Enum in Python
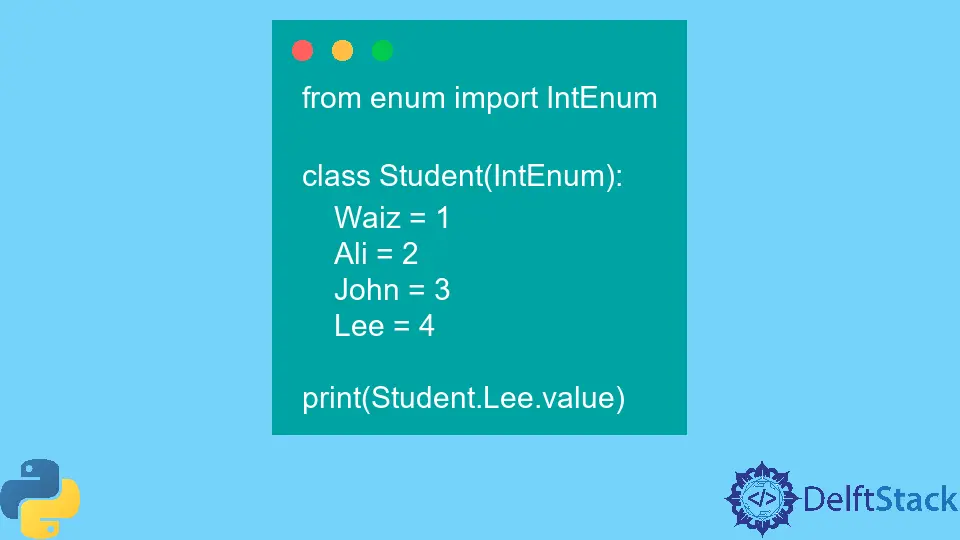
In this tutorial, we will look into different methods of using or implementing the enum
in Python. The enum
or enumeration is a special type of class representing a set of named constants of numeric type. Each named constant in the enum
type has its own integer value, which depends on the set’s named constant position.
The enum
type is used to assign the constant names to the set of integer values in many programming languages like C#, Java, Go, C and C++, etc. We can implement and use the enumeration type in Python by using the methods which are explained below.
Enum in Python Using the enum
Module
The enum
module of Python has four classes which are Enum
, IntEnum
, Flag
, and IntFlag
, for further details about the module and classes, this link can be visited.
In the Enum
class, the value of each name constant is an integer by default, but we can also pass datatypes like string or float, etc. The values of name constants of the IntEnum
class, as the name suggests, can only be integers.
We can create and use the enumeration in Python using the Enum
and IntEnum
classes. The below example codes demonstrate how to use these classes to implement different types of enumerators in Python.
Simple enumerator:
from enum import Enum
Names = Enum("Names", ["Waiz", "Tom", "Sara", "Lee"])
We can use the iterator of the (name, value)
pair to save specific values with each name, representing id or marks, etc., of the name constants. We can access the name constant value and name using the Enum.nameconstant.value
to access values and Enum.nameconstant.name
to access names.
We can do so in Python using the below example code.
from enum import Enum
Names = Enum("Names", [("Waiz", 8), ("Tom", 5), ("Sara", 7), ("Lee", 6)])
print(Names.Sara.value)
print(Names.Waiz.name)
Output:
7
Waiz
Another way to create an enumerator is by using the class syntax and then passing the Enum
or IntEnum
as shown in the below example code:
from enum import IntEnum
class Student(IntEnum):
Waiz = 1
Ali = 2
John = 3
Lee = 4
print(Student.Lee.value)
Output:
4
Implement Enum in Python Using a Regular Class Syntax
In Python versions older than 2.4, the Enum
module is not available, in that case, we can implement our own class of the enumerator Enum
using the class syntax.
We can implement the simple enumerator class with the default values starting from 1
as in the Enum
class of enum
module, as demonstrated in the below example code:
class Zoo:
Lion, Tiger, Cheetah, Leopard = range(1, 5)
print Zoo.Lion
Output:
1