How to Install SQLite in Python
-
Use
pip
to Install Sqlite3 in Python - Use Conda to Install Sqlite3
- Use the Standard Library to Use Sqlite3 in Python
-
Use a Third-Party Library (
sqlite-utils
) to Use Sqlite3 in Python - Use an ORM (Object-Relational Mapping) - SQLAlchemy in Python
- Use an External SQLite Client in Python
- Conclusion
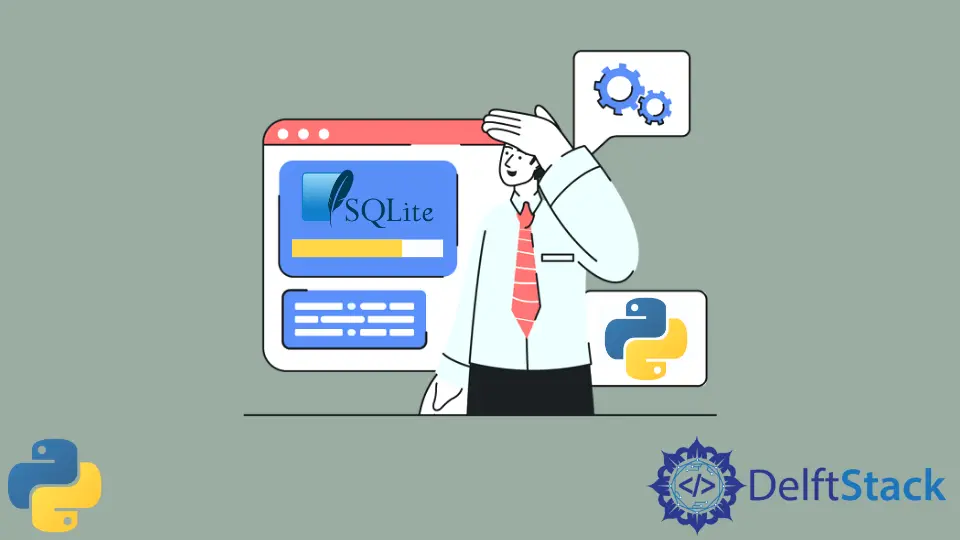
SQLite is a lightweight, serverless, self-contained database engine that is easy to set up and use with Python. The only downside is that it provides only one writer at a time.
We can access SQLite databases with Python. Python provides support to work with such databases and manipulate them. The support for this is included by default in the standard Python library.
The sqlite3
package provides different functionalities to work with SQLite databases. However, if one faces an issue with this, we can very easily install it using the pip
command.
The pip
allows us to download and manage different packages in Python.
In this guide, we will explore different methods to install and use SQLite in Python, providing example code and explanations for each approach.
Use pip
to Install Sqlite3 in Python
pip
is a package manager for Python. It’s a command-line tool that allows you to install, manage, and uninstall Python packages (libraries or modules) from the Python Package Index (PyPI) and other package indexes.
We will walk you through the steps to install SQLite3 in Python using pip
.
-
Open a Terminal or Command Prompt
First, open a terminal or command prompt on your system. This is where you’ll enter the commands to install SQLite3.
-
Check if Python is Installed
Before proceeding, make sure you have Python installed on your system. You can check by running the following command:
python --version
This should display the installed Python version. If Python is not installed, download and install it from the official website.
-
Install SQLite3 using
pip
SQLite3 is part of the Python standard library, so you don’t need to install it separately. However, it’s always a good practice to ensure you have the latest version, and you can do this by running:
pip install --upgrade sqlite3
If you’re using an older version of Python (2.x), you may need to use
pip3
instead ofpip
:pip3 install --upgrade sqlite3
-
Verify the Installation
To confirm that SQLite3 is installed, you can open a Python shell by typing
python
orpython3
in the terminal. Then, enter the following Python code:import sqlite3 print(sqlite3.sqlite_version)
This will print the version of SQLite3 that is installed on your system.
Additional Information
-
What if SQLite3 is Already Installed?
If you’re using a recent version of Python (3.4 and above), SQLite3 is already included in the standard library. However, it’s still recommended to ensure you have the latest version by running the upgrade command.
-
Using SQLite3 in Your Python Projects:
Once installed, you can start using SQLite3 in your Python projects. The
sqlite3
module provides a simple and efficient way to interact with SQLite databases.You can create connections, execute queries, and manage transactions using the functions provided by this module.
-
Installing Specific Versions:
If you need to install a specific version of SQLite3, you can specify the version number in the installation command. For example:
pip install sqlite3==3.36.0
-
Installing on Virtual Environments:
If you’re working in a virtual environment, you can install SQLite3 in the same way as described above. Just make sure your virtual environment is activated before running the
pip
command.
Use Conda to Install Sqlite3
Conda is an open-source package management and environment management system that runs on Windows, macOS, and Linux. It allows you to easily install, run, and update packages and their dependencies.
Below are the steps of how to install SQLite3 using Conda.
-
Install Conda
If you haven’t installed Conda yet, you can download it from the official Anaconda website, or you can install Miniconda, which is a smaller, lighter version of Anaconda from here.
Follow the installation instructions for your operating system. Once Conda is installed, you’ll have access to the
conda
command in your terminal or command prompt. -
Create a New Environment
It’s always good practice to work in a dedicated environment for your project. This helps manage dependencies and ensures that your project doesn’t interfere with other packages on your system.
To create a new environment, open your terminal or command prompt and run:
conda create --name my_env python=3.x
Replace
my_env
with the desired name of your environment and3.x
with your preferred Python version (e.g.,3.7
,3.8
, etc.). -
Activate the Environment
After creating the environment, you need to activate it. This will ensure that any packages you install will be specific to this environment.
conda activate my_env
Replace
my_env
with the name of your environment. -
Install SQLite3
To install SQLite3, you can use Conda’s package manager. In your activated environment, run:
conda install -c conda-forge sqlite
This command instructs Conda to install the
sqlite
package from theconda-forge
channel. Theconda-forge
channel is a community-maintained repository of Conda packages. -
Verify the Installation
You can now verify that SQLite3 has been successfully installed in your environment. Open a Python shell in your terminal or command prompt and try importing the SQLite3 module:
import sqlite3
If there are no error messages, SQLite3 is installed and ready to use.
Use the Standard Library to Use Sqlite3 in Python
Python’s standard library includes the sqlite3
module, which provides an interface for interacting with SQLite databases. This method does not require any additional installations as it comes pre-packaged with Python.
Example Code:
import sqlite3
# Create a connection to a SQLite database (this will create a new one if it doesn't exist)
conn = sqlite3.connect("example.db")
# Create a cursor object to execute SQL queries
cursor = conn.cursor()
# Execute a simple SQL command to create a table
cursor.execute(
"""CREATE TABLE IF NOT EXISTS users
(id INTEGER PRIMARY KEY, name TEXT, age INTEGER)"""
)
# Commit the changes and close the connection
conn.commit()
conn.close()
The import sqlite3
line imports the sqlite3
module, allowing us to use its functions to interact with SQLite databases.
Then, sqlite3.connect('example.db')
creates a connection to the SQLite database. If 'example.db'
does not exist, it will be created, but if it does exist, a connection to the existing database will be established.
conn.cursor()
creates a cursor object. A cursor is required to execute SQL commands on the database.
Next, the cursor.execute(...)
method is used to execute SQL commands. In this example, we create a table named users
with columns id
, name
, and age
.
The conn.commit()
commits the changes to the database. Without this, the changes will not be saved.
Finally, the conn.close()
closes the connection to the database. It’s important to always close the connection when you’re done to avoid potential issues.
Use a Third-Party Library (sqlite-utils
) to Use Sqlite3 in Python
sqlite-utils is a Python library that provides additional functionalities for SQLite. It can be installed using pip
.
Installation:
pip install sqlite-utils
Example Code:
import sqlite_utils
# Create a SQLite database
db = sqlite_utils.Database("example.db")
# Create a table with columns
db["users"].insert({"name": "John Doe", "age": 30})
Explanation:
The import sqlite_utils
line imports the sqlite_utils
module.
Then, the sqlite_utils.Database("example.db")
creates an SQLite database. If 'example.db'
does not exist, it will be created, and if it does exist, a connection to the existing database will be established.
Lastly, the db["users"].insert(...)
line inserts a new record into the users
table. In this example, we insert a user with the name "John Doe"
and age 30
.
Use an ORM (Object-Relational Mapping) - SQLAlchemy in Python
SQLAlchemy is a popular Python library that provides a high-level interface for interacting with databases. It supports multiple database systems, including SQLite.
Installation:
pip install sqlalchemy
Example Code:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Define the model
Base = declarative_base()
class User(Base):
__tablename__ = "users"
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
# Create an SQLite database
engine = create_engine("sqlite:///example.db")
# Create tables
Base.metadata.create_all(engine)
# Create a session
Session = sessionmaker(bind=engine)
session = Session()
# Add a user
new_user = User(name="Jane Doe", age=25)
session.add(new_user)
session.commit()
Explanation:
First, the from sqlalchemy import ...
lines import necessary components from SQLAlchemy. We create a base class for declarative models using the Base = declarative_base()
.
Then, the class User(Base)
defines a model class called User
while the engine = create_engine('sqlite:///example.db')
creates an SQLite database engine. The Base.metadata.create_all(engine)
creates the tables defined in our models.
Next, we set up a session factory using the Session = sessionmaker(bind=engine)
. With the session = Session()
, we create a session, which is used to interact with the database.
The new_user = User(name='Jane Doe', age=25)
then creates a new User
object. We add the new user to the session using session.add(new_user)
.
Finally, we commit the changes to the database by utilizing the session.commit()
.
Use an External SQLite Client in Python
You can also install an external SQLite client and interact with it using Python. One popular client is sqlite3
, available via the command line.
Installation:
# Install sqlite3 via your system's package manager
# For example, on Ubuntu:
sudo apt-get install sqlite3
Example Code:
import subprocess
def execute_sql(query):
subprocess.run(f'sqlite3 example.db "{query}"', shell=True)
execute_sql("CREATE TABLE tasks (id INTEGER PRIMARY KEY, task TEXT)")
execute_sql('INSERT INTO tasks (task) VALUES ("Task 1")')
Explanation:
The import subprocess
module allows you to spawn new processes, which we’ll use to interact with the sqlite3
command-line client. We then use the def execute_sql(query): ...
function to take an SQL query as input and use the subprocess.run()
to execute it using the sqlite3
command-line client.
Then, the execute_sql('CREATE TABLE tasks (id INTEGER PRIMARY KEY, task TEXT)')
creates a new table named tasks
with two columns: id
and task
. And finally, we utilize the execute_sql('INSERT INTO tasks (task) VALUES ("Task 1")')
to insert a new task into the tasks
table.
Conclusion
This comprehensive guide covers various methods of working with SQLite in Python. It begins by introducing SQLite3, a lightweight and easily set up database engine.
The guide then explores different ways to interact with SQLite databases using Python, including the standard sqlite3
module, third-party libraries like sqlite-utils
, using an ORM like SQLAlchemy, and executing SQL queries through an external SQLite client.
Each method is explained in detail with code examples, providing a range of options for integrating SQLite into Python projects based on specific requirements and preferences.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn