Tkinter Create_rectangle Method
-
Use the
create_rectangle()
Method to Create Rectangle in Tkinter - Create a Rectangle With Text in Tkinter
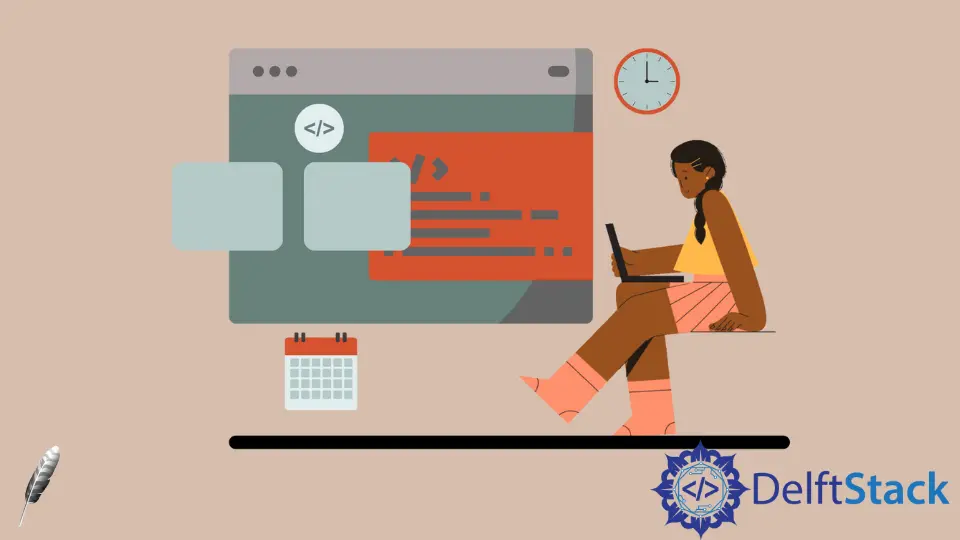
In this discussion, we will introduce the TK
canvas widget and what it is used for, how you can place it onto a GUI window and how to create a rectangle on canvas using the create_rectangle()
method in Tkinter.
It is the simplest method the canvas widget allows for drawing shapes upon itself, and it is useful for drawing graphs. It allows for the plotting of values, and ultimately it can be used to implement custom widgets that you as a programmer can develop from scratch.
Use the create_rectangle()
Method to Create Rectangle in Tkinter
As we know that the module used to make the GUI within Python is the Tkinter module, so first, we need to import our Tkinter module; for all sorts of functions and methods, we need to write the following code.
from tkinter import *
Now we will declare one variable called window
equal to the Tk()
class.
For our termination, the statement must be a window.mainloop()
; we are ready to run it out. We will see a simple GUI created on our screen when we run it.
window = Tk()
window.mainloop()
We have a class called the Canvas
, a subclass of Tk
; this is not just a class. It is like a graphical tool that allows us to create certain figures or write certain text on the GUI window.
The Canvas
accepts some parameters: parent
, width
, and height
; a parent window object, in our case, is a window
variable. So basically, this line of code will create an inner window into the parent window.
# create an inner window
cv = Canvas(window, width=500, height=500)
cv.pack()
Now we will create a rectangle onto the canvas using the create_rectangle()
method of the Canvas
class.
This method will need some parameters to create a rectangle. There are four required parameters to make it executable: x0
, y0
, x1
, and y1
.
The x0 y0
parameter is like the upper right corner, and the x1 y1
is like the lower right corner.
cv.create_rectangle(50, 20, 200, 200, fill="red")
So this way, we can create a rectangle in our Tkinter GUI window.
from tkinter import *
# create a GUI window widget
window = Tk()
# create an inner window
cv = Canvas(window, width=500, height=500)
cv.pack()
# create a rectangle
cv.create_rectangle(50, 20, 200, 200, fill="red")
window.mainloop()
Output:
Create a Rectangle With Text in Tkinter
We change a few things to create text into a rectangle in this section. So we have created four with different orientations.
# create a red rectangle
cv.create_rectangle(0, 0, 200, 200, fill="red")
# create a blue rectangle
cv.create_rectangle(205, 0, 405, 200, fill="blue")
# create a yellow rectangle
cv.create_rectangle(0, 205, 200, 405, fill="yellow")
# create a green rectangle
cv.create_rectangle(205, 205, 405, 405, fill="green")
We are now familiar with creating rectangles, as we have looked at in the previous example, so we talk about the create_text()
method.
This method accepts two coordinates point to place the text, and we are already familiar with the text
and fill
parameters.
cv.create_text(100, 100, text="Red Rectangle", fill="white")
Here is our entire code of this example, so copy and run it on your PC.
from tkinter import *
# create a GUI window widget
window = Tk()
# create an inner window
cv = Canvas(window, width=500, height=500)
cv.pack()
# create a red rectangle
cv.create_rectangle(0, 0, 200, 200, fill="red")
# This method create a text in rectangle
cv.create_text(100, 100, text="Red Rectangle", fill="white")
# create a blue rectangle
cv.create_rectangle(205, 0, 405, 200, fill="blue")
cv.create_text(300, 100, text="Blue Rectangle", fill="white")
# create a yellow rectangle
cv.create_rectangle(0, 205, 200, 405, fill="yellow")
cv.create_text(100, 300, text="Yellow Rectangle")
# create a green rectangle
cv.create_rectangle(205, 205, 405, 405, fill="green")
cv.create_text(300, 300, text="Green Rectangle")
window.mainloop()
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn