Tkinter askopenfilename
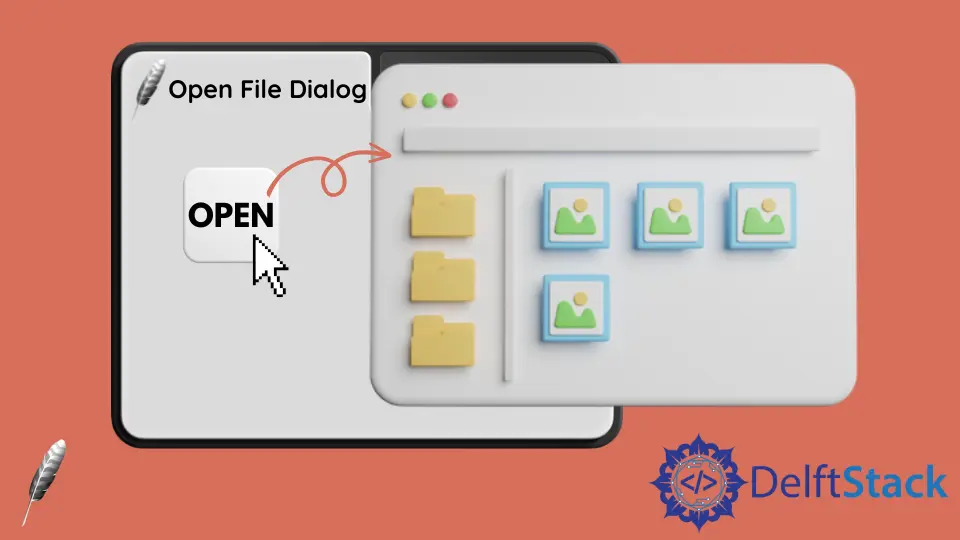
This article will demonstrate how to use the file dialog box to open files using the askopenfilename()
method from the filedialog
class. No matter where the file is on your computer.
When we deal with real-world GUI applications and would like to add a file dialog feature that will open a file (an image, a PDF file or anything at all), how do you do that with Tkinter? It is really easy to use something called a filedialog
class.
Use the askopenfilename()
Method to Open the File Dialog Box in Tkinter
The askopenfilename()
is responsible for opening and reading files in the Tkinter GUI application; this method exists in the filedialog
class.
So first, we will need to import this class, such as below.
from tkinter import filedialog
After creating an instance of the Tk()
class, we need to add a button in our GUI, so when we click on this button, it will launch a file dialog box, and we can select our file.
open_btn = Button(text="Open", command=openFile)
open_btn.pack()
We have created an openFile()
function that helps open a file dialog box. We are using a method from the filedialog
class.
def openFile():
# takes file location and its type
file_location = filedialog.askopenfilename(
initialdir=r"F:\python\pythonProject\files",
title="Dialog box",
filetypes=(("text files", "*.txt"), ("all files", "*.*")),
)
# read selected file by dialog box
file = open(file_location, "r")
print(file.read())
file.close()
Inside this method, we got to pass a bunch of different options.
initialdir
: This option tells the dialog box what directory to start in, so the box pops up what directory you want to be showing, so you should go with this option.title
: This is just the box’s title that pops up. It will have a little caption title at the top, and you could put anything you want.filetypes
: This option helps choose the type of files to show. You could just put all the files, specifically the.txt
file or other file extensions.
The askopenfilename()
method returns a string, and that string is the file path of where your file is located, so we stored a file path in a variable called file_location
.
Now we will open and read the content of this file to create a file variable, and we have used the open
function that takes a file path and mode.
We used "r"
mode to open a file in reading mode. The read()
method helps read the entire content and displays it in the console.
The complete source code is here.
from tkinter import *
from tkinter import filedialog
def openFile():
# takes file location and its type
file_location = filedialog.askopenfilename(
initialdir=r"F:\python\pythonProject\files",
title="Dialog box",
filetypes=(("text files", "*.txt"), ("all files", "*.*")),
)
# read selected file by dialog box
file = open(file_location, "r")
print(file.read())
file.close()
gui_win = Tk()
gui_win.geometry("200x200")
gui_win.title("Delftstack")
open_btn = Button(text="Open", command=openFile)
open_btn.pack()
gui_win.mainloop()
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn