How to Factorize Data Values in Pandas
- What is Factorization in Pandas?
-
Using the
factorize()
Function - Factorizing a DataFrame Column
- Handling Missing Values During Factorization
- Conclusion
- FAQ
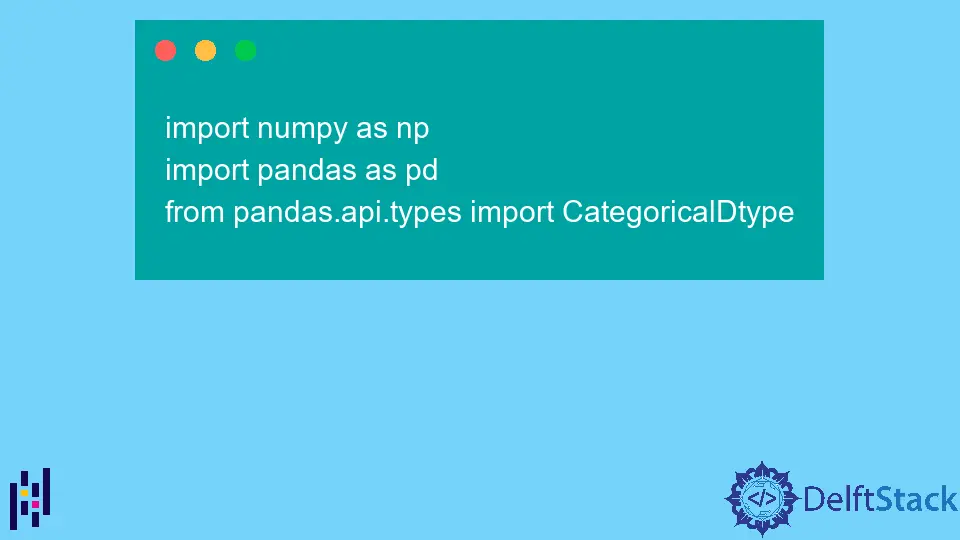
Pandas is an incredibly powerful library for data manipulation and analysis in Python. One of its many features is the ability to factorize data values, which can be particularly useful when working with categorical data. Factorization helps convert categories into numeric codes, making it easier to perform operations and analyses.
In this tutorial, we will explore how to effectively factorize data values using Pandas. Whether you’re a beginner or an experienced data analyst, understanding how to factorize data can significantly enhance your data preprocessing skills. Let’s dive in and learn how to use this valuable feature in your data analysis toolkit.
What is Factorization in Pandas?
Factorization in Pandas refers to the process of converting categorical variables into a format that can be more easily analyzed. When you have a column with distinct categories, like colors or names, factorization transforms these categories into numeric codes. This transformation not only reduces memory usage but also allows for easier manipulation of the data during analysis. For instance, if you have a column with values like ‘Red’, ‘Green’, and ‘Blue’, factorization will convert these into numeric codes like 0, 1, and 2, respectively. This process is particularly useful in machine learning and statistical modeling, where algorithms often require numeric input.
Using the factorize()
Function
Pandas provides a built-in function called factorize()
that simplifies the process of factorization. This function takes a series of categorical values and returns an array of labels and an array of unique values. Here’s how you can use it:
import pandas as pd
data = pd.Series(['Red', 'Green', 'Blue', 'Green', 'Red'])
codes, uniques = pd.factorize(data)
print(codes)
print(uniques)
Output:
[0 1 2 1 0]
['Red' 'Green' 'Blue']
The factorize()
function returns two outputs: the first is an array of integers representing the factorized values, and the second is an array of the unique categories found in the original data. In this example, ‘Red’ is represented by 0, ‘Green’ by 1, and ‘Blue’ by 2. This makes it easier to work with the data, especially when performing operations like grouping or statistical analysis.
Factorizing a DataFrame Column
In many cases, you’ll be working with a DataFrame that contains multiple columns. Factorizing a specific column is straightforward with the factorize()
function. Let’s see how this can be done:
df = pd.DataFrame({
'Color': ['Red', 'Green', 'Blue', 'Green', 'Red'],
'Value': [10, 20, 30, 20, 10]
})
df['Color_Code'], uniques = pd.factorize(df['Color'])
print(df)
Output:
Color Value Color_Code
0 Red 10 0
1 Green 20 1
2 Blue 30 2
3 Green 20 1
4 Red 10 0
In this example, we create a DataFrame with two columns: ‘Color’ and ‘Value’. We then factorize the ‘Color’ column and store the resulting codes in a new column called ‘Color_Code’. The resulting DataFrame shows the original colors alongside their corresponding numeric codes, making it easier to analyze and visualize the data.
Handling Missing Values During Factorization
When working with real-world datasets, it’s common to encounter missing values. The factorize()
function handles these gracefully by assigning a unique code for NaN values. Here’s how it works:
data_with_nan = pd.Series(['Red', 'Green', None, 'Blue', 'Red'])
codes, uniques = pd.factorize(data_with_nan)
print(codes)
print(uniques)
Output:
[ 0 1 -1 2 0]
['Red' 'Green' 'Blue']
In this case, the missing value (None) is represented by -1 in the codes array. The unique values array only contains the non-null categories. This feature is particularly useful as it allows you to retain the integrity of your data while still performing factorization, ensuring that you can analyze datasets without losing track of missing entries.
Conclusion
Factorizing data values in Pandas is a powerful technique that can streamline your data analysis process. By converting categorical variables into numeric codes, you make it easier to perform various operations and analyses. Whether you’re dealing with simple series or complex DataFrames, the factorize()
function provides a straightforward solution for handling categorical data. With the insights gained from this tutorial, you can enhance your data preprocessing skills and prepare your datasets for more effective analysis. Happy coding!
FAQ
-
What is the purpose of factorization in Pandas?
Factorization is used to convert categorical data into numeric codes, making it easier to analyze and manipulate. -
Can I factorize multiple columns in a DataFrame?
Yes, you can apply thefactorize()
function to multiple columns individually or use it in a loop for all categorical columns. -
How does Pandas handle missing values during factorization?
Pandas assigns a unique code (typically -1) to missing values when using thefactorize()
function. -
Is factorization necessary for machine learning?
Yes, many machine learning algorithms require numeric input, so factorizing categorical data is often a necessary preprocessing step. -
Can I reverse the factorization process in Pandas?
Yes, you can use the unique values array returned by thefactorize()
function to map numeric codes back to their original categories.