Bilateral Filtering in Python
- What Is Bilateral Filtering
- Steps to Perform Bilateral Filtering in Python
-
the
bilateralFilter()
Function in Python -
Use the
bilateralFilter()
Function to Perform Bilateral Filtering in Python
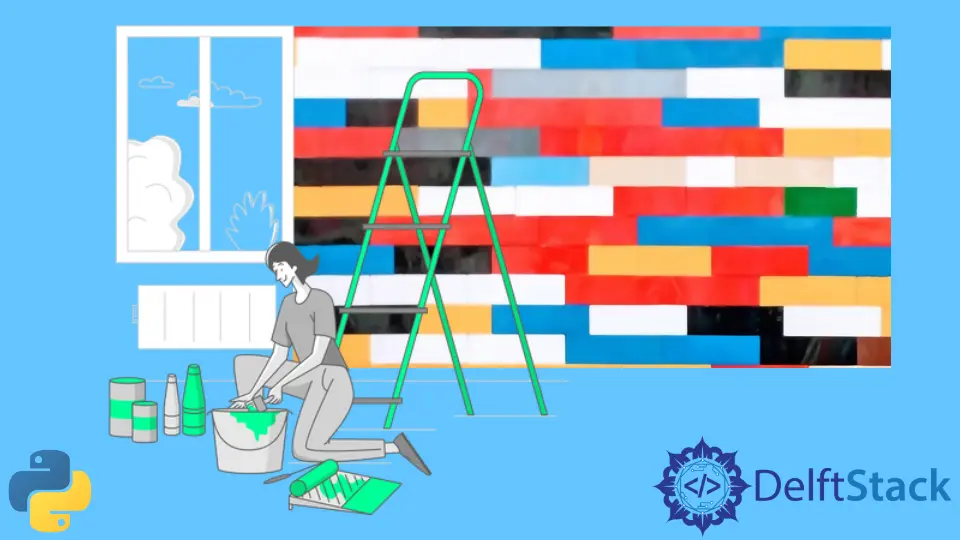
Filtering is used to process images in Computer Vision applications. This article will discuss the implementation of bilateral filtering in Python using the OpenCV module.
What Is Bilateral Filtering
Bilateral filtering is a smoothing filtering technique. It is a non-linear and noise-reducing filter that replaces each pixel value with the weighted average pixel value of the neighbors.
Bilateral filtering is also called edge-preserving filtering as it doesn’t average the pixel across edges.
Steps to Perform Bilateral Filtering in Python
To perform bilateral filtering, we mainly perform four tasks.
-
We replace each pixel in the image with the weighted average of its neighbors.
-
Each neighbor’s weightage is decided by its distance from the current pixel. We assign each pixel a weight where the nearest pixels get the highest weightage, and distant pixels are assigned the lowest weight.
To perform this task, we use a spatial parameter.
-
The neighbor’s weightage also depends on the difference in intensity of the pixels. Pixels with similar intensity to the current pixel are assigned more weight, whereas pixels with large intensity differences are assigned lesser weights.
To perform this task, we use a range parameter.
-
By increasing the spatial parameter, you can smoothen the larger features of the image. On the other hand, if you increase the range parameter, bilateral filtering behaves as Gaussian filtering.
the bilateralFilter()
Function in Python
We can perform bilateral filtering in Python using the OpenCV module using the bilateralFilter()
function. The syntax for bilateralFilter()
function is as follows.
bilateralFilter(src, d, sigmaColor, sigmaSpace, borderType)
Here,
-
The parameter
src
takes the source image that has to be processed as an input argument. -
The parameter
d
takes the diameter of the neighborhood in which the pixels are to be considered while filtering. -
The parameter
sigmaColor
is the value of the filter sigma in the colorspace. Having a higher value ofsigmaColor
means that the colors farther apart in the color space are considered while filtering.The parameter
sigmaColor
should contain a value in the range ofsigmaSpace
. -
The parameter
sigmaSpace
denotes the value of sigma in the spatial domain. A higher value ofsigmaSpace
means that the pixels farther away from the current pixel are considered while filtering.The parameter
sigmaSpace
should contain a value in the range ofsigmaColor
. -
The parameter
borderType
is used to define a mode for extrapolating the pixels outside the image while filtering the pixels in image boundaries.
Use the bilateralFilter()
Function to Perform Bilateral Filtering in Python
The following are the steps to perform bilateral filtering in Python.
-
First, we will import
cv2
. -
Next, we will open an image using the
imread()
function, which takes the file path of an image as its input argument and returns an array representing the image. -
We will store the array in a variable
img
. -
After loading the image, we will use the
bilateralFilter()
function to perform bilateral functioning in Python. After execution, thebilateralFilter()
function returns an array containing the processed image. -
After obtaining the processed image, we will save it to the file system using the
imwrite()
function, which takes a string containing the filename of the output file as its first input argument and the array containing the processed image as its second input argument. After executing the function, the file is saved to the file system.
Below is the image we will use to perform bilateral filtering in Python.
The following is the code to perform bilateral filtering in Python.
import cv2
img = cv2.imread("pattern.jpg")
output_image = cv2.bilateralFilter(img, 15, 100, 100)
cv2.imwrite("processed_image.jpg", output_image)
Here is the output image after performing bilateral filtering on the input image:
In the given image, you can observe that the features of the strips have been blurred in the output image. This is because the neighboring pixels of each pixel are considered while creating the output pixel.
Averaging of the pixels gives a blurring effect, and the features are blurred.
When compared to Gaussian filtering, bilateral filtering preserves the edges. Therefore, while performing smoothing operations, you can always use bilateral filtering if you need to preserve the edges in your image.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub