Tkinter Mainloop
-
Tkinter
mainloop()
in Python -
Tkinter
mainloop()
Blocking in Python -
Tkinter
mainloop()
Non-Blocking in Python
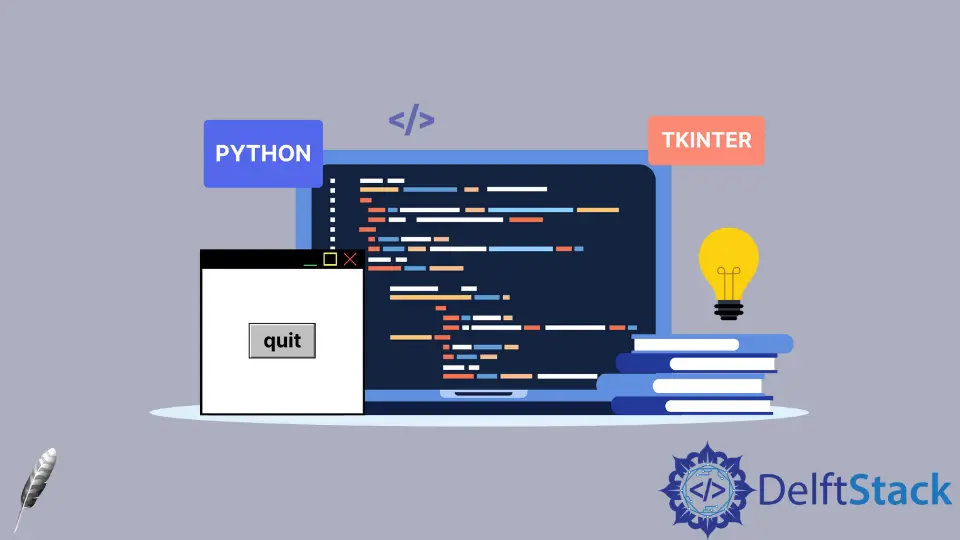
Tkinter is known as a popular module that is written in Python. It is the easiest module in creating a GUI application.
There are many visual elements that we can create and visualize. The mainloop()
method plays the most important role in creating a GUI interface.
This tutorial describes the importance of the mainloop()
method and tries to understand what happens behind this method.
Tkinter mainloop()
in Python
The mainloop()
event is like a while
loop; this will keep running until the user exits. We can say that the mainloop()
works as a driver to update GUI.
Nothing will appear on the window screen if no mainloop()
is used. This approach takes all the creations and has a collaborative response.
The following are a few points we can relate to.
- The GUI window is like a screen that keeps on destroying every microsecond, but the
mainloop
keeps refraining from being closed and appears on the updated screen. - The human eye can’t see the process of destroying the previous screen and recreating a new screen when new activity happens.
- The process execution is fast, so the GUI will not disappear for a microsecond.
The mainloop()
is a method of the TK()
class that helps appear a GUI window. Follow the code that’s written below.
from tkinter import *
# Create a gui or screen
gui = Tk()
gui.title("Delftstack")
# Updates activities
gui.mainloop()
Output:
Tkinter mainloop()
Blocking in Python
There are many reasons why the mainloop()
method keeps blocking the code outside the mainloop
.
- Python executes the code line by line when the
mainloop()
method calls the execution control and waits until the user exits the program. - The
mainloop()
method does not allow Python to the next execution outside themainloop
.
This code can easily differentiate between the command line and the GUI-based application.
# GUI based application
from tkinter import *
gui = Tk()
gui.title("Delftstack")
gui.geometry("400x300")
gui.config(bg="DarkOliveGreen")
quit_btn = Button(gui, text="quit", command=lambda: gui.destroy())
quit_btn.pack(expand=True)
gui.mainloop()
# command line based application
age = input("Enter your age ")
print("your age is :", age)
We can notice when a Python file runs, the GUI application executes, and the command-line application is unable to execute because the command-line application is outside the code; however, the GUI exit using the destroy()
method, then the command-line application execute.
Output:
Tkinter mainloop()
Non-Blocking in Python
We learned how to block the code outside the mainloop
in the previous example.
Now we will demonstrate how to use non-blocking code in the mainloop
.
- The threading is a built-in library in Python. This library is used for the multitasking process, which means the multi-process execute separately.
- The threading is recommended for huge applications, but we can use the
after()
method for threads in a small application.
from tkinter import *
# create an instance of TK
gui = Tk()
gui.title("Delftstack")
gui.geometry("400x300")
gui.config(bg="DarkOliveGreen")
def AskName():
name = input("Please type your name ")
print("your name is: ", name)
quit_btn = Button(gui, text="Quit", command=lambda: gui.destroy())
quit_btn.pack(expand=True)
# call the function after zero second
gui.after(0, AskName)
gui.mainloop()
We realize that the GUI and command-line applications simultaneously execute if we run this code.
Click here to read more about mainloop
.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn