Events in Python
- What are Events in Python?
- Using Tkinter for Event Handling
- Event Handling with Pygame
- Asynchronous Event Handling with asyncio
- Conclusion
- FAQ
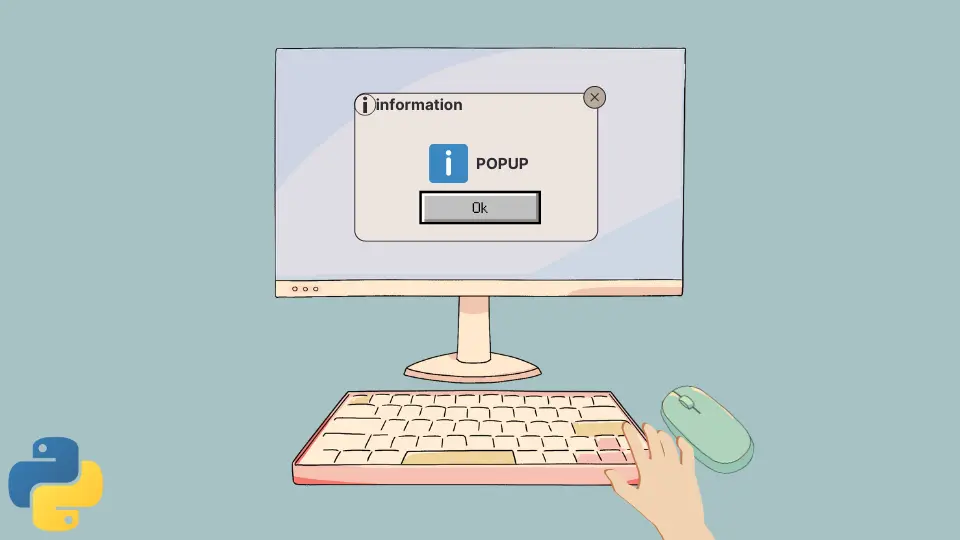
In the realm of programming, events are crucial for creating interactive applications. Python, renowned for its simplicity and versatility, provides robust frameworks for event handling.
This tutorial delves into the concept of events in Python, focusing on how to manage them effectively. Whether you’re building a GUI application with Tkinter or working with asynchronous programming, understanding events will enhance your coding skills. We will explore various methods to handle events in Python, including practical examples that illustrate how to implement these techniques. By the end of this article, you will have a solid grasp of event handling in Python, enabling you to create more dynamic and responsive applications.
What are Events in Python?
Events in Python refer to actions or occurrences that happen during the execution of a program. These can include user interactions like mouse clicks, keyboard presses, or even system-generated events such as timers or signals. Event handling is the process of responding to these events, allowing the program to perform specific actions when an event occurs.
In Python, event handling is often managed through frameworks and libraries that provide the necessary tools to capture and respond to events. For instance, libraries like Tkinter, Pygame, and asyncio are popular choices for handling events in GUI applications and asynchronous programming. Understanding how to work with events is essential for anyone looking to create interactive applications or games.
Using Tkinter for Event Handling
Tkinter is the standard GUI toolkit for Python and is widely used for creating desktop applications. It allows developers to handle events such as button clicks, key presses, and mouse movements seamlessly. Here’s a simple example of how to use Tkinter for event handling:
import tkinter as tk
def on_button_click():
print("Button clicked!")
root = tk.Tk()
button = tk.Button(root, text="Click Me", command=on_button_click)
button.pack()
root.mainloop()
Output:
Button clicked!
In this example, we start by importing the Tkinter library. We define a function, on_button_click
, which will be executed when the button is clicked. The tk.Button
widget is created with a label “Click Me” and is linked to the on_button_click
function through the command
parameter. Finally, we call root.mainloop()
to start the event loop, allowing the application to respond to events.
This simple program demonstrates how easy it is to handle events in Tkinter. When the user clicks the button, the function is triggered, and the message is printed to the console. Tkinter’s event handling is intuitive, making it an excellent choice for beginners looking to develop GUI applications.
Event Handling with Pygame
Pygame is a popular library for creating games in Python, and it has a robust event handling system designed specifically for game development. Events in Pygame can include user input, such as keyboard and mouse actions, as well as system events. Here’s an example of handling events in a Pygame application:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((640, 480))
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((0, 0, 0))
pygame.display.flip()
In this example, we initialize Pygame and create a window with a resolution of 640x480 pixels. The main loop continuously checks for events using pygame.event.get()
. If the user closes the window (triggering the QUIT
event), the program quits gracefully. The screen is filled with black in each iteration, and pygame.display.flip()
updates the display.
Pygame’s event handling is efficient and allows for real-time responses to user inputs, making it ideal for game development. By understanding how to manage events in Pygame, developers can create engaging and interactive gaming experiences.
Asynchronous Event Handling with asyncio
Asynchronous programming in Python is another area where event handling plays a significant role. The asyncio
library allows developers to write concurrent code using the async/await syntax, making it easier to handle events in a non-blocking manner. Here’s a basic example of using asyncio for event handling:
import asyncio
async def handle_event():
print("Event handled!")
async def main():
while True:
await handle_event()
await asyncio.sleep(1)
asyncio.run(main())
Output:
Event handled!
Event handled!
Event handled!
...
In this code snippet, we define an asynchronous function handle_event
, which simulates handling an event by printing a message. The main
function runs an infinite loop, calling handle_event
and then sleeping for one second. The asyncio.run(main())
command starts the event loop.
Using asyncio
for event handling allows for more efficient resource use, especially in applications that require handling multiple events concurrently. This approach is particularly useful for web servers or applications that involve I/O-bound tasks.
Conclusion
Understanding events and event handling in Python is essential for creating interactive applications, whether they are GUI-based or game-oriented. By utilizing libraries like Tkinter, Pygame, and asyncio, developers can effectively manage events and respond to user interactions. This tutorial provided an overview of different methods to handle events in Python, complete with practical examples. As you continue your programming journey, mastering event handling will empower you to build more dynamic and responsive applications.
FAQ
-
What are events in Python?
Events in Python are actions or occurrences that happen during the execution of a program, such as user interactions or system-generated signals. -
How can I handle events in a GUI application?
You can handle events in a GUI application using libraries like Tkinter, which allows you to create interactive elements and respond to user actions. -
What is Pygame, and how does it handle events?
Pygame is a library for creating games in Python. It provides a robust event handling system that allows developers to respond to user inputs and system events efficiently. -
How does asyncio work for event handling?
Asyncio is a library for asynchronous programming in Python. It allows developers to write non-blocking code using the async/await syntax, enabling efficient event handling for I/O-bound tasks. -
Can I use event handling in web applications with Python?
Yes, you can use event handling in web applications using frameworks like Flask or Django, which can handle HTTP requests and user interactions.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn