The which Command in Python
-
Method 1: Using the
shutil
Module -
Method 2: Using the
os
Module -
Method 3: Using the
subprocess
Module - Conclusion
- FAQ
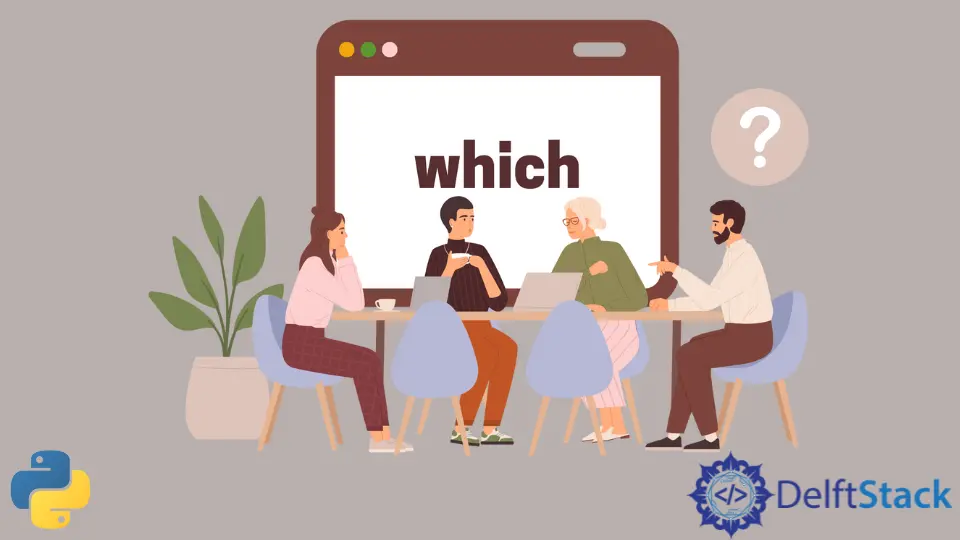
When working in a command-line environment, the which
command is a handy tool that helps you locate the executable file associated with a given command. But what if you’re working within Python and need to achieve similar functionality?
This tutorial dives into the equivalent of the which
command in Python, providing you with practical methods to find the paths of executables and scripts. Whether you’re a seasoned developer or a newcomer, understanding how to implement this in Python can streamline your workflow and enhance your productivity. Let’s explore the methods you can use to replicate the which
command functionality in Python.
Method 1: Using the shutil
Module
Python’s shutil
module provides a simple way to locate executables. The shutil.which()
function is directly analogous to the which
command in Unix-like systems. It returns the path of the executable if it exists in the user’s PATH
environment variable, or None
if it doesn’t.
Here’s how you can use it:
import shutil
executable_name = 'python'
executable_path = shutil.which(executable_name)
print(executable_path)
Output:
/usr/bin/python
The code above imports the shutil
module and uses the which()
function to find the path of the specified executable, in this case, python
. If the executable is found, it returns its path; otherwise, it returns None
. This method is straightforward and efficient, making it an excellent choice for quickly locating executables in your Python scripts.
Method 2: Using the os
Module
Another way to mimic the functionality of the which
command is by using the os
module. You can manually check each directory listed in the PATH
environment variable to see if the executable exists. This method gives you more control and allows for customization if needed.
Here’s an example:
import os
def find_executable(executable_name):
for path in os.environ["PATH"].split(os.pathsep):
full_path = os.path.join(path, executable_name)
if os.path.isfile(full_path) and os.access(full_path, os.X_OK):
return full_path
return None
executable_name = 'python'
executable_path = find_executable(executable_name)
print(executable_path)
Output:
/usr/bin/python
In this code snippet, we define a function called find_executable
, which iterates through each directory in the PATH
. It constructs the full path to the executable and checks if it exists and is executable. If found, it returns the path; if not, it returns None
. This method is particularly useful when you want to implement additional checks or custom logic while searching for executables.
Method 3: Using the subprocess
Module
If you want to directly invoke the which
command from within Python, you can use the subprocess
module. This method allows you to call shell commands and capture their output, effectively leveraging the existing functionality of the command line.
Here’s how to do it:
import subprocess
def which_command(executable_name):
try:
result = subprocess.run(['which', executable_name], stdout=subprocess.PIPE, text=True, check=True)
return result.stdout.strip()
except subprocess.CalledProcessError:
return None
executable_name = 'python'
executable_path = which_command(executable_name)
print(executable_path)
Output:
/usr/bin/python
In this example, we define a function called which_command
that uses subprocess.run()
to execute the which
command. It captures the output and returns the path of the executable. If the command fails, it handles the exception and returns None
. This method is particularly useful when you want to retain the behavior of the shell command while working within Python, making it a powerful tool in your arsenal.
Conclusion
Understanding how to replicate the which
command in Python can significantly enhance your scripting capabilities. Whether you choose to use the shutil
module, manually check the PATH
with the os
module, or call the command directly via subprocess
, each method has its advantages. By implementing these techniques, you can efficiently locate executables and streamline your development process. So, the next time you’re in need of a command’s path, you’ll know exactly how to find it using Python.
FAQ
-
What is the
which
command used for?
Thewhich
command is used to locate the executable file associated with a command in the command line. -
Can I use the
which
command in Windows?
Yes, Windows has a similar command calledwhere
, which serves the same purpose aswhich
. -
Is using
shutil.which()
the best method?
For most use cases, yes, as it is simple and efficient. However, you can choose other methods based on your specific needs. -
Can I find executables in directories not listed in
PATH
?
The methods discussed focus on thePATH
variable, but you can modify them to search specific directories if needed. -
What happens if the executable is not found?
Each method will returnNone
if the executable is not found, allowing you to handle the situation gracefully in your code.