How to Use a Production WSGI Server to Run a Flask App
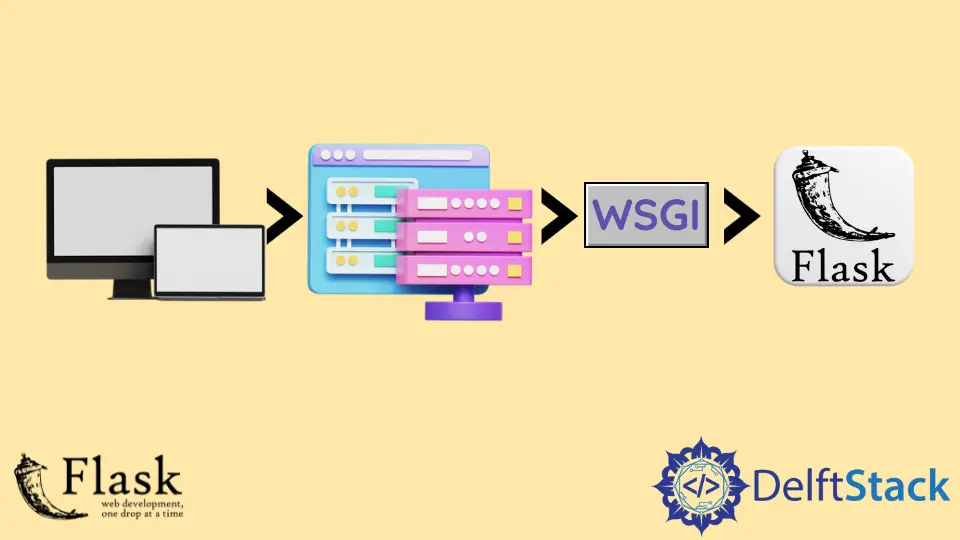
We will learn, with this explanation, about the WSGI server and see how it works. We will also learn how to create a WSGI server and run an app inside this server in Flask and Python.
Create a WSGI Server and Run an App Inside the Server in Flask and Python
The Web Server Gateway Interface, also known as WSGI, follows the process when it gets the request from the client that points to a function or a class with lines of code. Each line of code is executed sequentially, and finally, the response is provided to the client.
Then the application handles the subsequent request and follows the same process until the client receives a response.
A question is raised about the web application: is the whole handling only one request at a time? No, the workers come to support and handle multiple requests simultaneously.
When deploying applications built on Django or Flask, we must have specified the number of workers handling requests, which is done in WSGI servers such as Gunicorn. These workers handle multiple requests from clients at a time.
For example, we take an analogy of making breakfast; in this case, the person prepares the tea and the second person makes an egg, so two workers are working in the kitchen at a time.
The question is: can we specify to have hundreds of workers handle tasks simultaneously? No, because size matters, and we can only fit as many workers as the size of the kitchen.
Similarly, we can only set up workers until server resources are exhausted. More number of workers will be needed for more server resource utilization.
Generally, the recommended number of workers is twice the number of CPU cores + 1, so in the case of a server with 6 CPU cores would be 13 workers.
Now, we will see how to create a basic function-based Web Server Gateway Interface in Flask. First, we will create a directory inside the root
directory called web
and create an app.py
file inside this directory.
Now, we will need to import a package called wsgiref
to use WSGI, which is a good start for us to build a WSGI. Now we will import the make_server
function from simple_server
, allowing us to make a WSGI server.
from wsgiref.simple_server import make_server
Next, we will create our web app, which will be a very simple way to create this app. We will define a function and call it Web_App()
.
It takes two required parameters. The first one is the environment
parameter which is a CGI-style variable.
The next parameter is response
, and we obviously want to see our page. Also, in the response, we usually respond to important information with headers like an HTML document or a text document.
Next, we will create a variable that will hold our status, a string. We will say 200 OK
that must be 4 characters long.
def Web_App(environment, response):
status = "200 OK"
Now, we need to create headers, which will accept a list, and a tuple would be its item because we can add multiple headers inside this list as its item. In this tuple, we will declare what kind of content type we are passing.
Now, we will set our response using status
and headers
like the following. And we go ahead and return our content to our website.
The content will be returned in binary data.
headers = [("content-type", "text/html; charset=utf-8")]
response(status, headers)
return [b"<h2>Hi there, this is WSGI server</h2>"]
Let’s go ahead and create a server using the with
block and use the make_server()
function with this statement. It takes a couple of arguments.
The first is the host that would be localhost by default which is why we leave an empty string. The next is the port that would be 5000
, and then we need to pass the Web_App()
function in the next argument.
This argument is to display our app or whatever content we want to serve. Now we will print a message to the user, letting them know where they would find their website and how to exit the server.
Now, we will serve up our server and use the serve_forever()
method, which means serve the server until we kill it via the terminal or if we have an error somewhere and it kills itself.
with make_server("", 5000, Web_App) as server:
print(
"serving on port 5000...\nvisit http://127.0.0.1:5000\nTo exit press ctrl + c"
)
server.serve_forever()
Let’s go ahead and run the server, then go to the browser where our app is running. When we visit, we shall see the content displayed as we defined inside our app.
To learn more about the WSGI server, click here to read the official docs.
Complete Python Code:
from wsgiref.simple_server import make_server
def Web_App(environment, response):
status = "200 OK"
headers = [("content-type", "text/html; charset=utf-8")]
response(status, headers)
return [b"<h2>Hi there, this is WSGI server</h2>"]
with make_server("", 5000, Web_App) as server:
print(
"serving on port 5000...\nvisit http://127.0.0.1:5000\nTo exit press ctrl + c"
)
server.serve_forever()
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn