How to Create an Auto Clicker in Python
-
Use the
pyautogui
Module to Create an Auto Clicker in Python -
Use
win32api
to Create an Auto Clicker in Python -
Use the
pynput
Module to Create an Auto Clicker in Python - Conclusion
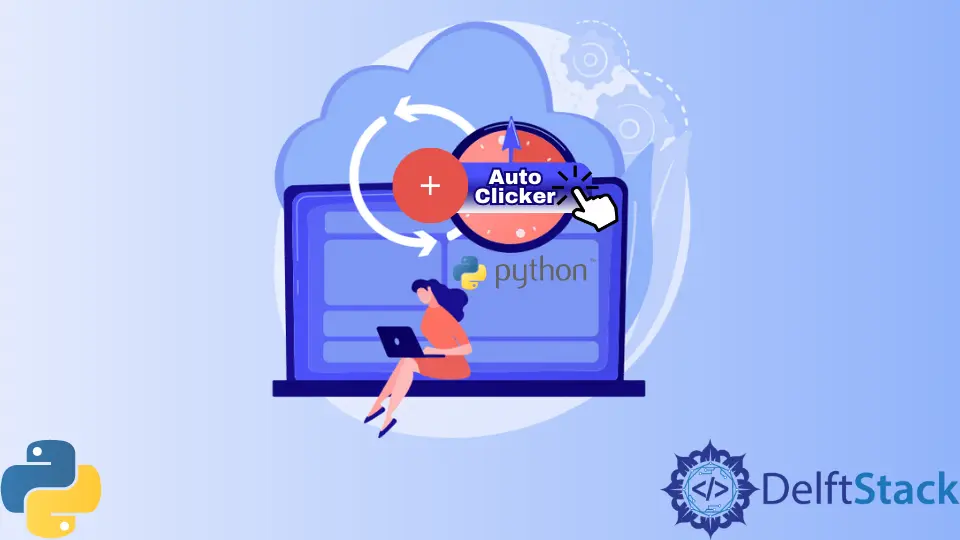
As the name suggests, an auto clicker in Python is a simple Python application that repeatedly clicks the mouse as per the user requirement. Different parameters, like speed, frequency, and position, can be altered depending on the user.
Python has different modules available to control devices like a keyboard, mouse, etc. Thus, we can easily create an auto clicker in Python using these modules.
This tutorial will demonstrate different ways to create an auto clicker in Python.
Use the pyautogui
Module to Create an Auto Clicker in Python
The pyautogui
module can create scripts that control the mouse and keyboard of the device.
We can use the pyautogui.click()
function to click the mouse. We can move the mouse beforehand to the required position using the pyautogui.moveTo()
function and specify the coordinates for the cursor.
To create an auto clicker with these functions, we will move the mouse and run a for
loop to execute every iteration’s pyautogui.click()
function. We will also slightly delay before every click to make this visible.
We will use the sleep()
function from the time
module for the delay.
We implement the logic for auto clicker in the code below.
import pyautogui
import time
pyautogui.moveTo(600, 800)
for i in range(20):
time.sleep(0.1)
pyautogui.click()
In the above example, we move the cursor to coordinates (600,800)
and click the mouse 20 times using the click()
function.
Use win32api
to Create an Auto Clicker in Python
The win32api
is used to control and automate Windows COM objects. We can use these objects from the win32con
module and mimic a mouse click.
For this, we use the win32api.mouse_event
function twice, once for win32con.MOUSEEVENTF_LEFTDOWN
and the other for win32con.MOUSEEVENTF_LEFTDOWN
to mimic a mouse click.
We will set the cursor’s position to the required coordinates with the win23api.SetCursorPos()
function. Then, we will use a similar approach as we did in the previous method to create an auto clicker in Python.
See the code below.
import win32api
import win32con
import time
win32api.SetCursorPos((600, 800))
for i in range(20):
win32api.mouse_event(win32con.MOUSEEVENTF_LEFTDOWN, 600, 800, 0, 0)
win32api.mouse_event(win32con.MOUSEEVENTF_LEFTUP, 600, 800, 0, 0)
time.sleep(0.1)
Use the pynput
Module to Create an Auto Clicker in Python
We also have the pynput
for automating mouse and keyboard actions in Python scripts. This method differs from the previous two, as we will create a class with the necessary functionalities to create an auto clicker.
We will create a class that will act as an auto clicker when a specific key is pressed. Another key will be assigned to stop the clicking.
We will need to import both pynput.mouse
and pynput.keyboard
modules.
The class created will extend to the threading.Thread
class so that we can control the threads with different functionalities.
In the __init__
method of the class, we will specify a slight delay between every click as we did previously and mention that we will click the left button of the mouse using the Button.Left
object.
The threads will keep running until the key to stopping clicking is pressed. We will use the start()
method of the threading.Thread
class to start clicking.
In the running()
method, we will also use the Controller
class object.
To start and stop the threads, we will create a function. The Listener()
function will execute the defined function while pressing the key using the on_press
argument.
This logic is implemented in the code below.
import time
import threading
from pynput.mouse import Button, Controller
from pynput.keyboard import Listener, KeyCode
start_end = KeyCode(char="a")
exit_key = KeyCode(char="z")
class auto_clicker_class(threading.Thread):
def __init__(self):
super(auto_clicker_class, self).__init__()
self.delay = 0.1
self.button = Button.left
self.running = False
self.program_run = True
def begin_clicking(self):
self.running = True
def clicking_stop(self):
self.running = False
def exit(self):
self.clicking_stop()
self.program_run = False
def run(self):
while self.program_run:
while self.running:
mouse_ob.click(self.button)
time.sleep(self.delay)
time.sleep(0.1)
mouse_ob = Controller()
t = auto_clicker_class()
t.start()
def fun(k):
if k == start_end:
if t.running:
t.clicking_stop()
else:
t.begin_clicking()
elif k == exit_key:
t.exit()
listener.stop()
with Listener(on_press=fun) as listener:
listener.join()
Conclusion
We discussed auto clickers in this tutorial. The first two methods are relatively simple as we execute a function repeated several times using the for
loop to mimic a mouse click.
The final method creates a proper auto clicker script in Python that can start and stop clicking based on the key pressed on the keyboard.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn