Tkinter askdirectory() Method
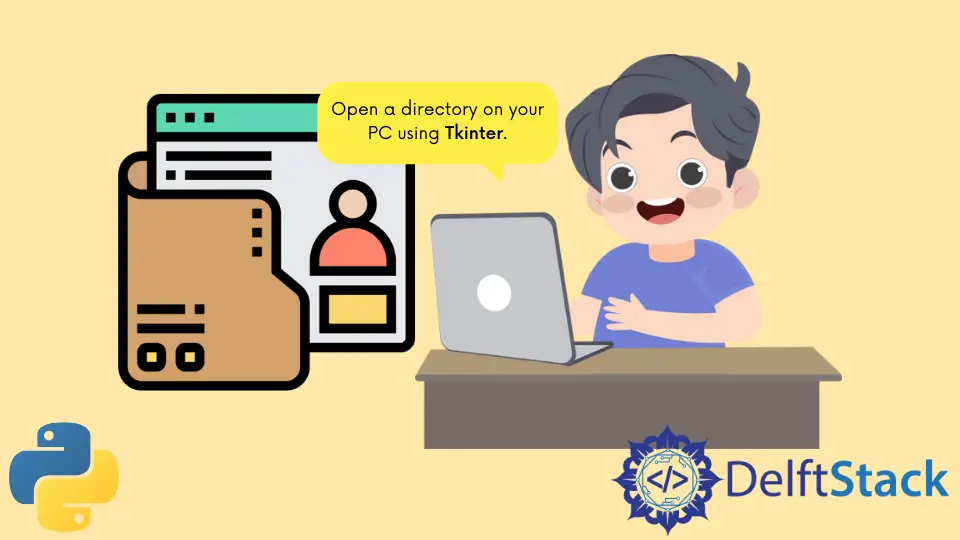
This tutorial will discuss graphical user interfaces with Python, specifically using tkinter
. Then we will look at how to work the askdirectory()
method to open a directory on your pc.
Tkinter
in Python
Tkinter
is the de facto standard GUI (Graphical User Interface) toolkit for Python, providing a robust and user-friendly platform for building desktop applications. With its simplicity and ease of use, Tkinter
is an ideal choice for both beginners and experienced developers.
The library comes bundled with Python, requiring no additional installations, making it readily available for developers across various platforms. Tkinter's
versatility extends to its vast array of widgets, allowing developers to create interactive interfaces effortlessly.
Its seamless integration with Python and widespread adoption make Tkinter
an indispensable tool for crafting graphical applications with efficiency and clarity.
Special GUI elements also allow users to browse the file system and select a file path. The user can either open up a file at a specific file path or save a file at a specific file path.
Use the askdirectory()
Method to Open File Dialog in Tkinter
The askdirectory()
method in Tkinter's
filedialog
module allows you to prompt the user to select a directory. Its syntax includes optional parameters that customize the appearance and behavior of the directory selection dialog.
The syntax for askdirectory()
is as follows:
filedialog.askdirectory(**options)
When using the askdirectory()
method in Tkinter's
filedialog
module, you can customize its behavior by providing optional keyword arguments, commonly referred to as options. These options allow you to tailor the directory selection dialog according to your application’s requirements, providing a more customized and user-friendly experience.
Depending on the project, the developer could choose to add options to customize the dialog box or not.
In this article, we’ll delve into the usage of Tkinter's
askdirectory()
method, providing a comprehensive guide with a practical example. Understanding how to integrate this method into your Python applications can greatly enhance the user experience when dealing with file-related tasks.
Case 1: Non-customized Dialog Box
In this example, a minimal structure is used. It creates a Tkinter
root window, hides it (root.withdraw()
), uses filedialog.askdirectory()
to get the selected directory, and then prints it.
This is a straightforward way to use askdirectory()
without customizing the appearance or behavior of the dialog box.
Example:
import tkinter as tk
from tkinter import filedialog
# Create the main window
root = tk.Tk()
root.withdraw() # Hide the main window
# Use askdirectory() to get the selected directory
selected_directory = filedialog.askdirectory()
# Print the selected directory
print("Selected Directory:", selected_directory)
# Main loop to keep the window open (optional)
root.mainloop()
In the code example provided, we begin by importing the necessary modules: tkinter
, the standard GUI package, and filedialog
, which includes classes and functions for creating file and directory dialogs.
Next, we create the main Tkinter
window using tk.Tk()
. The withdraw()
method is employed to temporarily hide the window, allowing us to focus on displaying the file dialog exclusively.
The heart of the example lies in the askdirectory()
method, which opens a dialog box, enabling the user to select a directory interactively. The chosen directory is then stored in the variable selected_directory
.
Following the directory selection, the code prints the selected directory to the console. In a practical application, this information could be utilized for further processing or displayed to the user.
Lastly, the mainloop()
function is essential for maintaining the open state of the main window. While seemingly redundant in this uncomplicated example, it becomes crucial for ensuring responsiveness in more intricate GUI applications.
Output:
Selected Directory: C:/Users/Jamie Beatrice/Downloads/WorkCodes
Case 2: Customized Dialog Box
In the more advanced example, we explore customization options for the askdirectory()
method by utilizing a dictionary named options
.
This example demonstrates more advanced usage by providing options
to customize the appearance and behavior of the dialog box. The choice between them depends on your specific requirements for the directory selection dialog.
You can customize the dialog box further by providing options. Here’s an example with some common options:
import tkinter as tk
from tkinter import filedialog
root = tk.Tk()
root.withdraw()
options = {
"initialdir": "/Downloads",
"title": "Select Directory",
"mustexist": True,
"parent": root,
}
selected_directory = filedialog.askdirectory(**options)
print("Selected Directory:", selected_directory)
root.mainloop()
The options
dictionary is then passed as keyword arguments to askdirectory(**options)
, enabling users to tailor the appearance and behavior of the dialog box according to their needs.
Various options can be included in the dictionary to modify the dialog’s behavior. For instance, initialdir
specifies the initial directory when the dialog opens, title
sets the title of the dialog box, mustexist
restricts the selection to existing directories if set to True
, and parent
designates the parent window for the dialog.
This flexible approach allows developers to fine-tune the file selection dialog for a more personalized user experience. You can customize it based on your specific requirements.
Output:
Selected Directory: C:/Users/Jamie Beatrice/Downloads
Case 3: Display List of Files in a Directory
Displaying a list of files in a directory using Tkinter's
askDirectory()
enhances user interaction by providing a visual overview of available files. This feature promotes user confidence and efficiency, allowing them to make informed decisions when selecting a directory.
With a clear representation of the directory’s contents, users can easily navigate and choose the precise location, streamlining file-related tasks within the application.
WorkCodes Folder
: (List of Files)
TKINTER.py
BUTTON.java
Scanz.java
BytetoInt.java
fontz.java
The provided code creates a simple Tkinter
application that allows users to select a directory using a file dialog and then displays the list of files in that directory using a Treeview
widget.
import os
import tkinter as tk
import tkinter.ttk as ttk
from tkinter import filedialog
gui_win = tk.Tk()
gui_win.geometry("400x200")
gui_win.grid_rowconfigure(0, weight=1)
gui_win.grid_columnconfigure(0, weight=1)
def browse_directory():
# get a directory path by user
folder_path = filedialog.askdirectory(
initialdir=r"F:\python\pythonProject", title="Dialog box"
)
if folder_path:
current_dir.set(folder_path)
list_files(folder_path)
label_path = tk.Label(gui_win, text=folder_path, font=("italic 14"))
label_path.pack(pady=20)
def list_files(folder_path):
for filename in os.listdir(folder_path):
if os.path.isfile(os.path.join(folder_path, filename)):
tree_view.insert("", "end", values=(filename,))
current_dir = tk.StringVar()
dialog_btn = tk.Button(gui_win, text="select directory", command=browse_directory)
dialog_btn.pack(pady=10)
folder_label = tk.Label(gui_win, textvariable=current_dir)
folder_label.pack()
tree_view = ttk.Treeview(
gui_win, columns=("Files",), show="headings", selectmode="browse"
)
tree_view.heading("Files", text="Files in Directory")
tree_view.pack(padx=20, pady=20, fill="both", expand=True)
gui_win.mainloop()
The tk.Tk()
creates the main Tkinter
window, and its geometry is set to 400x200 pixels. The window is configured to allow resizing with the grid_rowconfigure
and grid_columnconfigure
methods.
A function browse_directory()
is defined, which is triggered when the select directory
button (dialog_btn
) is pressed. Inside this function, filedialog.askdirectory()
prompts the user to choose a directory, and the selected directory path is stored in the variable folder_path
.
If a directory is selected (if folder_path:
), the current_dir
variable is updated with the selected path. The list_files()
function is then called to populate the Treeview
widget with the list of files in the selected directory.
A label (label_path
) is created to display the selected directory path, and it is packed into the window.
The Treeview
widget (tree_view
) is configured with a single column (Files
) to display the list of files. The heading
method sets the column heading, and the widget is packed into the window.
The main loop (gui_win.mainloop()
) is executed to keep the Tkinter
window open.
When the select directory
button is pressed, the file dialog appears, allowing the user to choose a directory. Once selected, the directory path is displayed, and the Treeview
widget is populated with the list of files in that directory.
Output:
C:/Users/Jamie Beatrice/Downloads/WorkCodes
BUTTON.java
BytetoInt.java
fontz.java
Scanz.java
TKINTER.py
C:/Users/Jamie Beatice/Downloads/WorkCodes
This code provides a user-friendly way to navigate directories, select a folder, and visualize its contents using a Tkinter
GUI.
Conclusion
By using askDirectory()
will enable users to interactively choose a directory through a user-friendly dialog. This functionality is invaluable for applications that involve file operations, as it streamlines the process and enhances the overall user experience.
Incorporating Tkinter's
askdirectory()
method into your Python applications empowers you to create more dynamic and user-friendly interfaces, making your software more intuitive and accessible.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn