Tkinter SimpleDialog Module
-
Use the
askinteger()
Method to Get Integer Value From User in Tkinter -
Use the
askfloat()
Method to Get Float Value From User in Tkinter -
Use the
askstring()
Method to Get String Value From User in Tkinter
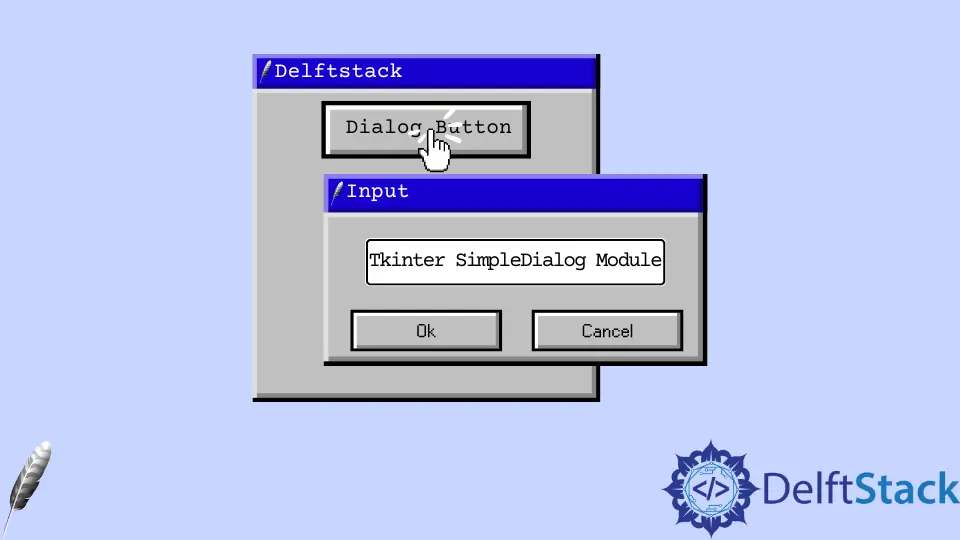
In this tutorial, we’ll learn how to create a simpledialog
box in Tkinter, and then we will look at how to get multiple values from the user with different methods of the simpledialog
box.
The simpledialog
box is a class from the Tkinter library in Python that creates dialog boxes to get user input in various ways. The simpledialog
are the little pop-up dialog boxes that respond from the user, allowing us to take various data from the user, such as integer, float, and string value.
Mostly we need to create an object of TK()
to operate different widgets. If the Tkinter window does not exist, these functions will create a pop-up window automatically.
Use the askinteger()
Method to Get Integer Value From User in Tkinter
This section will look at the askinteger()
method that allows the user to input an integer in the text field that will appear in the pop-up window. This method returns an integer.
We need to import a simpledialog
class that allows us to use its methods.
from tkinter import simpledialog
We’ll create a Button
widget that calls the intdialogbox
function using the command parameter.
dialog_btn = Button(window, text="dialog button", command=intdialogbox)
dialog_btn.pack()
The askinteger()
method takes multiple parameters. The first parameter accepts input as a value, and the second parameter is a text message that will be appeared on the dialog box.
The third parameter is the parent
(this parameter is optional), which takes the root window created by an object of Tk()
. In the following example, the Label
widget stores the output of the dialog box in the dialog_output
variable.
Code:
from tkinter import *
from tkinter import simpledialog
# Create an object of TK (tkinter window)
window = Tk()
window.geometry("200x200")
window.title("Deltstack")
def intdialogbox():
# this method accepts integer and returns integer
employee_age = simpledialog.askinteger("Input", "Enter your age", parent=window)
dialog_output = Label(
window, text=f"Employee age is {employee_age}", font=("italic 12")
)
dialog_output.pack(pady=20)
dialog_btn = Button(window, text="dialog button", command=intdialogbox)
dialog_btn.pack()
window.mainloop()
Output:
Use the askfloat()
Method to Get Float Value From User in Tkinter
We can use the askfloat()
method for a float input which is a number with decimals. This method returns a float value.
This method works the same as the askinteger()
method but differs in the returned value. We’ll discuss the parameters called minvalue
and maxmavlue
.
The minvalue
and maxmavlue
decide a range of value where the user will be restricted in a limited range, and it is a kind of validation.
Code:
from tkinter import *
from tkinter import simpledialog
# Create an object of TK (tkinter window)
window = Tk()
window.geometry("300x200")
window.title("Deltstack")
def floatdialogbox():
# this method accepts float and returns float
income_tax = simpledialog.askfloat(
"Input", "Enter the tax", parent=window, minvalue=1.0, maxvalue=10.0
)
dialog_output = Label(
window, text=f"Your tax is {income_tax} percent", font=("italic 12")
)
dialog_output.pack(pady=20)
dialog_btn = Button(window, text="dialog button", command=floatdialogbox)
dialog_btn.pack()
window.mainloop()
Output:
Use the askstring()
Method to Get String Value From User in Tkinter
The askstring()
method helps the user input a string value in the dialog box and returns a string as an output. The dialog box takes a name in the code below and displays it in a label.
Code:
from tkinter import *
from tkinter import simpledialog
# Create an object of TK (tkinter window)
window = Tk()
window.geometry("300x200")
window.title("Deltstack")
def stringdialogbox():
# this method accepts float and returns float
Employee_name = simpledialog.askstring("Input", "Enter your name", parent=window)
dialog_output = Label(
window, text=f"The employee name is {Employee_name}", font=("italic 12")
)
dialog_output.pack(pady=20)
dialog_btn = Button(window, text="dialog button", command=stringdialogbox)
dialog_btn.pack()
window.mainloop()
Output:
Click here to read more about the simpledialog
box.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn