How to Access Environment Variables in Python
- What Are Environment Variables
-
Environment Variables in Python -
os.environ
- Read Environment Variables in Python
- Set Environment Variables in Python
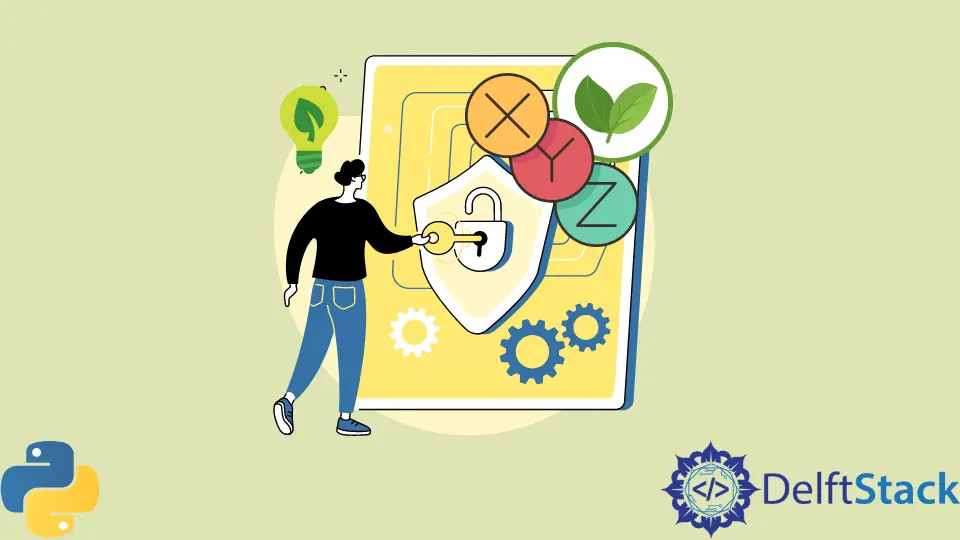
What Are Environment Variables
Environment variables are variables that are assigned values externally to the Python program. Developers usually set them on the command-line before invoking the Python executable. The operating system then makes these variables accessible to a Python program from within.
Environment variables exist for program flexibility. In this way, a user may change specific parameters before program execution, and the program will be able to check these parameters and modify its behavior dynamically.
No code modification is required, and such use of environment variables is called program configuration.
Environment Variables in Python - os.environ
The mechanism of setting environment variables is platform-dependent. For that reason, they are made available through Python’s built-in os
module, which abstracts away operating system dependent functionality.
Python runtime keeps all environment variables of the program in a dictionary-like os.environ
object.
Note that the os.environ
object is populated when the Python runtime loads the os
module. If you try to change environment variables after the fact (for example, exporting a new environment variable in the terminal emulator), it will not work.
Read Environment Variables in Python
Accessing environment variables in Python is done by performing dictionary-like operations on the os.environ
object.
>>> import os
>>> os.environ
environ({'HOME': '/Users/john', 'LANG': 'en_US.UTF-8', 'LOGNAME': 'john', 'OLDPWD': '/Users/john', 'PATH': '/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin', 'PWD': '/Users/john/python-tutorial', 'SHELL': '/bin/zsh', 'TERM': 'screen', 'TERM_PROGRAM': 'Apple_Terminal', 'TERM_PROGRAM_VERSION': '433', 'TERM_SESSION_ID': 'CDC91EF3-15D6-41AD-A17B-E959D97BC4B5', 'TMPDIR': '/var/folders/md/31nwv67j113d19z0_1287f1r0000gn/T/', 'TMUX': '/private/tmp/tmux-501/default,3319,0', 'TMUX_PANE': '%28' , 'USER': 'john', '_': '/usr/local/bin/python3', '__PYVENV_LAUNCHER__': '/usr/local/bin/python3'})
>>> os.environ['HOME']
'/Users/john'
>>> os.environ['LANG']
'en_US.UTF-8'
If you get an environment variable in Python using a subscript []
notation, and that variable is undefined, you will get a runtime error.
>>> os.environ['I_DONT_EXIST']
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/usr/local/Cellar/python/3.7.5/Frameworks/Python.framework/Versions/3.7/lib/python3.7/os.py", line 679, in __getitem__
raise KeyError(key) from None
KeyError: 'I_DONT_EXIST'
To avoid this problem, you can read the environment variable from the os.environ
object using the get()
interface. If a looked up environment variable has not been defined, get()
returns None
.
>>> result = os.environ.get('I_DONT_EXIST')
>>> print(result)
None
A convenient feature of the get()
interface is that you can specify a default value to use if a searched for environment variable doesn’t exist.
>>> os.environ.get('I_DONT_EXIST', 'I_AM_DEFAULT')
'I AM DEFAULT'
An alternative to os.environ.get()
is to use Python os.getenv()
method. Both functions work the same, and you may treat the latter as a convenience API.
Set Environment Variables in Python
Sometimes you need to change or append an environment variable from within your program. For example, it may happen if you need to modify your application’s behavior upon receiving a configuration network request. You change environment variables using the subscript []
operator as if os.environ
has been a standard dictionary.
>>> import os
>>> os.environ['LANG']
'en_US.UTF-8'
>>> os.environ['LANG'] = 'en_GB.UTF-8'
>>> os.environ['LANG']
'en_GB.UTF-8'
Note that environment variable values should be a string type. If you try to assign an integer or any other non-string value to an environment variable, you will get a runtime error.
>>> import os
>>> os.environ['LANG'] = 4
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/usr/local/Cellar/python/3.7.5/Frameworks/Python.framework/Versions/3.7/lib/python3.7/os.py", line 684, in __setitem__
value = self.encodevalue(value)
File "/usr/local/Cellar/python/3.7.5/Frameworks/Python.framework/Versions/3.7/lib/python3.7/os.py", line 754, in encode
raise TypeError("str expected, not %s" % type(value).__name__)
TypeError: str expected, not int