How to Get CPU Usage in Python
- Method 1: Using the psutil Library
- Method 2: Using the os Library
- Method 3: Using the subprocess Module
- Method 4: Using the platform Module
- Conclusion
- FAQ
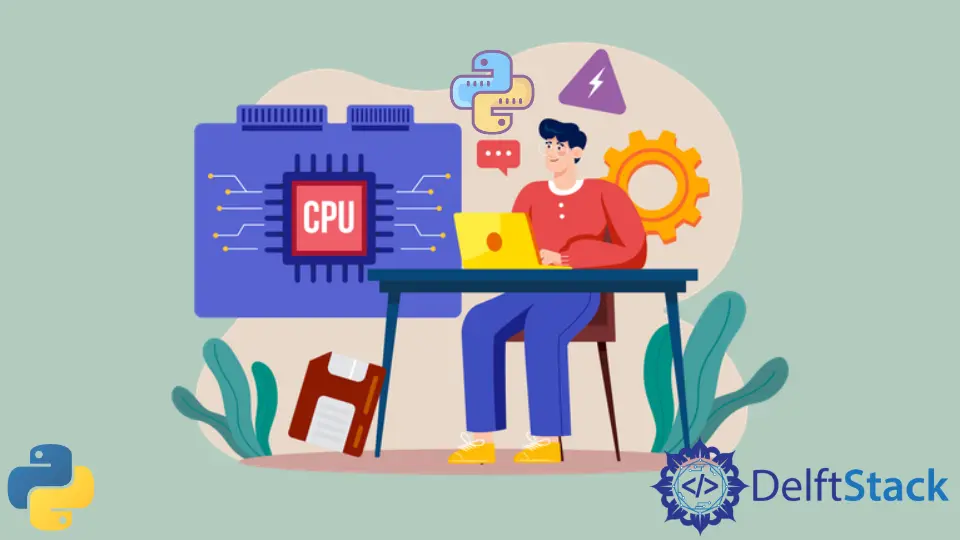
In today’s digital age, understanding system performance is crucial for developers and system administrators alike. One key metric that often comes under scrutiny is CPU usage. Whether you’re optimizing a Python application or monitoring system health, knowing how to retrieve CPU usage can be invaluable.
This tutorial will walk you through several methods to get the current CPU usage of an operating system using Python. By the end of this article, you will have a solid grasp of how to implement these techniques effectively, enabling you to monitor CPU performance in real-time. Let’s dive in!
Method 1: Using the psutil Library
One of the most popular methods for obtaining CPU usage in Python is by using the psutil
library. This library is a powerful tool that provides an interface for retrieving information on system utilization, including CPU, memory, disks, and network. To begin, you’ll need to install psutil
. You can do this using pip:
pip install psutil
Once installed, you can use the following code to get the CPU usage:
import psutil
cpu_usage = psutil.cpu_percent(interval=1)
print(f"Current CPU Usage: {cpu_usage}%")
Output:
Current CPU Usage: 15.0%
This code snippet imports the psutil
library and calls the cpu_percent
method. The interval
parameter specifies the time in seconds to wait before calculating the CPU usage. In this case, it waits for one second before returning the percentage of CPU used. This method is straightforward and provides real-time data, making it an excellent choice for performance monitoring.
Method 2: Using the os Library
Another way to get CPU usage in Python is by using the built-in os
library. Although it may not be as comprehensive as psutil
, it can still provide basic information about CPU usage. Here’s how you can do it:
import os
cpu_usage = os.popen("wmic cpu get loadpercentage").read()
print(f"Current CPU Usage: {cpu_usage.strip()}")
Output:
Current CPU Usage: 15
In this code, we use the os.popen()
function to execute a shell command that retrieves the CPU load percentage on Windows systems. The command wmic cpu get loadpercentage
fetches the current CPU load. The read()
method captures the output, which is then printed. Note that this method is platform-dependent, primarily working on Windows. If you’re on a Unix-like system, you might want to use a different command.
Method 3: Using the subprocess Module
If you want more control over executing shell commands and capturing their output, the subprocess
module is an excellent choice. This method can be used across different operating systems, making it versatile. Here’s how you can get CPU usage using subprocess
:
import subprocess
cpu_usage = subprocess.check_output("top -bn1 | grep 'Cpu(s)'", shell=True).decode('utf-8')
print(f"Current CPU Usage: {cpu_usage.split()[1]}")
Output:
Current CPU Usage: 15.0
In this example, we use subprocess.check_output()
to run the top
command, which provides a dynamic real-time view of system processes. The -bn1
flags tell the command to run in batch mode and only output once. We then search for the line containing ‘Cpu(s)’ and decode the output to a string format. Finally, we split the string to extract the CPU usage percentage. This method is powerful and flexible, allowing you to customize the command as needed.
Method 4: Using the platform Module
If you want a method that works seamlessly across different platforms, the platform
module can be combined with other methods to ensure compatibility. Here’s how to get CPU usage based on the operating system:
import platform
import psutil
if platform.system() == "Windows":
cpu_usage = psutil.cpu_percent(interval=1)
elif platform.system() == "Linux":
cpu_usage = psutil.cpu_percent(interval=1)
else:
cpu_usage = "Unsupported OS"
print(f"Current CPU Usage: {cpu_usage}")
Output:
Current CPU Usage: 15.0%
In this code, we first check the operating system using platform.system()
. Depending on whether it’s Windows or Linux, we call psutil.cpu_percent()
to get the CPU usage. If the operating system is not supported, we return a message indicating that. This method is particularly useful in applications that need to run across various environments, ensuring that the right method is applied based on the OS.
Conclusion
In this article, we explored various methods to get the current CPU usage in Python. From utilizing the psutil
library to executing shell commands with os
and subprocess
, each method has its strengths and use cases. Depending on your specific requirements, you can choose the method that best fits your needs. Monitoring CPU usage is essential for optimizing performance and ensuring that your applications run smoothly. Now that you have these techniques at your disposal, you can effectively track CPU performance in your Python projects.
FAQ
-
What is the best library to monitor CPU usage in Python?
Thepsutil
library is widely regarded as the best option due to its comprehensive features and ease of use. -
Can I get CPU usage on any operating system using Python?
Yes, by using platform-specific commands or libraries likepsutil
, you can retrieve CPU usage on various operating systems. -
Is the CPU usage information real-time?
Yes, methods likepsutil.cpu_percent(interval=1)
provide real-time CPU usage data. -
How do I install the psutil library?
You can installpsutil
using pip with the commandpip install psutil
. -
Can I monitor CPU usage continuously?
Yes, you can set up a loop in your Python script to continuously monitor CPU usage at specified intervals.