How to Append Data to CSV using Pandas
- Understanding the Basics of CSV and Pandas
- Method 1: Appending Data with DataFrame.to_csv()
- Method 2: Appending Data Using a Loop
- Method 3: Appending Data from Another DataFrame
- Conclusion
- FAQ
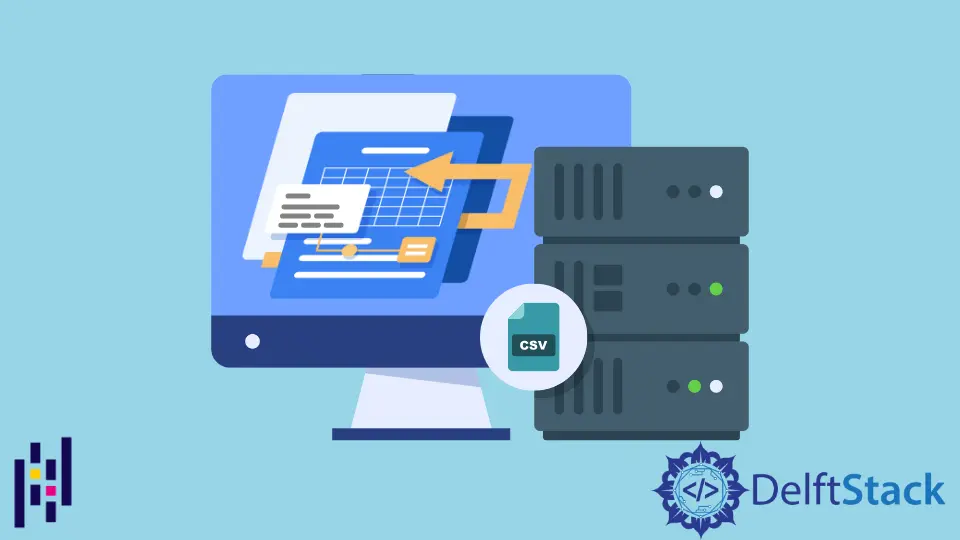
Appending data to a CSV file is a common task in data analysis and data management. Whether you’re working on a data science project or simply need to update a report, knowing how to efficiently add new rows to an existing CSV file can save you a lot of time.
In this tutorial, we’ll explore how to use the to_csv()
method in Pandas to append data to a CSV file. We’ll walk through the steps, provide clear code examples, and explain how you can leverage this powerful library to manage your data effectively. Let’s dive in!
Understanding the Basics of CSV and Pandas
CSV, or Comma-Separated Values, is a popular file format used to store tabular data. Its simplicity and compatibility with various applications make it a preferred choice for data storage. Pandas is a powerful data manipulation library in Python that provides easy-to-use data structures and data analysis tools. When you need to append data to a CSV file, the to_csv()
function in Pandas comes in handy.
The to_csv()
function allows you to write a DataFrame to a CSV file, and by using the mode='a'
parameter, you can append new data instead of overwriting existing content. This feature is particularly useful when dealing with large datasets or when you need to continuously log data over time.
Method 1: Appending Data with DataFrame.to_csv()
To append data to an existing CSV file using Pandas, the first step is to create a DataFrame that contains the new data you want to add. After that, you can utilize the to_csv()
method with the appropriate parameters. Here’s a simple example to illustrate this process.
import pandas as pd
# New data to append
new_data = {
'Name': ['Alice', 'Bob'],
'Age': [28, 34],
'City': ['New York', 'Los Angeles']
}
# Create DataFrame
df_new = pd.DataFrame(new_data)
# Append to existing CSV
df_new.to_csv('existing_file.csv', mode='a', header=False, index=False)
In this code snippet, we first import the Pandas library. We then create a new DataFrame called df_new
containing the new data we want to append. The to_csv()
function is called with the parameters mode='a'
to append the data, header=False
to avoid writing the header again, and index=False
to prevent writing row indices into the CSV file. This way, the new data seamlessly integrates with the existing content.
Output:
The new data has been appended to existing_file.csv without overwriting.
This method is straightforward and efficient for appending data to a CSV file. Remember to ensure that the structure of your new DataFrame matches the existing CSV file; otherwise, you may encounter issues with data alignment.
Method 2: Appending Data Using a Loop
Sometimes, you may want to append multiple rows to a CSV file in a loop. This is particularly useful when you are collecting data in real-time or processing data in batches. Here’s how you can achieve this using a loop.
import pandas as pd
# Data to append
data_to_append = [
{'Name': 'Charlie', 'Age': 30, 'City': 'Chicago'},
{'Name': 'David', 'Age': 25, 'City': 'Miami'}
]
# Loop through data and append to CSV
for data in data_to_append:
df = pd.DataFrame([data])
df.to_csv('existing_file.csv', mode='a', header=False, index=False)
In this example, we define a list of dictionaries called data_to_append
, where each dictionary represents a new row of data. We then loop through each item in the list, converting it to a DataFrame and appending it to the existing CSV file. The same parameters are used in to_csv()
to ensure we append without overwriting.
Output:
Multiple rows have been appended to existing_file.csv successfully.
This approach allows for dynamic data appending, making it easy to handle various data sources or user inputs. You can easily modify the data_to_append
list based on your application’s requirements.
Method 3: Appending Data from Another DataFrame
If you already have another DataFrame and want to append its content to an existing CSV file, the process is just as simple. You can directly use the to_csv()
method on the existing DataFrame. Here’s how:
import pandas as pd
# Existing DataFrame
df_existing = pd.read_csv('existing_file.csv')
# New DataFrame to append
df_new = pd.DataFrame({
'Name': ['Eve', 'Frank'],
'Age': [29, 31],
'City': ['Seattle', 'Austin']
})
# Append new DataFrame to existing CSV
df_new.to_csv('existing_file.csv', mode='a', header=False, index=False)
In this snippet, we first read the existing CSV file into a DataFrame called df_existing
. We then create another DataFrame, df_new
, which contains the new data to append. Finally, we use the to_csv()
method to append df_new
to the existing CSV file. The parameters remain the same to ensure a smooth append operation.
Output:
The new DataFrame has been successfully appended to existing_file.csv.
This method is particularly useful when you have multiple DataFrames and need to consolidate them into a single CSV file. It allows for efficient data management and organization.
Conclusion
Appending data to a CSV file using Pandas is a straightforward yet powerful technique that can significantly enhance your data management capabilities. By using the to_csv()
method with the appropriate parameters, you can easily add new rows to an existing file without losing any previous data. Whether you’re working with a single DataFrame, looping through multiple entries, or consolidating data from various sources, Pandas provides the tools necessary to make your data handling seamless. Embrace the power of Pandas, and take your data management skills to the next level!
FAQ
-
How do I check if my CSV file exists before appending data?
You can use theos.path.exists()
method from theos
module to check if a file exists before attempting to append data. -
Can I append data to a CSV file without using Pandas?
Yes, you can use Python’s built-incsv
module to append data to a CSV file, but using Pandas is generally easier and more efficient for data manipulation. -
What happens if the column names in the new data do not match the existing CSV file?
If the column names do not match, the new data may not align correctly, leading to issues in data integrity. It’s essential to ensure that the DataFrame structure is consistent. -
Is it possible to append data to a CSV file in a specific order?
Yes, you can control the order of columns in the DataFrame before appending it to the CSV file. -
How can I append data from a CSV file to another CSV file?
You can read the source CSV file into a DataFrame and then use theto_csv()
method to append it to the target CSV file, ensuring the parameters are set correctly.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn