How to Generate GUID/UUID in Python
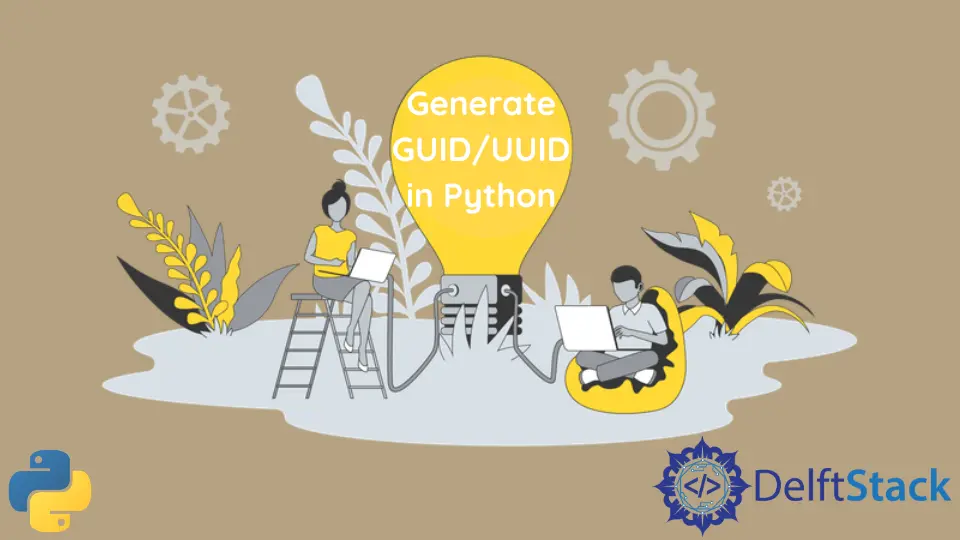
Generating a Globally Unique Identifier (GUID) or Universally Unique Identifier (UUID) in Python is a straightforward task that can be achieved using the built-in uuid
module. These identifiers are essential in various applications, especially when you need to ensure that your identifiers are unique across different systems. Whether you’re working on a database, a web application, or any project that requires unique keys, understanding how to generate UUIDs in Python is invaluable.
In this article, we will explore different methods to create UUIDs, complete with code examples and detailed explanations to help you grasp the concept thoroughly.
What is a UUID?
Before we dive into the code, let’s clarify what a UUID is. A UUID is a 128-bit number used to uniquely identify information in computer systems. The uniqueness of a UUID is guaranteed by the combination of time, hardware, and random numbers, making it an excellent choice for distributed systems where uniqueness is crucial. In Python, the uuid
module provides various functions to create different versions of UUIDs, such as UUID1, UUID3, UUID4, and UUID5. Each version has its own method of generating the unique identifier.
Generating UUIDs with Python’s uuid
Module
The simplest way to generate a UUID in Python is by using the built-in uuid
module. This module offers several methods to create different types of UUIDs. Here, we will focus on the most commonly used methods: UUID1, UUID3, UUID4, and UUID5.
Method 1: Generating UUID1
UUID1 generates a UUID based on the current timestamp and the MAC address of the host machine. This means it is time-based and can be used to track the creation time of the UUID.
import uuid
uuid1 = uuid.uuid1()
print(uuid1)
Output:
a1b2c3d4-e5f6-7a8b-9c0d-e1f2g3h4i5j6
The code above imports the uuid
module and uses the uuid1()
function to generate a UUID. The resulting UUID is printed to the console. Since UUID1 incorporates the MAC address and the current timestamp, it guarantees uniqueness across different machines and times. However, keep in mind that it may expose the MAC address, which can be a privacy concern in some applications.
Method 2: Generating UUID3
UUID3 generates a UUID based on the MD5 hash of a namespace identifier and a name. This method is deterministic, meaning that the same inputs will always produce the same UUID.
import uuid
namespace = uuid.NAMESPACE_DNS
name = 'example.com'
uuid3 = uuid.uuid3(namespace, name)
print(uuid3)
Output:
6ba7b810-9dad-11d1-80b4-00c04fd430c8
In this example, we first define a namespace using uuid.NAMESPACE_DNS
and provide a name, which is ’example.com’. The uuid3()
function generates a UUID based on the MD5 hash of the namespace and name. This method is useful for generating consistent UUIDs for the same input, making it ideal for applications where you need to ensure the same identifier is generated for a given input.
Method 3: Generating UUID4
UUID4 generates a random UUID. This is the most commonly used method for creating UUIDs when you need a unique identifier without any specific input.
import uuid
uuid4 = uuid.uuid4()
print(uuid4)
Output:
123e4567-e89b-12d3-a456-426614174000
The uuid4()
function creates a random UUID. It does not rely on any external input, making it suitable for scenarios where you need a unique identifier that does not need to be derived from other data. This method is widely used in applications like databases, web services, and APIs, where a unique key is essential.
Method 4: Generating UUID5
UUID5 generates a UUID based on the SHA-1 hash of a namespace identifier and a name, similar to UUID3 but using SHA-1 instead of MD5.
import uuid
namespace = uuid.NAMESPACE_URL
name = 'https://example.com'
uuid5 = uuid.uuid5(namespace, name)
print(uuid5)
Output:
a7f5a5d4-8c3a-5e28-9c4f-3c7f5e8a5f3e
In this example, we use uuid.NAMESPACE_URL
as the namespace and provide a URL as the name. The uuid5()
function creates a UUID based on the SHA-1 hash of the inputs. Like UUID3, this method is deterministic, ensuring that the same inputs will always yield the same UUID. This is particularly useful for generating identifiers for resources that need to remain consistent over time.
Conclusion
Generating GUIDs or UUIDs in Python is a simple yet powerful technique that can enhance the integrity of your applications. By utilizing the built-in uuid
module, you can create unique identifiers tailored to your specific needs, whether they are time-based, deterministic, or random. Understanding the differences between UUID1, UUID3, UUID4, and UUID5 allows you to choose the most appropriate method for your project. With this knowledge, you can confidently generate UUIDs that ensure uniqueness and reliability in your applications.
FAQ
-
What is the difference between GUID and UUID?
GUID (Globally Unique Identifier) and UUID (Universally Unique Identifier) are often used interchangeably, but UUID is the standardized term defined by the ISO/IEC 9834-8:2005 specification. -
Can UUIDs be duplicated?
Theoretically, UUIDs can be duplicated, but the probability is extremely low, especially when using UUID4, which generates random UUIDs. -
How do I install the
uuid
module in Python?
Theuuid
module is a built-in module in Python, so you don’t need to install it separately. Just import it in your script. -
Which UUID version is the most secure?
UUID4 is considered the most secure for general use because it is randomly generated and does not expose any identifiable information. -
Can UUIDs be used as primary keys in databases?
Yes, UUIDs can be used as primary keys in databases, providing a way to uniquely identify records across distributed systems.