How to Create a Tkinter Table
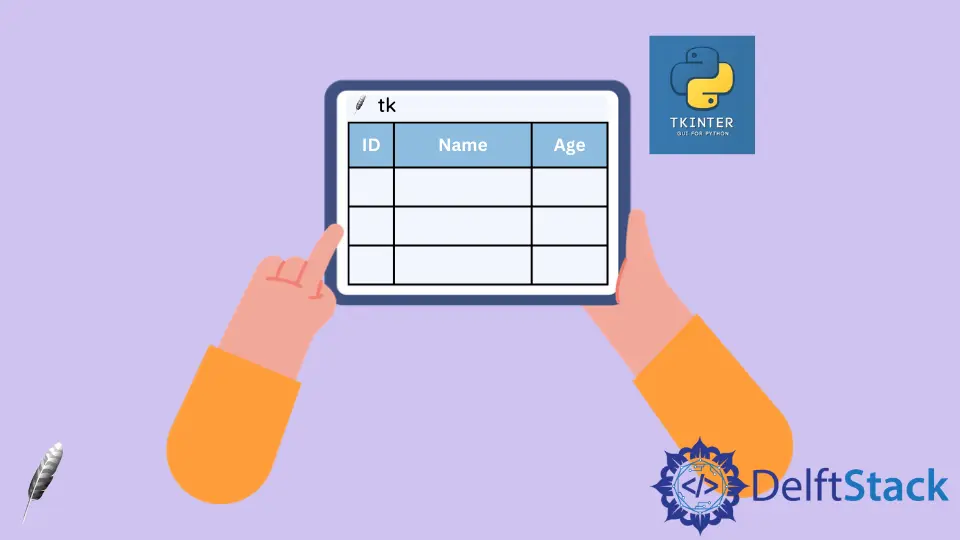
You can use the GUI as standalone or integrated applications in a wider variety of applications.
This tutorial shows how to create a Tkinter table in Python, and also, we will discuss an approach related to the Tkinter table in Python.
Use Entry Widget to Create Table in Tkinter
Tkinter helps create tables, but it does not provide a user interface for the table widget. You can create a table by repeatedly displaying entry widgets in rows and columns.
However, to make a table with six rows and four columns, we will have to use a for
loop (see below).
for i in range(6):
for j in range(4):
We need to create an Entry
widget within these loops by creating an Entry
class object (see below).
entry = Entry(gui, width=22, fg="blue", font=("Arial", 15, "bold"))
Now, we need to set this intersection in the rows and columns. This can be done using a grid()
method, in which we can pass row and column as arguments, such as below.
# here row and column indicate
# row and column positions
entry.grid(row=row, column=column)
Now we can use the insert()
method to insert data into the Entry
widget (see below).
entry.insert(END, data)
The insert()
method takes two parameters, END
and data
. The End
parameter is responsible for adding continuous data at the end of the previous data in the Entry()
widget.
You will create the data
parameter by yourself; it can be a list, Excel sheet or database.
This is a logical approach used in the system below using data from the list. In this code, we take a list containing 6 tuples, and each contains four values holding the Employee id
, Name
, City
and Age
.
So, we will have a table with 6 rows and 4 columns in each row. This program can also be used with an Excel sheet or database to display all data in a table format.
from tkinter import *
# Table class
class Table:
# Initialize a constructor
def __init__(self, gui):
# An approach for creating the table
for i in range(total_rows):
for j in range(total_columns):
print(i)
if i == 0:
self.entry = Entry(
gui,
width=20,
bg="LightSteelBlue",
fg="Black",
font=("Arial", 16, "bold"),
)
else:
self.entry = Entry(gui, width=20, fg="blue", font=("Arial", 16, ""))
self.entry.grid(row=i, column=j)
self.entry.insert(END, employee_list[i][j])
# take the data
employee_list = [
("ID", "Name", "City", "Age"),
(1, "Gorge", "California", 30),
(2, "Maria", "New York", 19),
(3, "Albert", "Berlin", 22),
(4, "Harry", "Chicago", 19),
(5, "Vanessa", "Boston", 31),
(6, "Ali", "Karachi", 30),
]
# find total number of rows and
# columns in list
total_rows = len(employee_list)
total_columns = len(employee_list[0])
# create root window
gui = Tk()
table = Table(gui)
gui.mainloop()
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn