Tkinter Dialog Box
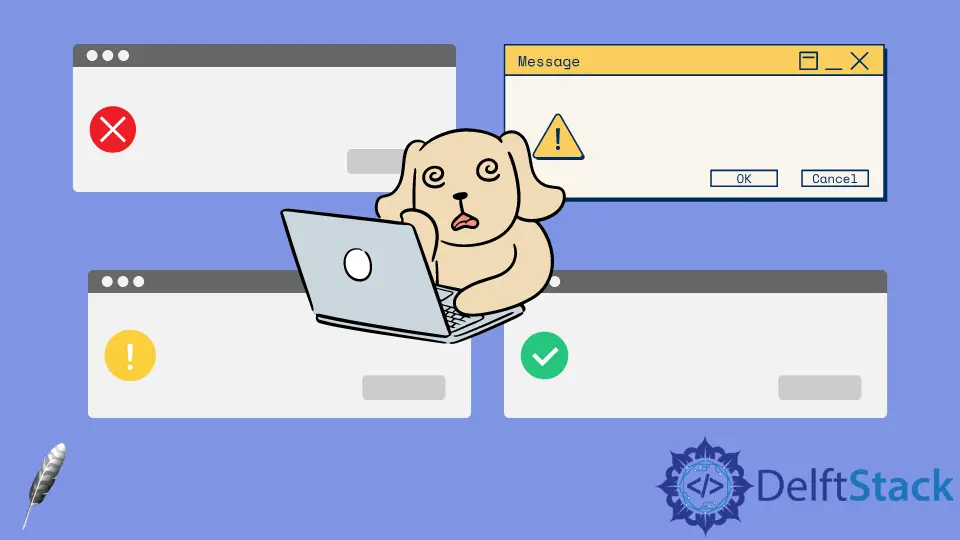
We will introduce the popup dialog boxes and see how to create different types of dialog boxes for different purposes.
In Python, graphical user interface application development popup dialogue boxes are small size Windows that you can use to display a message box, error message box, warning messages, question message box, etc.
We can generate the popup dialogue box using the messagebox
class from the tkinter
module.
Create Different Dialog Boxes With messagebox
in Tkinter
To start, we’ll import these two stated in the code below.
from tkinter import *
from tkinter import messagebox
You have to set up a window to start working with the GUI window whenever you want to do anything in Tkinter. The below line of code puts our window in the center of the screen on the top level of everything.
GUI.eval("tk::PlaceWindow %s center" % GUI.winfo_toplevel())
The following code line specifies that it will not be hidden behind another window once we open it. It will make sure that it is as in front.
We withdraw our window, and this will make our window invisible. Without using this code, you’ll be able to see another window behind the popup window.
GUI.withdraw()
The next line uses the showinfo()
method that accepts two string parameters. The first is a title
, and the second is a message
that you want to display in this dialog box.
messagebox.showinfo("information", "You have created successfully the showinfo box")
Finally, we make sure in the last code lines to quit our window once we’re finished, so it no longer shows on the screen. Here’s the complete example code of the showinfo
dialog box.
Code:
from tkinter import *
from tkinter import messagebox
GUI = Tk()
GUI.eval("tk::PlaceWindow %s center" % GUI.winfo_toplevel())
GUI.withdraw()
messagebox.showinfo("information", "You have created successfully the showinfo box")
GUI.deiconify()
GUI.destroy()
GUI.quit()
Output:
If you wish to show an error dialog box, you will type the showerror()
message box.
Code:
from tkinter import *
from tkinter import messagebox
GUI = Tk()
GUI.eval("tk::PlaceWindow %s center" % GUI.winfo_toplevel())
GUI.withdraw()
# Displays an error dialog box
messagebox.showerror("Error", "You have generated an error successfully")
GUI.deiconify()
GUI.destroy()
GUI.quit()
Output:
The other is a warning dialog box like where you go with the warning sign. Use the showwarning()
message box.
Code:
from tkinter import *
from tkinter import messagebox
GUI = Tk()
GUI.eval("tk::PlaceWindow %s center" % GUI.winfo_toplevel())
GUI.withdraw()
# Displays a warning dialog box
messagebox.showwarning("Warning", "This is warning message by tkinter")
GUI.deiconify()
GUI.destroy()
GUI.quit()
Output:
We’ll use the askyesno()
message box in the following dialog box to have the buttons yes/no on the popup window.
Code:
from tkinter import *
from tkinter import messagebox
GUI = Tk()
GUI.eval("tk::PlaceWindow %s center" % GUI.winfo_toplevel())
GUI.withdraw()
# Displays yes/no dialog box
messagebox.askyesno("Asking", "Do you love delftstack?")
GUI.deiconify()
GUI.destroy()
GUI.quit()
Output:
The askokcancel()
dialog box helps us make two decisions, either "ok"
for "cancel"
. In this example, we make a few changes with two conditions.
Since the dialog box returns a True
or False
value by user action, we can set the condition onto the dialog box.
Code:
from tkinter import *
from tkinter import messagebox
GUI = Tk()
GUI.eval("tk::PlaceWindow %s center" % GUI.winfo_toplevel())
GUI.withdraw()
# Displays askokcancel dialog box
if messagebox.askokcancel("askokcancel", "Update your tkinter version") == True:
print("ok")
else:
print("cancel")
GUI.deiconify()
GUI.destroy()
GUI.quit()
Output:
The askretrycancel()
dialog box asks us to retry the procedure. The icon
parameter helps us to set any icon.
In our case, we just set an error icon.
Code:
from tkinter import *
from tkinter import messagebox
GUI = Tk()
GUI.eval("tk::PlaceWindow %s center" % GUI.winfo_toplevel())
GUI.withdraw()
# Displays askretrycancel dialog box
messagebox.askretrycancel("Retry", "Invalid pin please Retry", icon="error")
GUI.deiconify()
GUI.destroy()
GUI.quit()
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn