How to Do Nothing Inside an if Statement in Python
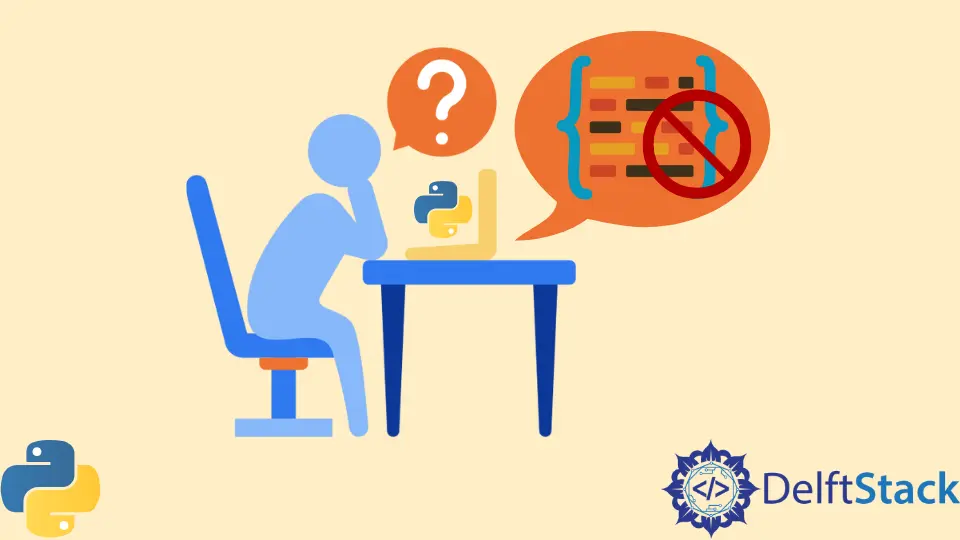
In Python, the if
statement is a fundamental part of the language, enabling the execution of specific code blocks based on certain conditions. However, there might be instances where you want your program to take no action when a condition is met.
This concept of doing nothing inside an if
statement might seem counterintuitive, but it’s a useful and often necessary practice in programming.
Before we delve into how to do nothing inside an if
statement, let’s consider why you might want to do this in your code. There are several scenarios in which doing nothing is a legitimate requirement:
-
Default Behavior: You may want to specify a default behavior when a certain condition is not met. In such cases, doing nothing inside an
if
block effectively means continuing with the default action. -
Testing and Debugging: When testing or debugging your code, you may want to temporarily disable a block of code without removing it entirely. Doing nothing in an
if
statement can help you achieve this. -
Future Use: You might be planning to implement functionality in the future, and you want to leave a placeholder for it in your code. Doing nothing allows you to indicate that there’s a condition that should be addressed later.
Use the pass
Statement to Perform Nothing in Python
Consider a scenario where you’re evaluating a condition, and based on its outcome, you want your code to explicitly do nothing. In Python, accomplishing this is simple and involves using the pass
statement.
The pass
statement in Python acts as a placeholder. It is a null operation or a no-op statement.
It doesn’t perform any action and is typically used when the syntax requires a statement, but you don’t want any particular action to occur.
Let’s dive into a few examples to understand how to do nothing inside an if
statement using the pass
statement:
Example 1: Basic if
Statement
x = 10
if x > 5:
pass # This block will do nothing if x is greater than 5
else:
print("x is not greater than 5")
Output:
# If x is greater than 5, there is no output.
# If x is 5 or less, the output will be:
x is not greater than 5
In this scenario, a variable x
is initialized with the value 10
. The code then features an if
statement, which serves to evaluate whether the value of x
is greater than 5
.
If x
is indeed greater than 5
, the code within the if
block is intended to execute. However, in this specific instance, the if
block contains a pass
statement, signifying that absolutely nothing will occur when x
exceeds 5
.
Conversely, if x
is less than or equal to 5
, the else
block comes into play. Inside the else
block, a print
statement is used to display the message x is not greater than 5
.
The outcome of this code hinges on the value of x
: if it’s greater than 5
, there will be no output, while if it’s 5
or less, the code will produce a message.
Example 2: Complex Conditional Checks
condition = True
some_other_condition = False
if condition:
# Perform some checks or operations
if some_other_condition:
pass # Do nothing in this block based on the secondary condition
else:
# Carry out specific actions
print("Secondary condition is not met")
else:
print("Main condition is not true")
Output:
# If 'condition' is True and 'some_other_condition' is False, the output will be:
Secondary condition is not met
# If 'condition' is False, the output will be:
Main condition is not true
In this code example, we encounter more complex conditional checks. There are two variables defined: condition
is set to True
, and some_other_condition
is set to False
.
The code begins with an outer if
statement, which examines the value of condition
. If the condition
is True
, it triggers a series of further checks or operations within the indented if
block.
Inside this block, there is another if
statement that evaluates some_other_condition
. If some_other_condition
is True
, we encounter the pass
statement, signifying that no action is taken within this inner block.
On the other hand, if some_other_condition
is False
, the code proceeds to the else
block, where specific actions are executed. In this case, a message, Secondary condition is not met
, is printed.
If the outermost condition (condition
) is False
, the code jumps straight to the else
block, and it prints the message Main condition is not true
.
Example 3: Placeholder for Future Code
user_input = input("Enter your choice: ")
if user_input == "A":
# Placeholder for future functionality
pass # Additional functionality for choice 'A' to be added later
elif user_input == "B":
# Perform actions for choice 'B'
print("You chose option B")
else:
print("Invalid choice")
Output:
# If the user enters 'A', there is no immediate output. Additional functionality for 'A' is expected in the future.
# If the user enters 'B', the output will be:
You chose option B
# If the user enters any other input, the output will be:
Invalid choice
In this code snippet, the program prompts the user to input a choice, which is stored in the user_input
variable. Subsequently, an if
statement is employed to determine what action to take based on the user’s input.
If the user enters A
, the code enters the if
block. Inside this block, there’s a pass
statement, indicating that this part of the code is a placeholder for future functionality, and no immediate action is taken for choice A
.
If the user enters B
, the program proceeds to the elif
block and executes the code within. In this case, it prints the message You chose option B
to confirm that the user selected option B
.
If the user enters any other input, the else
block is executed, and the message Invalid choice
is printed to inform the user that their input is not recognized.
Conclusion
In Python, the pass
statement serves as a tool to maintain the structure of code when a specific action is not necessary. It helps in scenarios where the syntax requires a statement, but no action needs to be performed within a certain code block.
While doing nothing might seem inconsequential, the pass
statement plays a crucial role in maintaining the integrity of the code’s structure, allowing for future expansions and ensuring a more readable and understandable codebase.
Understanding when and how to use the pass
statement inside an if
block is essential for writing clean, maintainable, and robust Python code.
In conclusion, the art of doing nothing in Python using the pass
statement within an if
statement is a practice that enhances code readability, facilitates future enhancements and ensures the structural integrity of your program.