Tkinter Intvar
-
Use
IntVar()
to Create an Integer Variable in Tkinter -
Use
IntVar()
to Calculate Sum of Two Numbers in Tkinter
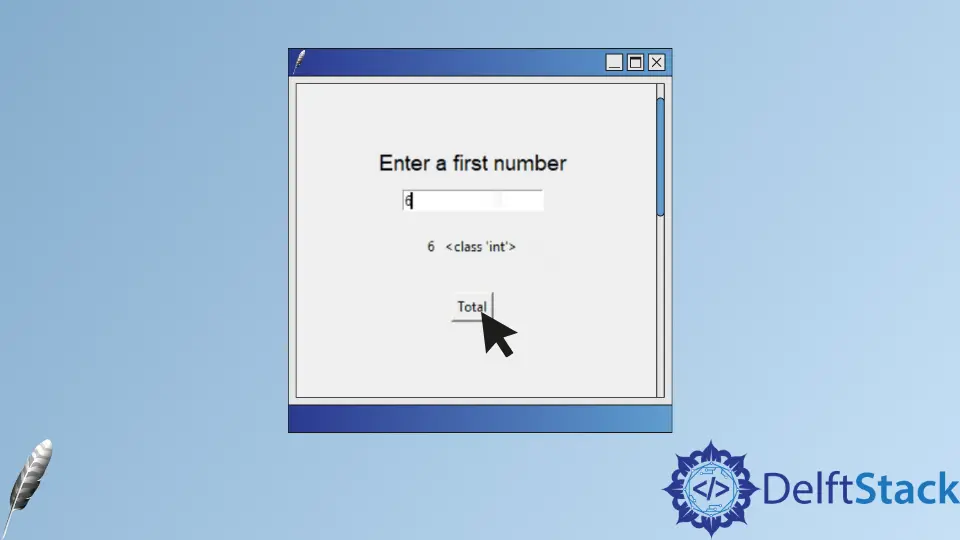
Tkinter contains a variety of built-in programs that act like a standard Python data type with additional features used to trick the widgets of Tkinter like label and entry widget very effectively, making them different from Python data types.
These changes also include getter and setter methods for accessing and changing their values.
This demonstration will look at how the IntVar()
datatype works and how to validate an Entry
widget as an integer in Tkinter.
Use IntVar()
to Create an Integer Variable in Tkinter
How do we determine whether somebody types a numeric or string value into an entry box when dealing with the Entry
widget in Tkinter? Creating an integer variable with the IntVar()
class in Tkinter is pretty simple.
So now we will import tkinter
for being able to use the Tkinter library in our program.
from tkinter import *
After creating an object of Tk
, title
, and geometry
, we need to create an object of the IntVar()
class so it will communicate with accepting variable input in an integer data type.
first_intvar = IntVar()
We will create a button that will call the showDatatype()
function; this function displays the data type of the first_intvar
object and its value. The get()
helps us get an object value from the Entry
widget.
def showDatatype():
second_label.config(text=f"{first_intvar.get()} {type(first_intvar.get())}")
Here is an entire program of this example.
from tkinter import *
# Create GUI window object of TK
GUI_WINDOW = Tk()
GUI_WINDOW.title("Deltstack")
GUI_WINDOW.geometry("400x300")
def showDatatype():
second_label.config(text=f"{first_intvar.get()} {type(first_intvar.get())}")
# Create integer object using IntVar class
first_intvar = IntVar()
first_label = Label(GUI_WINDOW, text="Enter a first number", font=("bold 14"))
first_label.pack()
first_entry = Entry(GUI_WINDOW, textvariable=first_intvar)
first_entry.pack(pady=10)
second_label = Label(GUI_WINDOW, text="")
second_label.pack(pady=10)
total_btn = Button(GUI_WINDOW, text="Total", command=showDatatype)
total_btn.pack(pady=20)
GUI_WINDOW.mainloop()
Output:
As we can look at the output, the IntVar()
data type is not different from the Python built-in data type, so now we will look at another example that helps calculate the sum of two numbers in Tkinter.
Use IntVar()
to Calculate Sum of Two Numbers in Tkinter
Let’s create two objects of IntVar()
for two different Entry
widgets.
first_intvar = IntVar()
second_intvar = IntVar()
After creating two different labels, we need to create another label that will be used in the sumTwoNumbers()
function for displaying our answer.
answer = Label(GUI_WINDOW, text="", font=("itelic 13"))
answer.pack(pady=20)
When clicking a button on the GUI window, sumTwoNumbers()
will be called.
So what happens when we enter something like a string or a character into an entry box?
If you type a word in there or a letter it could not accept as an input by the user, it will throw an error. If we want to figure out whether or not the thing we type into the entry box is a number or not, there are many different ways you can do it, but the best way is to do a try
and except
block.
The try
and except
blocks will restrain the whole program from crashing if we type an invalid input, using the error handling technique. The answer
label is responsible for displaying the sum of two numbers or a raised Exception
; this depends on the input.
def sumTwoNumbers():
try:
# Try to getting integer values and sum two numbers
total = first_intvar.get() + second_intvar.get()
answer.config(text=f"The sum of two numbers is {total}")
except Exception:
answer.config(text="Please enter valid values")
Here is the entire source code of the demo, so copy and run it on your PC.
from tkinter import *
# Create GUI window object of TK
GUI_WINDOW = Tk()
GUI_WINDOW.title("Deltstack")
GUI_WINDOW.geometry("400x300")
def sumTwoNumbers():
try:
# Try to getting integer values and sum two numbers
total = first_intvar.get() + second_intvar.get()
answer.config(text=f"The sum of two numbers is {total}")
except Exception:
answer.config(text="Please enter valid values")
# Create integer object using IntVar class
first_intvar = IntVar()
second_intvar = IntVar()
first_label = Label(GUI_WINDOW, text="Enter a first number", font=("bold 16"))
first_label.pack()
first_entry = Entry(GUI_WINDOW, textvariable=first_intvar)
first_entry.pack(pady=10)
second_label = Label(GUI_WINDOW, text=" Enter a second number", font=("bold 16"))
second_label.pack(pady=10)
second_entry = Entry(GUI_WINDOW, textvariable=second_intvar)
second_entry.pack(pady=10)
total_btn = Button(GUI_WINDOW, text="Total", command=sumTwoNumbers)
total_btn.pack(pady=20)
answer = Label(GUI_WINDOW, text="", font=("itelic 13"))
answer.pack(pady=20)
GUI_WINDOW.mainloop()
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn