Gaussian Kernel Python
- Overview of Gaussian Kernel
- NumPy Library in Python
- Use NumPy to Calculate the Gaussian Kernel Matrix
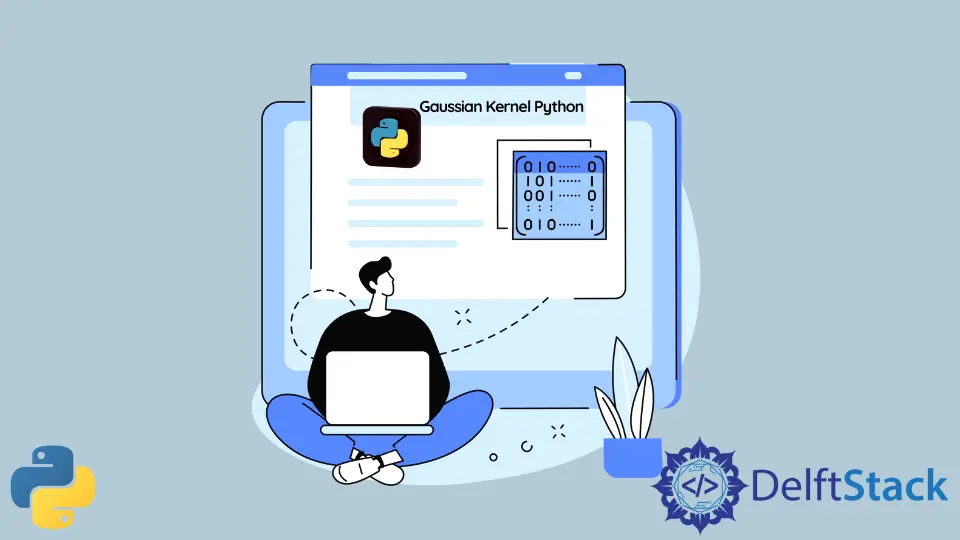
This tutorial describes the gaussian kernel and demonstrates the use of the NumPy library to calculate the gaussian kernel matrix in Python.
Overview of Gaussian Kernel
The Gaussian kernel is a popular function used in various machine learning algorithms. It is also known as the Radial Basis Function (RBF) kernel.
The Gaussian kernel is a function that takes two inputs (x
and y
) and returns a value that indicates the similarity between the two inputs.
The Gaussian kernel is often used in Support Vector Machines (SVMs) and other supervised learning algorithms. The kernel function is defined as:
K(x, y) = e^-{(\frac{||x-y||^{2}}{2\sigma^2})}
where ||x-y||
is the Euclidean distance between x
and y
, and sigma
is a parameter that controls the width
of the Gaussian function.
The Gaussian kernel is a positive definite function, which always returns a positive number. This property is helpful in many machine learning algorithms, as it allows the algorithm to penalize points far from the decision boundary.
NumPy Library in Python
The NumPy library is the best tool for performing numerical operations in Python. In addition, it provides a wide range of features that make it an ideal choice for scientific and technical computing.
Some of the main features of NumPy include:
- A powerful N-dimensional array object.
- Sophisticated (broadcasting) functions.
- Tools for integrating C/C++ and Fortran code.
- Linear algebra, Fourier transform, and random number capabilities.
The NumPy is free and open-source, and its community is active and supportive. As a result, it is an excellent choice for anyone looking for a powerful numerical computing library for Python.
We can use the Numpy library for scientific computing in Python. It provides a high-performance multidimensional array object and tools for working with these arrays.
We can also use it for numerical analysis and machine learning in Python. And it is also the basis for the famous Panda’s library and the foundation of the popular SciPy library.
Use NumPy to Calculate the Gaussian Kernel Matrix
In Numpy, the Gaussian function is implemented via the Gaussian Kernel function. This function takes two arrays as input. The first array is a standard regular array, and the second array is a matrix of weights.
The output from Gaussian Kernel is a matrix of outcomes. A Gaussian kernel matrix is a 3 x 3
matrix and an array of 9
numbers.
For example numbers will be like w_0
, w_1
, w_2
, w_3
, epsilon
, sigma
, gamma
, and kappa
.
The Gaussian kernel matrix is a matrix that is used to approximate the results of a Gaussian process. It is composed of the product of two matrices:
- The data matrix.
- The inverse of the covariance matrix.
The data matrix is a matrix of the data points used in the Gaussian process. The inverse of the covariance matrix is a matrix used to estimate the uncertainties in the data.
To calculate the Gaussian kernel matrix, you first need to calculate the data matrix’s product and the covariance matrix’s inverse. It can be done using the NumPy library.
We can use the NumPy function pdist
to calculate the Gaussian kernel matrix. This function takes an array of data points and calculates the pairwise distances between them.
We can then use the exponential function to calculate the Gaussian kernel for each pair of data points.
The Gaussian kernel matrix is a square matrix with dimensions n x n
, where n
is the number of data points. The elements of the matrix are the pairwise distances between the data points.
Example Code:
import numpy as np
import scipy.stats as st
def gkern(kernlen=21, nsig=3):
"""Returns a 2D Gaussian kernel."""
x = np.linspace(-nsig, nsig, kernlen + 1)
kern1d = np.diff(st.norm.cdf(x))
kern2d = np.outer(kern1d, kern1d)
return kern2d / kern2d.sum()
gkern(5, 2.5) * 273
Output:
array([[ 1.0278445 , 4.10018648, 6.49510362, 4.10018648, 1.0278445 ],
[ 4.10018648, 16.35610171, 25.90969361, 16.35610171, 4.10018648],
[ 6.49510362, 25.90969361, 41.0435344 , 25.90969361, 6.49510362],
[ 4.10018648, 16.35610171, 25.90969361, 16.35610171, 4.10018648],
[ 1.0278445 , 4.10018648, 6.49510362, 4.10018648, 1.0278445 ]])
The Gaussian kernel is a normalized radial basis function to solve partial differential equations. In Numpy, the Gaussian kernel is represented by a 2-dimensional NumPy array.
The 2-dimensional array is called the kernel matrix
. The Gaussian kernel matrix can be obtained using the np.exp(x)
function on a NumPy array.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn