How to Convert Timestamp Series to String in Pandas
-
Use the
dt.strftime()
Function to Convert Pandas Timestamp Series to String -
Use the
pd.Series.astype()
Function to Convert Pandas Timestamp Series to String -
Use
apply()
With thestr()
Function to Convert Pandas Timestamp Series to String - Conclusion
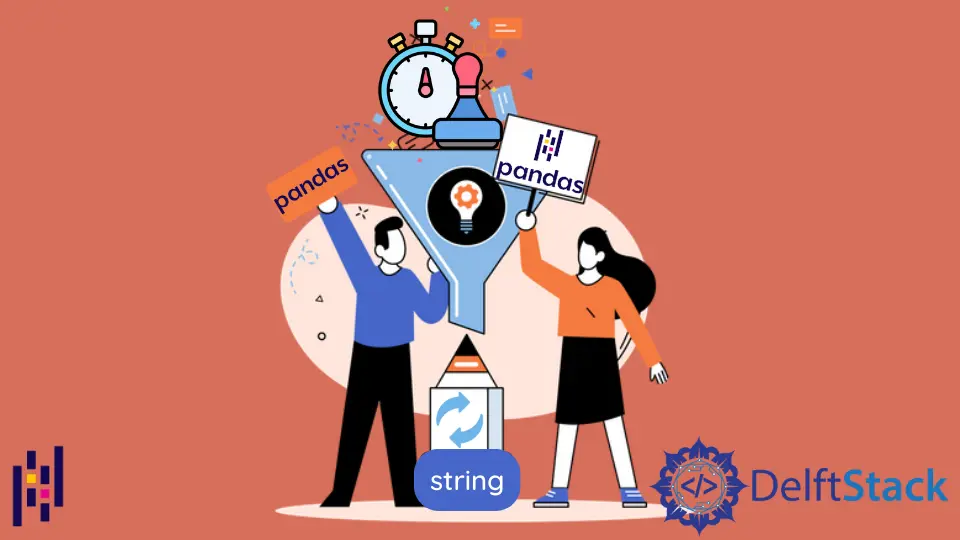
In data analysis and manipulation with Pandas, you often encounter timestamps representing date and time values. There are various scenarios where you might need to convert these timestamps to string format for visualization, reporting, or exporting data.
This article will explore different methods to convert timestamps to strings in Pandas.
Use the dt.strftime()
Function to Convert Pandas Timestamp Series to String
The strftime()
function converts a datetime object into a string. It is simply a string representation of any given datetime object.
When combined with the accessor dt
in Python as a prefix, the dt.strftime()
function can return a sequence of strings after converting them from the series of timestamps or datetime objects.
The following code uses the dt.strftime()
function to convert a Pandas timestamp series to a string in Python.
import pandas as pd
# Sample DataFrame with Timestamp
data = {"timestamp_column": ["2023-09-06 10:30:00", "2023-09-06 12:45:00"]}
df = pd.DataFrame(data)
# Convert Timestamp to String using .dt.strftime()
df["formatted_timestamp"] = pd.to_datetime(df["timestamp_column"]).dt.strftime(
"%Y-%m-%d %H:%M:%S"
)
print(df)
First, import the Pandas library as pd
. Then, create a sample DataFrame with a column containing timestamps. Replace 'timestamp_column'
and the timestamp values with your data.
Use .dt.strftime('%Y-%m-%d %H:%M:%S')
to format the timestamp series as strings.
The format string '%Y-%m-%d %H:%M:%S'
specifies how you want to format the timestamps as strings. You can customize this format according to your needs.
Finally, the formatted timestamps are added as a new column 'formatted_timestamp'
to the DataFrame.
Output:
timestamp_column formatted_timestamp
0 2023-09-06 10:30:00 2023-09-06 10:30:00
1 2023-09-06 12:45:00 2023-09-06 12:45:00
Use the pd.Series.astype()
Function to Convert Pandas Timestamp Series to String
The astype()
function will convert the data type of a Pandas DataFrame
columns. The astype()
function works alike in cases of both a single column and a set of columns.
Syntax:
df.astype(dtype, copy=True, errors="raise")
The parameters for the functions mentioned above are explained in detail below.
-
dtype
- It specifies the data type we want to convert the given timestamp series into. -
copy
- When set toTrue
, it creates a copy of the contents and makes the necessary changes. -
errors
- It specifies whether we want to allow the raising of exceptions or not. Its value can be eitherraise
orignore
.
The following example uses the astype()
function to convert a Pandas timestamp series to a string in Python.
import pandas as pd
# Sample DataFrame with Timestamp Series
data = {"timestamp_column": ["2023-09-06 10:30:00", "2023-09-06 12:45:00"]}
df = pd.DataFrame(data)
# Convert Timestamp Series to String using pd.Series.astype()
df["formatted_timestamp"] = df["timestamp_column"].astype(str)
print(df)
First, import the Pandas library as pd
; then create a sample DataFrame with a column containing timestamps. Replace 'timestamp_column'
and the timestamp values with your data.
Use .astype(str)
to directly convert the timestamp series to strings. This method is straightforward and doesn’t require specifying a format string.
Similar to the first method, the formatted timestamps are added as a new column 'formatted_timestamp'
to the DataFrame.
Output:
timestamp_column formatted_timestamp
0 2023-09-06 10:30:00 2023-09-06 10:30:00
1 2023-09-06 12:45:00 2023-09-06 12:45:00
Use apply()
With the str()
Function to Convert Pandas Timestamp Series to String
You can use .apply()
along with the str()
function to convert each timestamp in the series to a string.
import pandas as pd
# Sample DataFrame with Timestamp Series
data = {"timestamp_column": ["2023-09-06 10:30:00", "2023-09-06 12:45:00"]}
df = pd.DataFrame(data)
# Convert Timestamp Series to String using .apply() with str()
df["formatted_timestamp"] = df["timestamp_column"].apply(lambda x: str(x))
print(df)
First, import the Pandas library as pd
. Next, create a sample DataFrame with a column containing timestamps.
Replace 'timestamp_column'
and the timestamp values with your data.
Use .apply()
with a lambda function to apply the str()
function to each timestamp in the series. This lambda function converts each timestamp to a string.
Like the previous methods, the formatted timestamps are added as a new column 'formatted_timestamp'
to the DataFrame.
Output:
timestamp_column formatted_timestamp
0 2023-09-06 10:30:00 2023-09-06 10:30:00
1 2023-09-06 12:45:00 2023-09-06 12:45:00
Conclusion
In this article, we’ve explored 3 different methods to convert a Pandas timestamp series to a string: using .dt.strftime()
, pd.Series.astype()
, and .apply()
with str()
. These methods offer flexibility and can be chosen based on your needs and coding preferences.
Following the examples and explanations, you can effectively convert timestamp series to strings for various data analysis tasks.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn