must pass some commands and values for authentication with the IRC server. We need to specify the password using the PASS
command.
We specify the username with the NICK
command and the USER
command to provide the username, hostname, server, and real name. Then the server responds with a PING
, and we need to provide a PONG
command.
Create Python Script Containing the Class
To create a simple bot, we will first need to create a Python script that contains the class, which will have the methods to generate connections and send messages.
See the code below.
import socket
import sys
class bot_irc:
irc_socket = socket.socket()
def __init__(self):
self.irc_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
def send_irc(self, channel, msg):
self.irc_socket.send(bytes("PRIVMSG " + channel + " " + msg + "\n", "UTF-8"))
def connect_irc(self, server, port, channel, bot_nick, bot_pass, bot_nickpass):
print("Server connection: " + server)
self.irc_socket.connect((server, port))
self.irc_socket.send(
bytes(
"USER " + bot_nick + " " + bot_nick + " " + bot_nick + " :python\n",
"UTF-8",
)
)
self.irc_socket.send(bytes("NICK " + bot_nick + "\n", "UTF-8"))
self.irc_socket.send(
bytes("NICKSERV IDENTIFY " + bot_nickpass + " " + bot_pass + "\n", "UTF-8")
)
self.irc_socket.send(bytes("JOIN " + channel + "\n", "UTF-8"))
def response_irc(self):
r = self.irc_socket.recv(2040).decode("UTF-8")
if r.find("PING") != -1:
self.irc_socket.send(
bytes("PONG " + r.split().decode("UTF-8")[1] + "\r\n", "UTF-8")
)
return r
In the example, we created a class to represent the structure for an IRC bot in Python.
We use the socket.socket()
constructor to create a socket
object. This object is used in every method of the class.
The class’s connect_irc()
method connects to the required server. We provide the value for the previously discussed commands within this function.
Since we are working with Python 3, we need to send bytes which are the values.
We achieve this by using the socket.send()
function and encoding the data as UTF-8 with the bytes()
function.
We have the send_irc()
function, which sends bytes. The response_irc()
function works on the received response.
In this function, the response is decoded as a string and returned. We then check it for the server’s returned PING
value.
We search for it using the find()
function. If there is a PING
value, we send a PONG
command to the server as bytes using the socket.send()
function.
Initiate Messages and Connect to a Server in Python
We can use instances of this class on the client-side to initiate messages and connect to the server.
Example:
server_irc = "10.x.x.10"
port_irc = 6697
channel_irc = "#python"
botnick_irc = "delftstack"
botnickpass_irc = "nickkpass"
botpass_irc = "botpass//"
irc = bot_irc()
irc.connect_irc(
server_irc, port_irc, channel_irc, botnick_irc, botpass_irc, botnickpass_irc
)
while True:
text = irc.response_irc()
print(text)
if "PRIVMSG" in text and channel in text and "hello" in text:
irc.send_irc(channel, "First message")
In the example, we created an instance of the bot_irc
class defined earlier and tried to connect to an IRC server with the necessary values.
After initiating the connection, we check the response to see the success and send our first message if the connection was successful.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn