How to Convert a Pandas Series of Datetime to String in Python
-
Method 1: Using the
astype
Function -
Method 2: Using the
dt.strftime
Method - Method 3: Using List Comprehension
- Conclusion
- FAQ
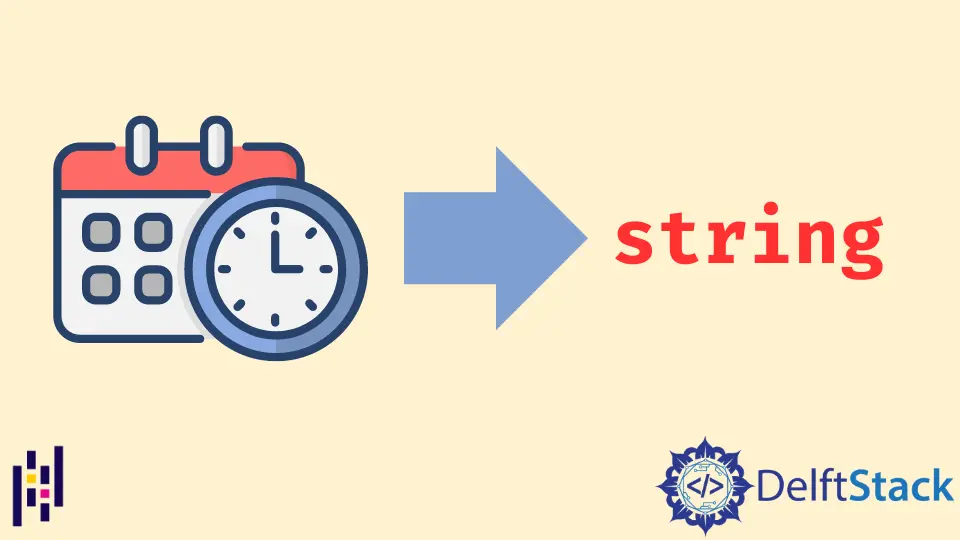
Converting a Pandas Series of datetime objects to strings is a common task in data manipulation and analysis. Whether you’re preparing data for visualization, exporting to a CSV, or simply cleaning your dataset, understanding how to handle datetime formats is essential. In Python, the Pandas library provides powerful tools to work with time series data, making it easy to convert datetime objects to string representations.
In this article, we will explore several methods to achieve this, ensuring you have a comprehensive understanding of how to manipulate datetime formats effectively. So, let’s dive into the world of Pandas and learn how to convert datetime to string in Python.
Method 1: Using the astype
Function
One of the simplest ways to convert a Pandas Series of datetime objects to strings is by using the astype
function. This method allows you to specify the desired string format directly. The astype
function is versatile and can be applied to many data types, making it a go-to option for many data transformation tasks.
Here’s how you can use the astype
function to convert a datetime Series to string:
import pandas as pd
# Create a sample datetime Series
datetime_series = pd.Series(pd.date_range("2023-01-01", periods=5, freq='D'))
# Convert to string
string_series = datetime_series.astype(str)
print(string_series)
Output:
0 2023-01-01 00:00:00
1 2023-01-02 00:00:00
2 2023-01-03 00:00:00
3 2023-01-04 00:00:00
4 2023-01-05 00:00:00
dtype: object
In this example, we first create a Pandas Series of datetime objects ranging from January 1, 2023, to January 5, 2023. By applying the astype(str)
method, we convert each datetime entry into its string representation. The output shows that each datetime is now formatted as a string, including the time component. This method is straightforward and works well for basic conversions, but it does not allow for custom formatting.
Method 2: Using the dt.strftime
Method
For more control over the output format, the dt.strftime
method is an excellent choice. This method allows you to specify a format string that defines how the datetime should be represented as a string. The format codes are similar to those used in Python’s built-in strftime
function, giving you flexibility in how you display dates and times.
Here’s an example of how to use dt.strftime
:
import pandas as pd
# Create a sample datetime Series
datetime_series = pd.Series(pd.date_range("2023-01-01", periods=5, freq='D'))
# Convert to string with a specific format
string_series = datetime_series.dt.strftime('%Y-%m-%d')
print(string_series)
Output:
0 2023-01-01
1 2023-01-02
2 2023-01-03
3 2023-01-04
4 2023-01-05
dtype: object
In this example, we again create a datetime Series. However, this time we use dt.strftime('%Y-%m-%d')
to convert the datetime objects into strings formatted as “YYYY-MM-DD”. The output clearly shows that the time component has been omitted, and we have a clean date format. The dt.strftime
method is particularly useful when you need to customize the output format for reporting or data export purposes.
Method 3: Using List Comprehension
If you prefer a more manual approach or want to apply a specific transformation that is not covered by the built-in methods, you can use list comprehension. This method involves iterating through the Series and applying a string conversion to each datetime object.
Here’s how to implement this approach:
import pandas as pd
# Create a sample datetime Series
datetime_series = pd.Series(pd.date_range("2023-01-01", periods=5, freq='D'))
# Convert to string using list comprehension
string_series = pd.Series([dt.strftime('%d-%m-%Y') for dt in datetime_series])
print(string_series)
Output:
0 01-01-2023
1 02-01-2023
2 03-01-2023
3 04-01-2023
4 05-01-2023
dtype: object
In this example, we create a datetime Series and then use list comprehension to iterate over each datetime object. We apply the strftime
method directly within the list comprehension to format each date as “DD-MM-YYYY”. The result is a new Series where each entry is a string representation of the datetime in the specified format. This method is flexible and can be tailored to suit various formatting needs, making it a powerful option for custom transformations.
Conclusion
Converting a Pandas Series of datetime objects to strings is a fundamental task in data analysis. Whether you choose to use the astype
function for simplicity, the dt.strftime
method for custom formatting, or list comprehension for more complex transformations, each method has its advantages. Understanding these techniques will enhance your data manipulation skills and enable you to prepare your datasets for further analysis or visualization. By mastering these methods, you’re well on your way to becoming proficient in handling datetime data in Python.
FAQ
-
What is a Pandas Series?
A Pandas Series is a one-dimensional array-like object that can hold any data type, including datetime objects, and is part of the Pandas library. -
Can I convert datetime objects to different formats?
Yes, you can use thedt.strftime
method to specify custom formats for converting datetime objects to strings. -
Is using
astype(str)
sufficient for all datetime conversions?
Whileastype(str)
is simple and effective, it does not allow for custom formatting. Usedt.strftime
for more control over the output. -
What is the difference between
dt.strftime
and list comprehension?
dt.strftime
is a built-in method for formatting datetime objects, while list comprehension allows for custom transformations and can be tailored to specific needs. -
How do I handle timezone-aware datetime objects in Pandas?
You can use thedt.tz_convert
method to adjust timezone-aware datetime objects before converting them to strings.