How to Create Dynamic URL Using url_for in Flask
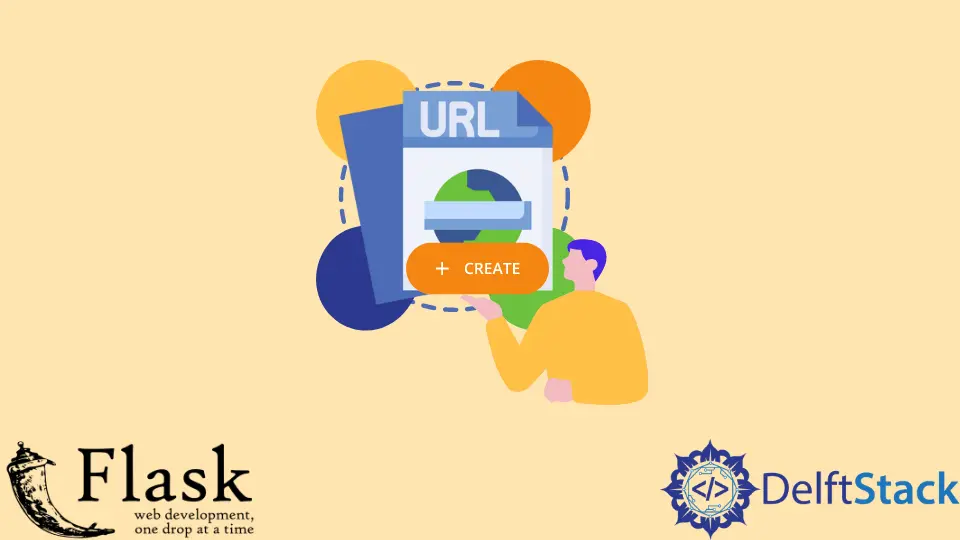
We will learn, with this explanation, what the url_for()
function does and how we can create a dynamic URL in Flask. We will also learn how to use url_for()
inside the template.
Create Dynamic URL With the Help of the url_for()
Function in Flask
url_for()
is a function in Flask that returns the URL used for a particular view function. And with that URL, you could either redirect the user to that page or only display that URL.
To demonstrate, we will show you how we do that. So, let’s start importing Flask along with url_for
and redirect
.
from flask import Flask, url_for, redirect
These are the two functions we will use in this example, and then we will have a route on the BASE_FUNC()
view.
@app.route("/")
def BASE_FUNC():
return "Hi there,the base index page"
Now we will create another route, which we will call FIRST_FUNC
, and we will return url_for('BASE_FUNC')
, which is the name of another function. The url_for()
function takes the function name and returns the route of that function which we passed inside the url_for()
function.
@app.route("/first")
def FIRST_FUNC():
return url_for("BASE_FUNC")
If we try to access this route, /first
, we will get the URL associated with the BASE_FUNC()
function, which means we will obtain its route. Let’s save this and run the server.
If we type this endpoint, /first
, we will only get a slash:
Let’s create another route and call it /second
with the function name SECOND_FUNC()
, then we will return url_for('THIRD_FUNC', user_name='Harry')
. In a Flask, the route even works with parameters, so we will need to create another route with a parameter that would be a string.
We will create a route like this /third/<string:user_name>
, and make sure you are using a parameter with the same name inside the route as you passed the url_for()
function. Now we will create a new function called THIRD_FUNC()
and pass it a parameter with the same name.
We will return the user_name
parameter and access this function from the other function’s route using the url_for()
function.
@app.route("/second")
def SECOND_FUNC():
return url_for("THIRD_FUNC", user_name="Harry")
@app.route("/third/<string:user_name>")
def THIRD_FUNC(user_name):
return "The user name is " + user_name
If we type /second
, it will give us the THIRD_FUNC()
function’s route:
We can use URLs or links to redirect the user to a specific page in the Flask application, and now instead of returning just the URL, we can redirect the user to a particular URL. We will need to call the redirect()
function, which takes the URL where we want to redirect the user.
redirect(url_for("THIRD_FUNC", user_name="Harry"))
Now, when we call /second
, it will get the URL for the /third/<string:user_name>
route and send us to that third route.
Now we will show you how to use url_for()
inside the templates using the Jinja template; we will import render_template()
and use it for rendering an HTML file called index.html
. We will use another route that will return a string.
@app.route("/")
def BASE_FUNC():
return render_template("index.html")
@app.route("/page")
def Page_FUNC():
return "Hello"
We will create an index.html
file inside the templates folder and then add a link to the for /page
route. We will pass the Page_FUNC
inside the url_for()
instead of passing a link.
<a href="{{url_for('Page')}}">Page</a>
Let’s save and refresh the page:
Now we will make it a little more complicated; we will add a dynamic element that can be passed along with /page
that would be the parameter. We will use this parameter inside the template so that the second keyword argument can be whatever arguments need to pass to that route function.
In our case, user_name
would be equal to Josh
:
<a href="{{ url_for('Page_FUNC',user_name='Josh') }}">Page</a>
Output:
Complete Python Source Code:
from flask import Flask, url_for, render_template
app = Flask(__name__)
# @app.route("/")
# def BASE_FUNC():
# return "Hi there,the base index page"
# @app.route("/first")
# def FIRST_FUNC():
# return url_for('BASE_FUNC')
# @app.route("/second")
# def SECOND_FUNC():
# return redirect(url_for('THIRD_FUNC', user_name='Harry'))
# @app.route("/third/<string:user_name>")
# def THIRD_FUNC(user_name):
# return "The user name is " + user_name
@app.route("/")
def BASE_FUNC():
return render_template("index.html")
@app.route("/page/<string:user_name>")
def Page_FUNC(user_name):
return "Hello " + user_name
if __name__ == "__main__":
app.run(debug=True)
Complete HTML Source Code:
<html>
<head>
<body>
<h1>Hi User, this is Base page</h1>
<a href="{{ url_for('Page_FUNC',user_name='Josh') }}">Page</a>
</body>
</head>
</html>
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn