Tkinter Threading
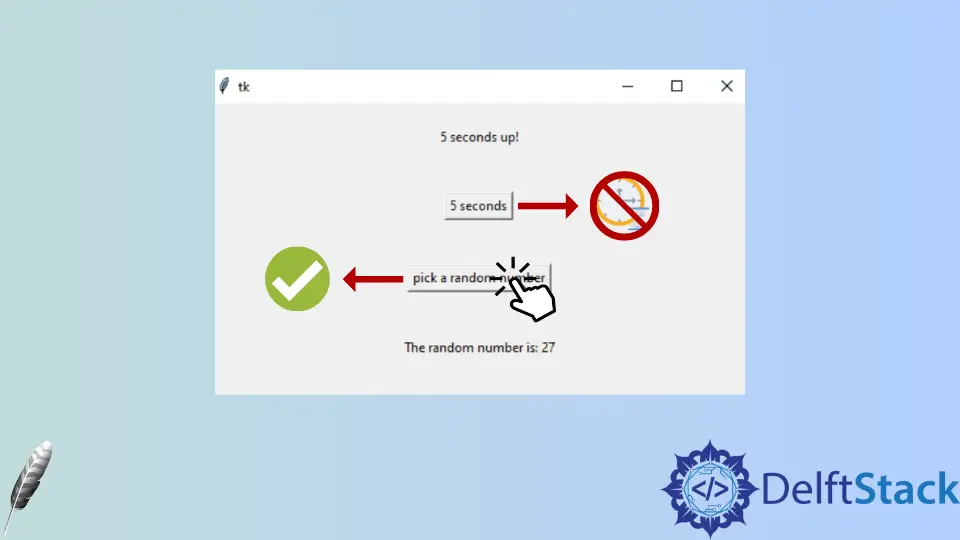
This tutorial shows how to handle single threading and multithreading in Tkinter.
Handle Single Threading in Tkinter
Python provides many options for creating GUI (Graphical User Interface). Of all the GUI modules, Tkinter is the most widely used.
The Tkinter module is the best and easy way to create GUI applications in Python. While creating a GUI, we maybe need to perform multiple tasks or operations in the backend.
Suppose we want to multitask simultaneously; the problem will occur when we assign the next task before executing the last one. Because in the backend, each task executes one by one.
During a single task, the GUI window will also not move, which is why we need threading in Tkinter.
Example of not using threading
:
from tkinter import *
import time
from random import randint
# Initialize a new window
root = Tk()
root.geometry("500x400")
# A function that interrupts for five seconds
def five_seconds():
time.sleep(5)
label.config(text="5 seconds up!")
# A function that generates a random number
def random_numbers():
rand_label.config(text=f"The random number is: {randint(1,100)}")
label = Label(root, text="Hello there!")
label.pack(pady=20)
# A button that calls a function
button1 = Button(root, text="5 seconds", command=five_seconds)
button1.pack(pady=20)
button2 = Button(root, text="pick a random number", command=random_numbers)
button2.pack(pady=20)
rand_label = Label(root, text="")
rand_label.pack(pady=20)
root.mainloop()
As you can see, if we do not use threading
, we have to wait to perform the next task.
This way, the entire program is stuck until the last task execution is completed.
Python has a built-in threading
library to handle this situation.
import threading
from tkinter import *
import time
from random import randint
# Initialize a new window
root = Tk()
root.geometry("500x400")
# A function that interrupts for five seconds
def five_seconds():
time.sleep(5)
label.config(text="5 seconds up!")
# A function that generates a random number
def random_numbers():
rand_label.config(text=f"The random number is: {randint(1,100)}")
label = Label(root, text="Hello there!")
label.pack(pady=20)
# A button that calls a function
button1 = Button(
root, text="5 seconds", command=threading.Thread(target=five_seconds).start()
)
button1.pack(pady=20)
button2 = Button(root, text="pick a random number", command=random_numbers)
button2.pack(pady=20)
rand_label = Label(root, text="")
rand_label.pack(pady=20)
root.mainloop()
See the difference with threading.
button1 = Button(
root, text="5 seconds", command=threading.Thread(target=five_seconds).start()
)
In this line of code, we are using the Thread()
class.
The Thread()
class has a target
option that takes a function as a value. The start()
method helps to start threading.
Handle Multithreading in Tkinter
from threading import *
from tkinter import *
from time import *
# Declare an instance of TK class
win = Tk()
win.geometry("400x400")
# Declare an instance of canvas class
cnv = Canvas(win, height=300, width=300)
cnv.pack()
# This class takes threading as an argument
class FirstOval(Thread):
def run(self):
sleep(1)
for i in range(1, 4):
# Create oval
cnv.create_oval(10 * i, 10 * i, 100, 35 * i, outline="blue", width=2)
sleep(2)
class SecondOval(Thread):
def run(self):
sleep(1)
for i in range(1, 4):
# Create oval
cnv.create_oval(50 * i, 10 * i, 200, 35 * i, outline="red", width=2)
sleep(2)
# Create an object
first_oval = FirstOval()
first_oval.start()
second_oval = SecondOval()
second_oval.start()
win.mainloop()
Now we are working with multithreading. Notice the FirstOval
and SecondOval
classes; they take Thread
as an argument and run simultaneously with the help of the run()
method.
The run()
method executes automatically that the code is wrapped in it.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn