The __Contains__ Method in Python
- Understanding Membership Testing
-
the
__contains__
Method in Python -
Customize the
__contains__
Method in Python -
Use the
__contains__
Method in Strings -
Overload the
__contains__
Method in Classes - Conclusion
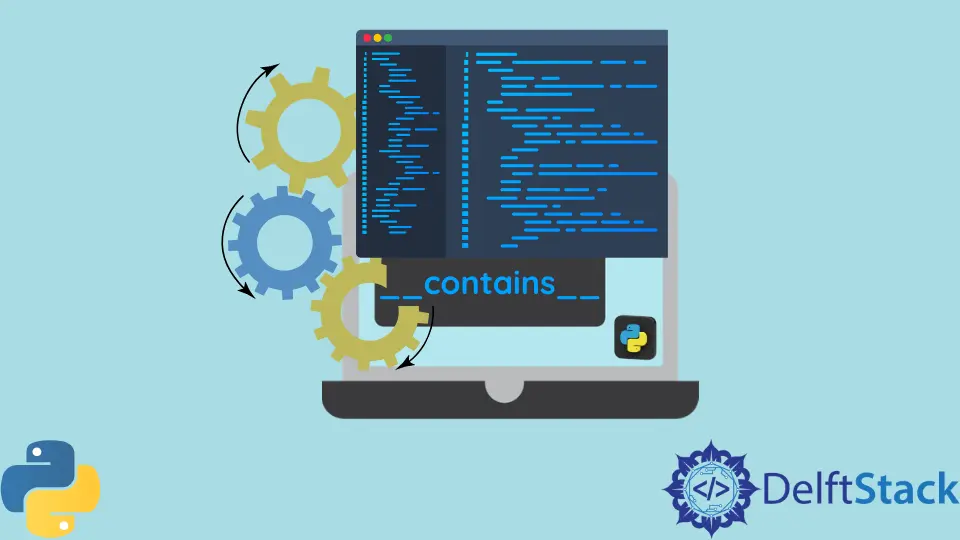
One of the fundamental operations in programming is checking whether an element is present in a collection like a list, tuple, or dictionary. Python provides a convenient way to do this using the in
keyword, which utilizes the __contains__
method under the hood.
In this article, we will discuss the __contains__
method in Python and discover how it simplifies membership testing.
Understanding Membership Testing
Membership testing is a common task in programming. It involves checking whether a given value exists within a collection (e.g., list, tuple, set, dictionary).
Python makes this operation straightforward and efficient through the in
keyword.
Consider the following code snippet:
my_list = [1, 2, 3, 4, 5]
if 3 in my_list:
print("3 is in the list")
Output:
3 is in the list
In this example, we use the in
keyword to check if the value 3
exists in the list my_list
. If the value is present, the message 3 is in the list
will be printed.
the __contains__
Method in Python
The __contains__
method is defined in Python as follows:
class YourClass:
def __contains__(self, item):
# Custom logic to check if 'item' is present in 'self'
pass
When you use the in
operator to check for the presence of an item in an object of YourClass
, Python will call the __contains__
method with the self
and item
as its arguments.
Essentially, this means that the in
keyword utilizes the __contains__
method to perform membership testing. When you use in
, Python effectively calls the __contains__
method of the collection on which you’re performing the test.
It returns True
if the item is found and False
otherwise.
The __contains__
method is a magic method, denoted by double underscores, that allows you to customize the behavior of membership testing for your classes.
Here’s a simple example of how you can define the __contains__
method in a custom class:
class MyContainer:
def __init__(self, data):
self.data = data
def __contains__(self, item):
return item in self.data
container = MyContainer([1, 2, 3, 4, 5])
if 3 in container:
print("3 is in the custom container")
When you run this code, it will output:
3 is in the custom container
In this code, the MyContainer
class takes a list [1, 2, 3, 4, 5]
as its data attribute. The __contains__
method checks if an item is present in the data
list.
When you use the in
operator with the container
instance and the value 3
, it returns True
, and the message 3 is in the custom container
is printed.
Customize the __contains__
Method in Python
The __contains__
method is a powerful tool for customizing how membership testing works for your objects. You can define the behavior of in
for your classes by implementing this method.
For example, you might want to check for membership based on specific criteria or filters.
Here’s an example of a custom class that implements a unique membership test:
class EvenNumbers:
def __contains__(self, item):
if isinstance(item, int) and item % 2 == 0:
return True
return False
even_numbers = EvenNumbers()
if 6 in even_numbers:
print("6 is an even number")
When you run this code, it will output:
6 is an even number
In this example, the EvenNumbers
class defines a custom membership test that checks if a number is even. When you use the in
operator with an instance of this class and the number 6
, it returns True
because 6
is indeed an even number.
This showcases how you can create specialized membership tests to suit your needs.
Use the __contains__
Method in Strings
Python’s built-in collection types, like lists and sets, are optimized for membership testing using the in
keyword.
However, the __contains__
method is not exclusive to user-defined classes. It is also an integral part of Python’s built-in classes, such as strings.
For example, you can use the __contains__
method in a string to check for the existence of a substring:
str1 = "Delftstack"
str2 = "Del"
val = str1.__contains__(str2)
print(val)
Output:
True
Here, the __contains__
method is employed to check if the substring Del
is present in the string Delftstack
.
Overload the __contains__
Method in Classes
Magic methods, like __contains__
, are invoked internally in classes. You can also use the __contains__
method in a class and overload it to customize its behavior.
In the example below, we overload the __contains__
method within a class to always return False
:
class abc(object):
name = "Delftstack"
def __contains__(self, m):
if self.name:
return False
b = abc()
print("stack" in b)
Output:
False
In the above example, the __contains__
method returns False
if a substring is in a string. When the in
operator is used, the final output is False
, even if the string stack
is present in Delftstack
.
Conclusion
The __contains__
method in Python empowers developers to customize membership testing for their classes and even extends its magic to built-in classes like strings.
Whether you are building custom containers, data structures, or specialized filtering mechanisms, the __contains__
method offers you the ability to tailor membership testing to your specific needs, ultimately enhancing the expressiveness and versatility of Python.
Additionally, this article has shed light on the presence of __contains__
in built-in classes, allowing you to explore its possibilities in various contexts, including strings and other Python objects.