How to Create Progress Bar in Tkinter
- Progress Bar Widget in Tkinter
- Use the Determinate Progress Bar in Tkinter
- Use the Indeterminate Progress Bar in Tkinter
- Use Text in Tkinter Progress Bar
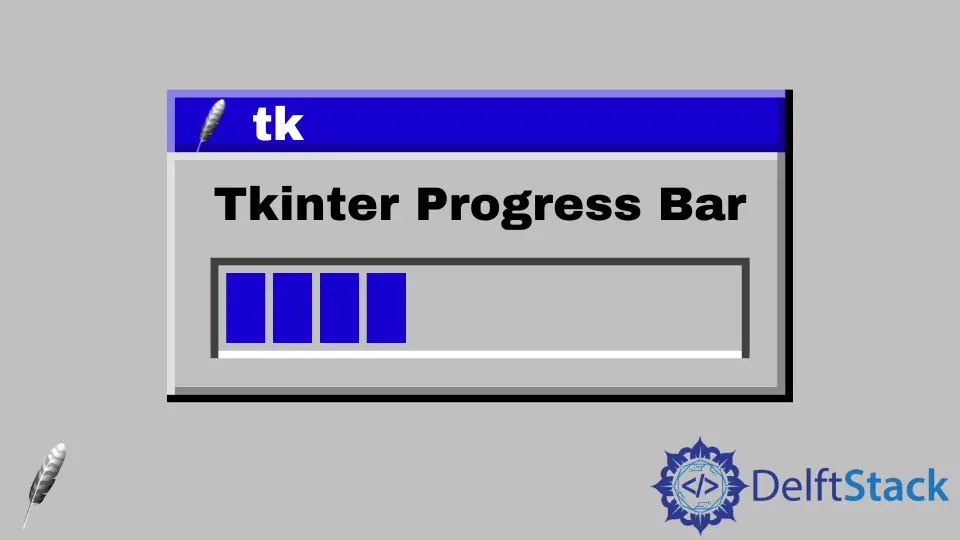
In this demonstration, we will learn how to create a progress bar in Tkinter in multiple ways.
Progress Bar Widget in Tkinter
The progress bar widget analyzes the user task in progress done by the user. We look at this widget in several places, like during installation or other GUI application processes.
This widget can develop in two modes, determinate mode and indeterminate mode. The widget lets you analyze progress when the process is finished in the determinate mode.
This mode shows progress in increasing order.
In the indeterminate mode, we can see animation using this widget to assume something is happening. In this mode, the cursor jumps back and forth between the edges of the widget.
Use the Determinate Progress Bar in Tkinter
from tkinter import *
from tkinter import ttk
gui = Tk()
gui.title("Delftstack")
gui.geometry("600x400")
def progress():
# increment of progress value
progress_var["value"] += 20
# create an object of progress bar
progress_var = ttk.Progressbar(gui, orient=HORIZONTAL, length=400, mode="determinate")
progress_var.pack(pady=30)
btn = Button(gui, text="progress", command=progress)
btn.pack(pady=30)
gui.mainloop()
The progress bar is a widget of the ttk
class. We can use this widget using the Progressbar()
class.
This class takes multiple arguments.
parent
: The root of the GUI window.orient
: This argument specifies the orientation. We can set two values,HORIZONTAL
andVERTICAL
.length
: The length can be adjusted using this attribute.mode
: This attribute accepts two modes,determinate
andindeterminate
.
In this case, we are controlling the progress bar with a button. The progress()
function will be called whenever a button is pressed, and the value will increase by 20 percent.
There are different methods of the Progressbar
class. This code used the start()
and stop()
methods.
The start()
method contains the value and stop()
method contains zero arguments.
from tkinter import *
from tkinter import ttk
gui = Tk()
gui.title("Delftstack")
gui.geometry("600x400")
def StartProgress():
# start progress
progress_var.start(10)
def StopProgress():
# stop progress
progress_var.stop()
# create an object of progress bar
progress_var = ttk.Progressbar(gui, orient=HORIZONTAL, length=400, mode="determinate")
progress_var.pack(pady=30)
btn = Button(gui, text="progress", command=StartProgress)
btn.pack(pady=30)
btn2 = Button(gui, text="stop", command=StopProgress)
btn2.pack(pady=30)
gui.mainloop()
Output:
Use the Indeterminate Progress Bar in Tkinter
from tkinter import *
from tkinter import ttk
gui = Tk()
gui.title("Delftstack")
gui.geometry("600x400")
def StartProgress():
# start progress
progress_var.start(10)
def StopProgress():
# stop progress
progress_var.stop()
# create an object of progress bar
progress_var = ttk.Progressbar(gui, orient=HORIZONTAL, length=400, mode="indeterminate")
progress_var.pack(pady=30)
btn = Button(gui, text="progress", command=StartProgress)
btn.pack(pady=30)
btn2 = Button(gui, text="stop", command=StopProgress)
btn2.pack(pady=30)
gui.mainloop()
This example is similar to the previous example, but its type is different. This progress bar indeterminate
mode shows the status in animated mode and lets you know something is happening there, but you can not determine where the progress is reached.
Use Text in Tkinter Progress Bar
This code demonstrates how to get value using the Label()
widget.
The code follows these steps.
-
Unfortunately, there is no text feature to display the progress of the progress bar, so we created a
stringvar
variable that accepts a string value and sets it in a label. TheLabel()
widget will display the value of the progress bar. -
The
StartProgress()
function will increase the status of the progress bar. In this function, the loop is wrapped, which will iterate 5 times.
In every iteration, the status of the progress bar will increase 20 percent. Theset()
method sets the current status in a string, and theget()
helps get the value ofstringvar
. -
The
update_idletasks()
method will update the status. There is a 1-second delay in every iteration. -
The status will be reset to zero percent when the
StopProgress()
method is called.
from tkinter import *
from tkinter import ttk
import time
gui = Tk()
gui.title("Delftstack")
gui.geometry("600x400")
def StartProgress():
for i in range(5):
progress_var["value"] += 20
stringvar.set(progress_var["value"])
# get the value
label.config(text=stringvar.get() + " %")
# update value
gui.update_idletasks()
time.sleep(1)
def StopProgress():
# stop progress
progress_var.stop()
# set zero percent
stringvar.set("00.0 %")
label.config(text=stringvar.get())
# create an instance of progress bar
progress_var = ttk.Progressbar(gui, orient=HORIZONTAL, length=400, mode="determinate")
progress_var.place(x=30, y=20)
start_btn = Button(gui, text="Next", command=StartProgress)
start_btn.place(x=30, y=50)
# string variable
stringvar = StringVar()
stringvar.set("00.0 %")
cancel_btn = Button(gui, text="Cancel", command=StopProgress)
cancel_btn.place(x=400, y=50)
label = Label(gui, text=stringvar.get(), font=("", 12))
label.place(x=430, y=25)
gui.mainloop()
Output:
Click here to read official Python docs. Another resource here.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn