Mastering Numerical Solutions With Fsolve in Python
-
Introduction to
fsolve
in Python -
Use the
fsolve
Function to Find Solutions in Python -
Practical Applications of the
fsolve
Function - Tips for Effective Usage
- Conclusion
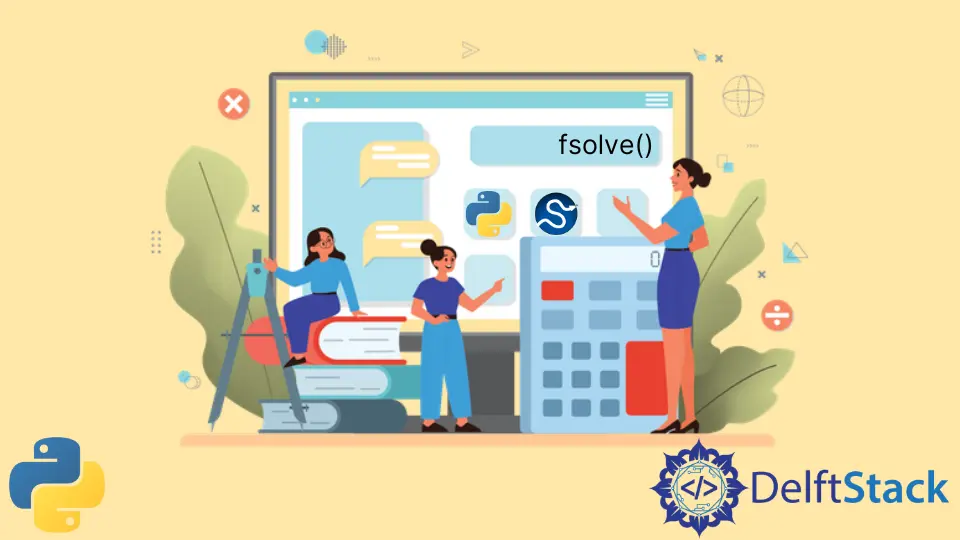
Numerical problem-solving is a common challenge in scientific computing, engineering, and various fields of data analysis. Python, being a versatile and powerful programming language, provides numerous tools for tackling such problems.
One key tool in the realm of numerical solutions is the fsolve
function from the scipy.optimize
module in Python. In this article, we’ll embark on a detailed exploration of fsolve
, uncovering its syntax, applications, and practical examples.
This article will explore how we can use fsolve
to find a solution in Python. We will also be exploring the scenarios where it is used and some sample code to understand better how and when it can be used to reach a certain kind of outcome.
Let’s begin by understanding what fsolve
is and why it is used.
Introduction to fsolve
in Python
Equations are the roots of data science, and they help Data Scientists, Mathematicians, Chemical Engineers, and Physicians make sense of the various scenarios they deal with daily. Now, with computers enveloped in our everyday lives, it gets harder to solve equations based on a larger scale sequentially.
Overview of scipy.optimize
The scipy.optimize
module in SciPy, a scientific computing library for Python, offers a collection of optimization algorithms, root-finding routines, and minimization tools. Among these, the fsolve
function is specifically designed for solving systems of nonlinear equations.
What Is fsolve
fsolve
is a powerful numerical solver used for finding the roots of a system of nonlinear equations. It employs iterative methods to approximate the solutions, making it particularly useful when analytical solutions are challenging or impossible to obtain.
Python’s fsolve
makes it easier for professionals and others to solve equations using different modules that the Python libraries offer. fsolve
is essentially a SciPy module that returns the roots of nonlinear equations.
Syntax
The basic syntax of fsolve
is as follows:
scipy.optimize.fsolve(
func,
x0,
args=(),
fprime=None,
full_output=0,
col_deriv=0,
xtol=1.49012e-08,
maxfev=0,
band=None,
epsfcn=None,
factor=100,
diag=None,
)
There are various parameters that fsolve
offers for various scenarios. You can find a detailed explanation for all parameters and what each entails in the SciPy documentation.
We will, however, be going through a brief yet easy-to-understand summary of these parameters:
Parameter | Description |
---|---|
func , callable f(x, *args) |
This is essentially the description of a function that takes one or more, possibly vector arguments, and returns a value with the same length as the argument. |
x0 , ndarray |
This argument signifies what the initial estimate for the roots of the function f(x)=0 is. |
args , tuple (optional) |
These are any extra arguments that may be required for the function. |
fprime , callable f(x, *args) (optional) |
This is a function for computing the estimated value of the function’s Jacobian with the derivatives across the rows. |
full_output , bool (optional) |
This returns any optional output values if a condition is satisfied or True . |
col_deriv , bool (optional) |
Via this argument, you specify whether or not the Jacobian function computes the derivatives down the columns. According to the SciPy documentation, this is faster because of the absence of a transpose operation. |
xtol , float (optional) |
This argument will allow the function to terminate a calculation based on the most xtol of the relative error between two consecutive iterated values. |
maxfev , int (optional) |
This defines the maximum number of calls to the function. |
band , tuple (optional) |
This is for when fprime is set to None . The Jacobi matrix is considered banded if the argument is set to a two-sequence containing the number of the sub and super diagonals within the matrix. |
epsfcn , float (optional) |
If fprime is set to None , this argument will contain the suitable length of steps for the approximation of the forward difference of the Jacobian. The relative errors in the functions are assumed to be of the order of the machine precision if the epsfcn is less than the machine precision. |
factor , float (optional) |
This argument determines the initial step bound and must be between (0.1, 100). |
diag , sequence (optional) |
These N-positive entries serve as a scale factor for the variables. |
Use the fsolve
Function to Find Solutions in Python
As you can probably tell by now, fsolve
can be used for various nonlinear equations in different scenarios. Let’s explore some simple code samples to get a better grip on how fsolve
may be used:
Basic Example of Using the Python fsolve
Function
Let’s delve into a simple example to demonstrate how fsolve
works. Consider a system of two nonlinear equations:
from scipy.optimize import fsolve
# Define the system of equations
def equations(vars):
x, y = vars
eq1 = x**2 + y**2 - 1
eq2 = x - y
return [eq1, eq2]
# Initial guess
initial_guess = [0.5, 0.5]
# Solve the system of equations
result = fsolve(equations, initial_guess)
print("Solution:", result)
Output:
Solution: [0.70710678 0.70710678]
This code utilizes the fsolve
function from the scipy.optimize
module to find the numerical solution to a system of nonlinear equations. The system of equations is defined in the equations
function.
The fsolve
function is then employed to find values for x
and y
that satisfy both equations simultaneously. The initial guess for the solution is set to [0.5, 0.5]
.
The result of the optimization, i.e., the values of x
and y
that satisfy the equations, is stored in the result
variable and printed to the console.
The code demonstrates the use of numerical optimization techniques, particularly the fsolve
function, to find solutions to a system of nonlinear equations.
Find the Roots of the Equation x+2cos(x)
With a Starting Point of -0.2
Example Code:
from math import cos
import scipy.optimize
def func(x):
y = x + 2 * cos(x)
return y
y = scipy.optimize.fsolve(func, 0.2)
print(y)
Output:
[-1.02986653]
In this code, we import the cos
function from the vast math
library in Python and optimize
from scipy
(source of fsolve
). Next, we define our equation and store it in y
in the func
method.
After our equation is initialized correctly, we merely use fsolve
to call on the method (func)
containing the equation. In the second argument, we define the starting point for the equation.
The result is then stored in a variable y
, printed for output.
Solve an Equation With Starting Points 0 and 2
Example Code:
from math import cos
import scipy.optimize
def func(x):
y = [x[1] * x[0] - x[1] - 6, x[0] * cos(x[1]) - 3]
return y
x0 = scipy.optimize.fsolve(func, [0, 2])
print(x0)
Output:
[6.49943036 1.09102209]
In this code, we follow the same steps as the previous code by importing cos
and scipy.optimize
from Python’s math
and scipy
libraries. We continue to the next step by defining an equation in a method called func
.
We then call this method an argument in the fsolve
function and provide it with the two starting points, 0 and 2, which are then stored and printed in a variable called x0
.
Find the Roots of the Equation 4sin(y)-4
With the Starting Point 0.3
Example Code:
from math import sin
import scipy.optimize
def func(y):
x = 4 * sin(y) - 4
return x
x = scipy.optimize.fsolve(func, 0.3)
print(x)
Output:
[1.57079633]
In this example code, we import sin
from the math
library instead of cos
. The structure of the code remains the same as in the examples above, where we also import scipy.optimize
for fsolve
and then define an equation inside a method func
.
We call this method an argument in fsolve
with a starting point of 0.3. The resulting value is stored in the variable x
and printed for output.
Practical Applications of the fsolve
Function
Engineering Problems
fsolve
is invaluable in engineering applications where systems of nonlinear equations frequently arise. It can be applied to optimize parameters in mechanical designs, electrical circuits, or chemical processes.
Physics Simulations
In physics, numerical solutions are often necessary for complex systems of equations. fsolve
can assist in obtaining solutions for physical phenomena described by nonlinear equations.
Financial Modeling
Financial models often involve nonlinear equations representing complex relationships between various factors. fsolve
can be utilized to find equilibrium points or optimal values in financial simulations.
Tips for Effective Usage
- Careful Selection of Initial Guesses: The convergence of
fsolve
can be sensitive to the initial guess. Experiment with different initial guesses to ensure the algorithm converges to the desired solution. - Provide Analytical Derivatives (if available): If you have access to analytical derivatives of your functions, providing them through the
fprime
parameter can significantly improve the performance offsolve
. - Understand Tolerance Parameters:
xtol
andmaxfev
parameters control the convergence criteria. Adjust these parameters based on the specific requirements of your problem.
Conclusion
In this article, we learned that fsolve
is used for finding the roots of nonlinear equations, about the different kinds of parameters fsolve
can take, and what each entails. Furthermore, we went through some working examples of fsolve
and studied how it can be used in your code.
The fsolve
function in the scipy.optimize
module is a powerful tool for solving systems of nonlinear equations in Python. Its ability to provide numerical solutions makes it indispensable in various scientific and engineering domains.
By mastering the usage of fsolve
and understanding its parameters, Python developers can tackle complex numerical problems efficiently and accurately. Whether you are optimizing engineering designs, simulating physical systems, or solving financial equations, fsolve
empowers you to navigate the intricacies of nonlinear systems with confidence.
Now that you’ve gone through the theory and the practical code samples of how fsolve
is used for deriving a solution, it is time to create code of your own to explore the workings of fsolve
further.
We hope you find this article helpful in understanding how to use fsolve
to solve problems in Python.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn