How to Fix Error - Module Pandas Has No Attribute Dataframe Error
- Understanding the Error
- Method 1: Check Your Pandas Installation
- Method 2: Avoid Naming Conflicts
- Method 3: Correct Import Statements
- Conclusion
- FAQ
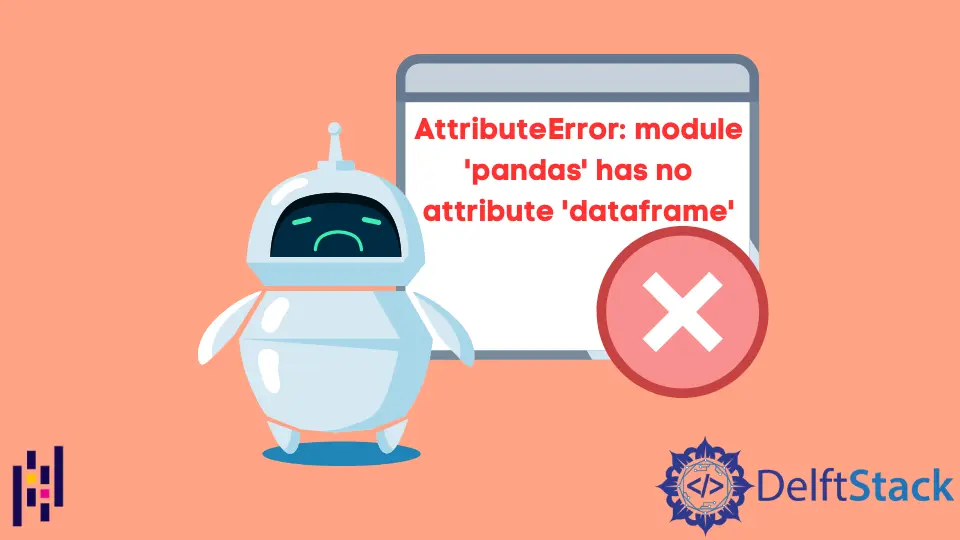
When working with Pandas, a popular data manipulation library in Python, encountering the error “module pandas has no attribute DataFrame” can be frustrating. This error usually arises due to various reasons, such as incorrect installation, naming conflicts, or improper imports.
In this tutorial, we’ll explore the common causes of this error and provide effective solutions to fix it. By the end of this article, you’ll have a clear understanding of how to troubleshoot and resolve this issue, allowing you to continue your data analysis tasks smoothly. So, let’s dive in and get your Pandas working seamlessly again!
Understanding the Error
Before we jump into the solutions, it’s essential to understand what the error means. The “module pandas has no attribute DataFrame” error occurs when Python cannot find the DataFrame class in the Pandas module. This can happen for several reasons, including:
- Incorrect installation of the Pandas library.
- A naming conflict with your script or variable names.
- Importing the library incorrectly.
By identifying the root cause, you can apply the appropriate solution to get back on track.
Method 1: Check Your Pandas Installation
The first step in resolving this error is to ensure that Pandas is correctly installed in your Python environment. Sometimes, a faulty installation can lead to missing attributes. You can check your installation using the following command:
pip show pandas
Output:
Name: pandas
Version: 1.3.3
Summary: Powerful data structures for data analysis, time series, and statistics
Home-page: https://pandas.pydata.org/
Author: Wes McKinney
If Pandas is not installed, or if you suspect an issue with the current version, you can reinstall it using:
pip install --upgrade --force-reinstall pandas
Output:
Collecting pandas
Downloading pandas-1.3.3-cp39-cp39-manylinux1_x86_64.whl (10.2 MB)
Installing collected packages: pandas
Successfully installed pandas-1.3.3
By confirming your installation and ensuring you have the latest version, you can eliminate installation-related issues. After reinstalling, try importing Pandas again and check if the error persists.
Method 2: Avoid Naming Conflicts
Another common cause of the “module pandas has no attribute DataFrame” error is naming conflicts in your code. If you have a variable or a script named “pandas.py”, Python may confuse that with the actual Pandas library. To resolve this, follow these steps:
- Check your script name. If it’s named “pandas.py”, rename it to something else, like “data_analysis.py”.
- Ensure you don’t have any variables named “pandas”.
After making these changes, try importing Pandas again:
import pandas as pd
df = pd.DataFrame({'A': [1, 2], 'B': [3, 4]})
print(df)
Output:
A B
0 1 3
1 2 4
By avoiding naming conflicts, you can help Python correctly identify and access the Pandas library. Always ensure that your variable names and script names do not clash with library names to prevent such errors.
Method 3: Correct Import Statements
Improper import statements can also lead to the “module pandas has no attribute DataFrame” error. Ensure you are importing Pandas correctly. The standard way to import Pandas is as follows:
import pandas as pd
data = {'A': [1, 2], 'B': [3, 4]}
df = pd.DataFrame(data)
print(df)
Output:
A B
0 1 3
1 2 4
In this code, we import Pandas as “pd” and then create a DataFrame using the pd.DataFrame()
method. If you mistakenly write:
from pandas import DataFrame
df = DataFrame({'A': [1, 2], 'B': [3, 4]})
print(df)
You might encounter the error if there are issues with the import. Always use the standard import method and ensure that you are not importing specific classes or functions incorrectly. This will help maintain clarity and prevent conflicts.
Conclusion
Encountering the “module pandas has no attribute DataFrame” error can be a stumbling block, but with the right approach, you can resolve it efficiently. By checking your installation, avoiding naming conflicts, and ensuring correct import statements, you can eliminate this error and continue your data analysis without interruption. Remember, maintaining good coding practices, such as proper naming conventions and organized imports, will help you avoid similar issues in the future. Happy coding!
FAQ
-
What does the error “module pandas has no attribute DataFrame” mean?
This error indicates that Python cannot find the DataFrame class in the Pandas module, often due to installation issues or naming conflicts. -
How can I check if Pandas is installed correctly?
You can use the commandpip show pandas
to check the installation details. If it’s not installed, you can install it usingpip install pandas
. -
Can naming my script “pandas.py” cause this error?
Yes, naming your script “pandas.py” can create a naming conflict, leading to this error. It’s best to rename your script to something unique. -
What is the correct way to import Pandas?
The standard way to import Pandas is usingimport pandas as pd
. This allows you to access all Pandas functionalities without conflicts. -
Is there a way to resolve this error without reinstalling Pandas?
Yes, you can resolve it by checking for naming conflicts and ensuring correct import statements. Reinstalling is usually a last resort.