How to Draw Line in Tkinter
-
Use
create_line()
Method to Create a Line in the Canvas in Tkinter - Draw the Line Without Canvas in Tkinter
- Draw the Line in the Tkinter Grid
- Draw the Line on the Image in Tkinter
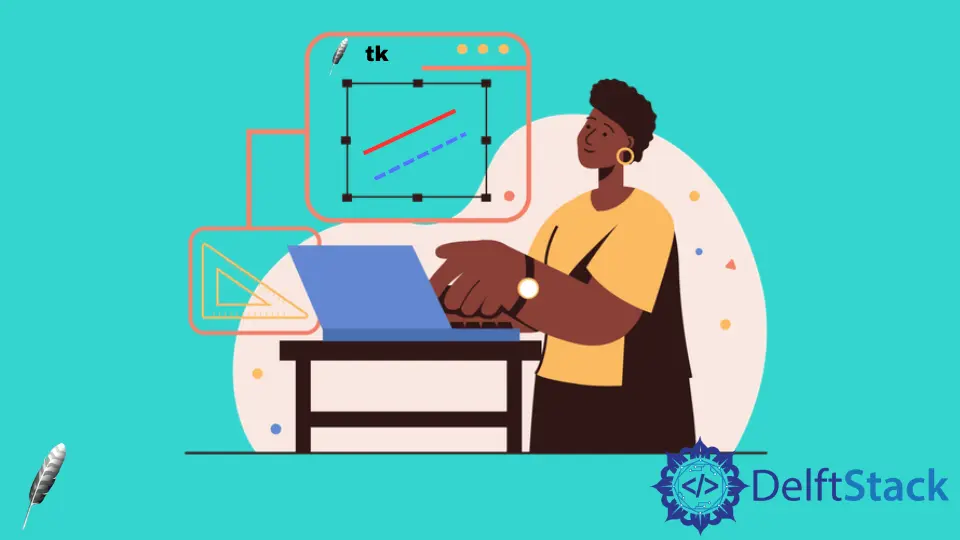
The Tkinter Canvas widget can be used for many purposes, such as drawing shapes and objects or creating graphics and images. We can use it for drawing several widgets: lines, arc bitmap, images, rectangles, text, ovals, polygons, rectangles, and more.
This demonstration will explore how to draw the different types of lines in the Tkinter once we initialize the Tkinter and canvas class.
Use create_line()
Method to Create a Line in the Canvas in Tkinter
We can draw two types of lines; these are simple and dashed. There is an option to specify a dashed line using the dash
property.
The create_line()
takes coordinates and orientation as an argument like 'x1,y1,x2,y2'
.
from tkinter import *
# Initialize an instance of tkinter window
root = Tk()
# Set the width and height of the tkinter window
root.geometry("600x300")
# Create a canvas widget
canvas = Canvas(root, width=500, height=300)
canvas.pack()
# Create a line in canvas widget
canvas.create_line(10, 25, 200, 200, width=5)
# Create a dashed line
canvas.create_line(210, 25, 410, 200, fill="red", dash=(10, 5), width=2)
root.mainloop()
The fill
attribute is also optional. If we do not set the color, the line will be filled with black color by default.
We used the dash
property, which takes two arguments: width and height.
You can see the output window with two lines.
Draw the Line Without Canvas in Tkinter
This is the fact that there is no standard purpose for drawing the line on a Tkinter other than canvas.
If all you need is a straight line as a separator, you can use a frame with a pixel width.
from tkinter import *
# # Initialize an instance of tkinter window
root = Tk()
# Create a vertical Frame
vertical = Frame(root, bg="red", height=50, width=1)
# Create a horizontal Frame
horizontal = Frame(root, bg="blue", height=1, width=70)
vertical.place(x=10, y=10)
horizontal.place(x=50, y=30)
root.mainloop()
The place()
method is the position holder that takes two arguments (starting, ending) points.
Draw the Line in the Tkinter Grid
Now we will see how it could be possible to create a line in the grid.
Once we initialize the Separator()
class and set it in the grid using the grid
widget, the Separator
takes two arguments: window and orient.
The orient
argument takes one value, vertical/horizontal.
from tkinter import *
from tkinter.ttk import Separator, Style
root = Tk()
# Create the left frame
left_frame = Frame(root, bg="blue", width=100, height=100)
left_frame.pack_propagate(False)
left_label = Label(
left_frame, text="Left_frame", fg="white", bg="blue", anchor="center"
)
left_label.pack()
# Set frame in the grid
left_frame.grid(column=2, row=1, pady=5, padx=10, sticky="n")
sp = Separator(root, orient="vertical")
sp.grid(column=3, row=1, sticky="ns")
# edit: To change the color of the separator, you need to use a style
style = Style(root)
style.configure("TSeparator", background="red")
right_frame = Frame(root, bg="blue", width=100, height=100)
right_frame.pack_propagate(False)
right_label = Label(right_frame, text="Right_frame", fg="white", bg="blue")
right_label.pack()
right_frame.grid(column=4, row=1, pady=5, padx=10, sticky="n")
root.mainloop()
The Style()
class helps for styling the whole window.
The pack_propagate()
widget takes Boolean argument. This widget will extend the frame corresponding to the content.
# left_frame.pack_propagate(False)
left_frame.pack_propagate()
# right_frame.pack_propagate(False)
right_frame.pack_propagate()
You can compare the previous and current output. The False
argument helps prevent, and by default, the argument is True
.
pack()
widget will produce an error when using it along with the grid()
widget.Draw the Line on the Image in Tkinter
Sometimes we need to draw the line on the image, the canvas has a create_image()
method that draws an image on the canvas, and we can draw the line using create_line()
as we discussed above.
from tkinter import *
from PIL import Image, ImageTk
root = Tk()
canvas = Canvas(root)
bg_image = Image.open("stop.png")
bg_image = bg_image.resize((300, 205), Image.ANTIALIAS)
bg_image = ImageTk.PhotoImage(bg_image)
canvas.pack(fill=BOTH, expand=1)
bg_img = canvas.create_image(0, 0, anchor=NW, image=bg_image)
line = canvas.create_line(20, 10, 150, 60, fill="red", width=5)
root.title("stop")
root.mainloop()
You can see the red line in the image.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn