Buffer in Python
-
Use the
buffer()
Function to Implement the Buffer Interface in Python -
Use the
memoryview()
Function to Implement the Buffer Interface in Python
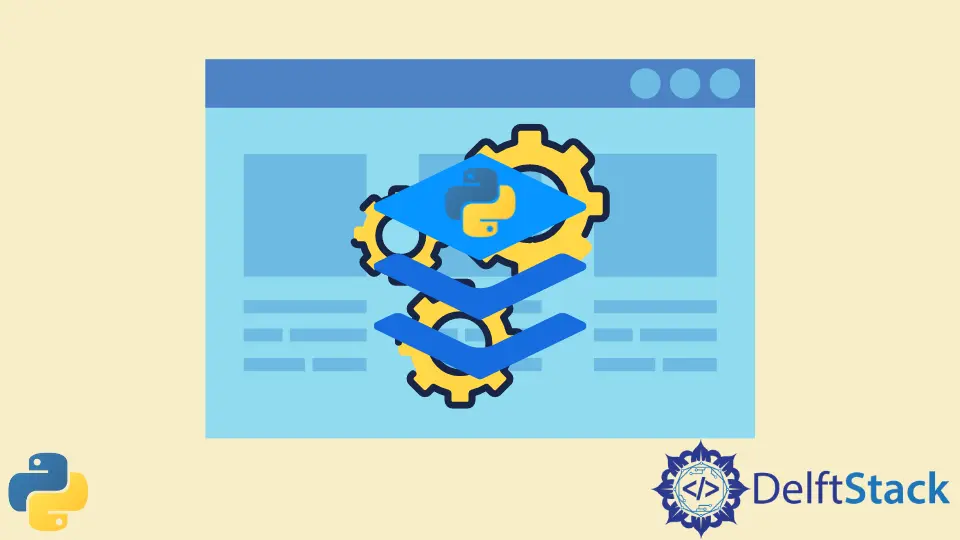
In Python, the buffer
type object is used to show the internal data of a given object in a byte-oriented format. Python’s main use of buffers is storing and manipulating huge data arrays and processing them without creating copies.
The buffer
interface is only supported by strings
, Unicode
, arrays
, and bytearrays
. The numpy
arrays also use this interface in the background.
We can work on the same buffer
instances without creating copies of data using the buffer
interface.
import numpy as np
arr = np.array([1, 2, 3])
arr2 = np.asarray(arr)
arr2[2] = 50
print(arr, arr2)
Output:
[ 1 2 50] [ 1 2 50]
In the above example, we create a numpy
array called arr
and, using this, we create another array called arr2
.
Because the numpy
module supports the buffer protocol and the data is sent using views of the array rather than generating a new array, updating the array arr2
also updates the original arr
.
Let us implement this interface on supported objects using the buffer()
and memoryview()
function.
Use the buffer()
Function to Implement the Buffer Interface in Python
With the buffer()
function, we can return a given object’s read-only view objects that support the buffer interface (strings
, arrays
, bytes
, Unicode
, bytesarray
). It is useful when working with huge data arrays because it eliminates copying the data.
Example:
a = "Sample String"
bf = buffer(a, 2, 5)
print bf, type(bf)
Output:
mple <type 'buffer'>
We created a buffer
type object in the above example. This object returns a view of the string a
.
However, the memoryview()
function replaced the buffer()
function in Python 3. Python 2.7 supports both functions.
Use the memoryview()
Function to Implement the Buffer Interface in Python
In Python 3, the memoryview()
function is used to return a memoryview
object that implements the buffer
interface and creates a view of an object that supports this interface.
memoryview()
takes the bytes-like object and returns its view. On printing, it shows the memory location of the original object.
a = bytearray("Sample Bytes", "utf-8")
m = memoryview(a)
print(m[2], type(m), m)
Output:
109 <class 'memoryview'> <memory at 0x7f83f2d5f940>
In the above example, we created a memoryview
object of an encoded bytesarray
object.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn