How to Find Quantiles in Pandas
- What Are Quantiles?
-
Using the
quantile()
Method - Calculating Multiple Quantiles at Once
- Customizing Quantile Calculation with Interpolation
- Visualizing Quantiles with Box Plots
- Conclusion
- FAQ
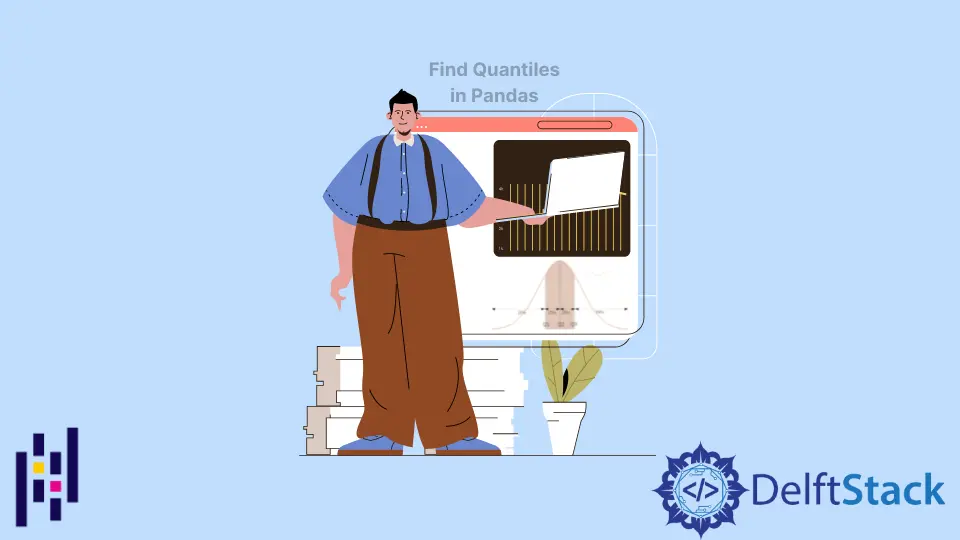
Understanding data is crucial in today’s world, and quantiles are an essential statistical tool that helps us summarize and interpret datasets.
In this tutorial, we will delve into how to find quantiles using the Pandas library in Python. Whether you’re a data analyst, a statistician, or just someone looking to enhance their data manipulation skills, this guide will walk you through the process step-by-step. We’ll explore various methods to calculate quantiles, providing clear examples and explanations along the way. By the end of this article, you’ll be equipped with the knowledge to efficiently extract quantiles from your datasets using Pandas.
What Are Quantiles?
Before jumping into how to find quantiles using Pandas, let’s clarify what quantiles are. Quantiles are values that divide a dataset into equal-sized intervals. For instance, the median is a type of quantile that divides the dataset into two equal halves. Other common quantiles include quartiles (which divide the data into four parts) and percentiles (which divide the data into 100 parts). These statistical measures help in understanding the distribution of data and identifying outliers.
Using the quantile()
Method
One of the most straightforward ways to find quantiles in Pandas is by using the quantile()
method. This method allows you to specify the quantile you want to calculate, expressed as a decimal. For example, to find the median (0.5 quantile), you would use 0.5 as the argument.
Here is a simple example:
import pandas as pd
data = {'values': [10, 20, 30, 40, 50]}
df = pd.DataFrame(data)
median = df['values'].quantile(0.5)
print(median)
Output:
30.0
In this example, we created a DataFrame containing a single column named ‘values’. By calling the quantile()
method on this column and passing 0.5, we retrieved the median value of the dataset, which is 30. Understanding how to use this method opens up a world of possibilities for analyzing your data.
Calculating Multiple Quantiles at Once
Sometimes, you may want to calculate multiple quantiles simultaneously. Pandas makes this easy with the quantile()
method as well. You can pass a list of quantiles to the method, and it will return the corresponding values for each quantile.
Here’s how you can do it:
import pandas as pd
data = {'values': [10, 20, 30, 40, 50]}
df = pd.DataFrame(data)
quantiles = df['values'].quantile([0.25, 0.5, 0.75])
print(quantiles)
Output:
0.25 20.0
0.50 30.0
0.75 40.0
Name: values, dtype: float64
In this example, we calculated the first quartile (0.25), the median (0.5), and the third quartile (0.75) all at once. The result is a Series object that displays the quantile values, allowing for quick insights into the dataset’s distribution. This method is particularly useful in exploratory data analysis, where understanding the spread of data is crucial.
Customizing Quantile Calculation with Interpolation
By default, Pandas uses linear interpolation when calculating quantiles. However, you can customize this behavior using the interpolation
parameter within the quantile()
method. This allows you to choose from different interpolation methods such as ’linear’, ’lower’, ‘higher’, ‘midpoint’, or ’nearest’.
Here’s an example demonstrating how to use the interpolation
parameter:
import pandas as pd
data = {'values': [10, 20, 30, 40, 50, 60]}
df = pd.DataFrame(data)
quantile_with_interpolation = df['values'].quantile(0.5, interpolation='higher')
print(quantile_with_interpolation)
Output:
40.0
In this example, we specified the ‘higher’ interpolation method while calculating the median. As a result, instead of getting 30 as the median, we received 40. This flexibility is essential when dealing with datasets that may have specific requirements for quantile calculation, ensuring that you can tailor the output to your analytical needs.
Visualizing Quantiles with Box Plots
Visual representation of data can enhance understanding significantly. Box plots are an excellent way to visualize quantiles, as they display the median, quartiles, and potential outliers in a dataset. Pandas integrates well with Matplotlib, making it easy to create box plots.
Here’s how to create a box plot to visualize quantiles:
import pandas as pd
import matplotlib.pyplot as plt
data = {'values': [10, 20, 30, 40, 50, 60]}
df = pd.DataFrame(data)
plt.boxplot(df['values'])
plt.title('Box Plot of Values')
plt.ylabel('Values')
plt.show()
This code snippet creates a box plot for the ‘values’ column in the DataFrame. The box plot visually represents the median, the first and third quartiles, and any outliers present in the data. This visualization can help you quickly grasp the spread and central tendency of your dataset, making it a valuable tool for data analysis.
Conclusion
Finding quantiles in Pandas is a straightforward process that can greatly enhance your data analysis capabilities. By utilizing methods like quantile()
, you can easily compute various quantiles, customize your calculations, and visualize your data for deeper insights. Whether you’re working with large datasets or just exploring data trends, mastering these techniques will empower you to make informed decisions based on statistical analysis. So, get started with Pandas today and unlock the potential of your data!
FAQ
-
What is the purpose of quantiles in data analysis?
Quantiles help summarize and interpret datasets by dividing them into equal parts, making it easier to understand the distribution and identify outliers. -
How can I calculate quantiles for multiple columns in a DataFrame?
You can apply thequantile()
method to each column individually or use theapply()
function to calculate quantiles for multiple columns at once. -
What interpolation methods can I use when calculating quantiles?
You can use methods such as ’linear’, ’lower’, ‘higher’, ‘midpoint’, or ’nearest’ to customize how quantiles are calculated in Pandas. -
Can I visualize quantiles using other types of plots?
Yes, besides box plots, you can use histograms and violin plots to visualize the distribution of data and understand quantiles better.
- Is it possible to calculate quantiles for non-numeric data?
No, quantiles are statistical measures that apply to numeric data. Non-numeric data must be converted to a numeric format before quantiles can be calculated.