The Difference Between len() and sys.getsizeof() in Python
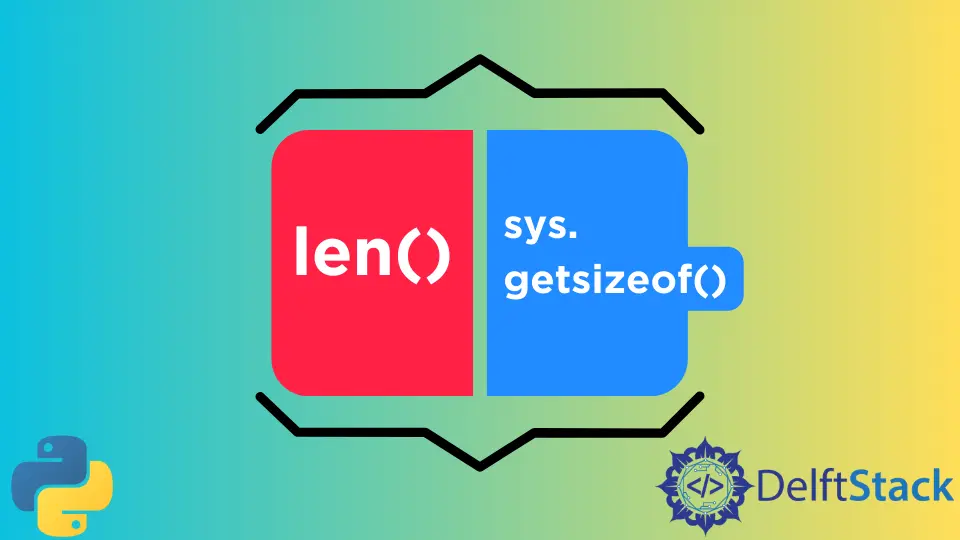
From a glance, the len()
and sys.getsizeof()
functions in Python seem to have the same functionality of finding the length of objects. Contrary, these two methods have different semantic meanings altogether when implemented.
the len()
Function in Python
The len()
function is used to query for an object’s number of items. The object may be an array, queue, tuple, or list. If this method is called on a string, it returns the number of characters in that particular string.
Example:
print(len("Batman"))
Output:
6
6 is the total number of characters making the Batman string.
When you call the len()
function on a list of items like below, it will return the total number of items that make up the list.
animals = ["Koala", "Guineapig", "Bear", "Zebra", "Giraffe"]
print(len(animals))
Output:
5
The animal list contains a total of five animals as the output that is returned.
the sys.getsizeof()
Function in Python
The sys module in Python provides a sys.getsizeof()
function, which essentially returns the memory size of an object that is passed to it in the unit of bytes. The object’s memory consumption is what is accounted for instead of the memory consumption the object refers to. This function is implementation specific, therefore, when built-in objects are passed to it, it returns correct results. Otherwise, it may return false results for third-party extensions.
sys.getsizeof()
Syntax
sys.getsizeof(object[, default])
While the object argument is the item to find the size of, the default argument lets you define a value that will be returned if the object argument does not retrieve the size. In case the object fails to retrieve the size and a default value is not provided, a TypeError
exception is raised.
When you use the sys.getsizeof()
function, it calls the object’s _sizeof_
method, and then it adds an extra garbage collector overhead if the garbage collector manages the object.
Example:
import sys
print(sys.getsizeof("hello"))
Output:
54
The output is in bytes
. This is the total memory size hello
occupies.
The empty string even takes 49 bytes in the memory.
import sys
print(sys.getsizeof(""))
Output:
49
The main difference that exists between len()
and sys.getsizeof()
function is that the former returns the actual length of elements within a container while the latter returns the memory size this object occupies.