How to Implement Forward Declaration in Python
- The Forward Declaration
- Why We Need Forward Declaration in Python
- Forward Declaration of a Variable in Python
- Forward Declare a Function in Python
- Conclusion
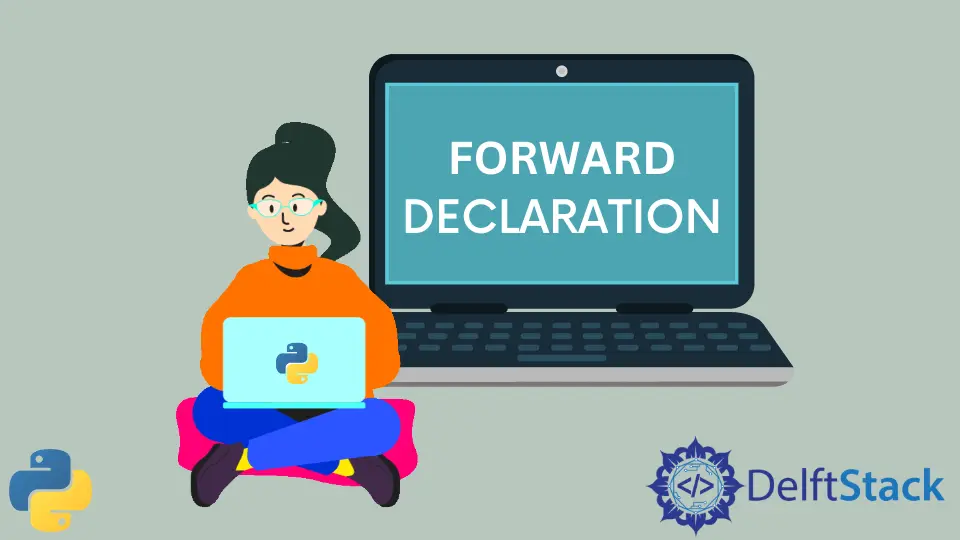
This article will discuss whether we can implement the forward declaration in Python or not. We will also look at different ways to simulate forward declaration in Python.
The Forward Declaration
The forward declaration is the declaration of the signature of a function, class, or variable before implementing the function, class, or variable usage.
In C++, the forward declaration is used to define the signature of a function, as shown below.
#include <iostream>
using namespace std;
int myFun(int, int);
int main() {
cout << myFun(5, 10);
return 0;
}
int myFun(int a, int b) {
int s = a + b;
return s;
}
In the above example, we used forward declaration to define the function myFun
signature using the statement int myFun(int,int);
. After this declaration, the compiler knows that a function named myFun
is present in the source code file.
Therefore, even if the function is called before its definition, the program will not run into any error and will execute successfully.
In Python, we don’t use forward declaration. However, using forward declaration can have various benefits while writing the source code for a Python program.
Why We Need Forward Declaration in Python
Although Python doesn’t have a syntax specification for the forward declaration, there are many situations where we need forward declaration.
If a function Fun1
uses Fun2
before Fun2
is implemented, the person reviewing the code might not know the signature, input, or output of Fun2
. We can avoid this by using a forward declaration to inform the code reviewer about the signature of Fun2
.
-
Sometimes, we might pass objects of user-defined classes to a function. Again, if the class is not implemented before the function, we will need to show the class’s attributes explicitly.
This can be avoided if we use forward declaration in Python.
-
Similar to passing as arguments, if a user-defined class is an attribute of another class, it is crucial to know the behavior of the class object given to understand the code. With forward declaration in Python, we can inform the behavior of the class object.
-
Sometimes, we might need to inform the code reviewer about the data type of the variables. In such cases, we can use forward declarations to define the properties of a variable.
Forward Declaration of a Variable in Python
We can use forward declarations to declare a variable using type hints and the typing module. Let us discuss both of them one by one.
Forward Declaration Using Type Hints in Python
Type hints allow us to define the type of a variable.
Syntax:
var: type
Here,
var
is the variable name.type
is the variable’s data type.
You can use type hints to forward declare a Python as follows.
myStr: str
myNumber: int
In the above example, the variables myStr
and myNumber
have no existence before they are declared. Therefore, using myStr
or myNumber
after the forward declaration using type hints will lead to a NameError
exception, as shown below.
myStr: str
myNumber: int
print(myStr)
print(myNumber)
Output:
Traceback (most recent call last):
File "/home/aditya1117/pythonProject/string12.py", line 3, in <module>
print(myStr)
NameError: name 'myStr' is not defined
Here, the variables myStr
and myNumber
are present. However, they haven’t been assigned a value.
Due to this, neither are they allocated memory nor are they present in the program’s symbol table. Therefore, the NameError
exception occurs when we use the variables without assigning values to them.
To use the variable, you must first assign it a value.
myStr: str
myNumber: int
myStr = "Delft-stack"
myNumber = 123
print(myStr)
print(myNumber)
Output:
Delft-stack
123
Once we assign values to the variables, they are allocated memory and are added to the symbol table. Hence, the program doesn’t run into an error and gets executed successfully.
Here, you can say that forward declaration of a variable using type hints is only as good as a comment.
Forward Declaration Using Typing Module in Python
Defining objects of user-defined classes with type hints needs to be implemented before the variable declaration. Otherwise, the program will run into the NameError
exception.
You can observe this in the following example.
myStr: str
myNumber: int
myObject: myClass
Output:
Traceback (most recent call last):
File "/home/aditya1117/pythonProject/string12.py", line 3, in <module>
myObject: myClass
NameError: name 'myClass' is not defined
Here, the literal myClass
is not defined. Hence, the program runs into the NameError
exception.
To avoid such an error, we first need to define that myClass
is a class name. We can use the NewType()
function defined in the typing
module.
The NewType()
function takes a string representing the class name as its first input argument. It takes the parent class of the new class as the second argument.
After execution, it returns a new function that we can assign to the class name of the new class. After that, we can use the new class name like we use literals like int, float, and double in type hints.
To define a class of the myClass
type, we will pass the literal myClass
as the first input argument and the None
object as the second argument. After executing the NewType()
function, we can use myClass
in type hints for the forward declaration, as shown below.
import typing
myClass = typing.NewType("myClass", None)
myStr: str
myNumber: int
myObject: myClass
The program will not run into the NameError
exception as we have already declared that myClass
is an object type.
Forward Declare a Function in Python
In Python, you should always define a function before using it. You can use the function fun1
inside the definition of another function, fun2
.
However, you need to ensure that fun2
will not be called before defining fun1
. Otherwise, the program will run into the NameError
exception.
fun1()
def fun1():
print("I am in fun1")
Output:
Traceback (most recent call last):
File "/home/aditya1117/string12.py", line 1, in <module>
fun1()
NameError: name 'fun1' is not defined
Process finished with exit code 1
Here, we have used the function fun1()
in the main()
function before its definition. Due to this, the program runs into a NameError
exception showing that the name fun1
is not defined.
By using fun1
only after defining it can avoid the NameError
exception as shown below.
def fun1():
print("I am in fun1")
fun1()
Output:
I am in fun1
Conclusion
This article has discussed how we can simulate forward declaration in Python. Forward declarations are only meaningful when using type hints to declare variables of user-defined types.
Also, type hints are only necessary when enforcing data types on variables. Therefore, use forward declarations only when enforcing type constraints on variables.
Otherwise, your program will do extra work for no reason. It would be best to always define a function before using it in Python.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub