How to Use Different Types of Parameters Inside the URL in Flask
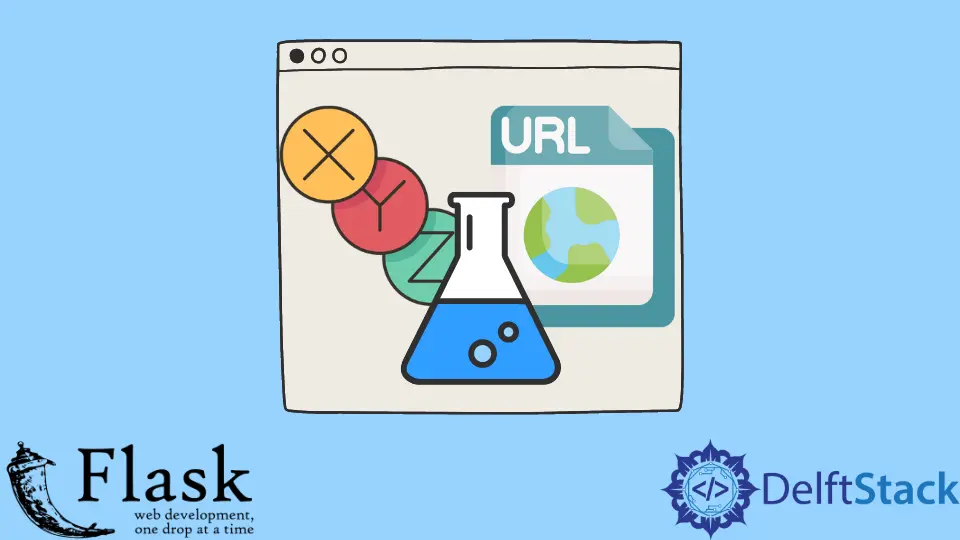
We will learn, with this explanation, about URL converters in the Flask app, which means the ability to pass parameters in any URL. We will also learn how to use different types of parameters inside the URL in the Flask app.
Use Different Types of Parameters Inside the URL in Flask
Now we will discuss URL parameters and demonstrate them; we’ll start with the default, which is the most basic example. To do that, we will create a route and pass in the route()
that would be '/default/<params>'
.
The /default
will lead to the URL. The second part of the URL is the parameter, where we specify the parameter within the greater-and-lesser-than symbol.
Then we will create a function called DEFAULT()
and pass the same name for the function parameter we defined in our route, so in this case, the parameter would be params
. Then we will return the value and concatenate the value with this string.
from flask import Flask
app = Flask(__name__)
@app.route("/default/<params>")
def DEFAULT(params):
return "The parameter is: " + params
if __name__ == "__main__":
app.run(debug=True)
Let us start our app and open up the browser, and after typing /default
when we pass a name, we will see the value of a parameter that is a string by default.
We will show you the other data types that you can use, but for the analysis of the string, we will show you that if we pass a number and decimal number, then these will all work because it gets converted to a string. This way, we will get the value of whatever we passed in the endpoint.
If we do not pass the parameter, we get a not found error because it expects a parameter. To handle this case, when the user does not pass anything, we will specify another route and just put it above.
Inside this route, we will pass a keyword argument called defaults
and take params
and its value.
@app.route('/default', defaults={'params': 'John'})
Now we will restart it, and if we go with the URL without passing a parameter, we get the default value that is John
, but if we pass the value, then we will get this value instead of the default value.
Let’s pass a data type as a string in our routes to specify that this can only be a string. We put string
first, then a colon, and then the parameter’s name.
We will define another function for this route called String_Func()
and return the string and concatenate the parameter we defined in the route.
@app.route("/str-url/<string:str_params>")
def String_Func(str_params):
return "The string parameter is: " + str_params
We can see that this is working the same as the default route works because, by default, the parameter type is a string.
Now let’s see what happens when we want to deal with different data types, and the next data type would be an integer. Let’s define another route pass <int:int_params>
; the parameter would be whatever you call it.
Then we define a function as usual and convert it into a string data type because we can not concatenate a string to an integer.
@app.route("/int-url/<int:int_params>")
def Int_Func(int_params):
return "The integer parameter is: " + str(int_params)
Output
The next data type we use would be a float, beginning with <float:float_params>
.
@app.route("/float-url/<float:float_params>")
def Float_Func(float_params):
return "The float parameter is: " + str(float_params)
If we pass 3.5, the page will display it, but if we pass only 3, we get an error because 3 is an integer, and the Flask does not allow it that way. If you want 3.0, you must pass 3.0 or create another route that will pick up any integers.
One more thing is a path URL so that we will define a new route for it, and we will be needed to pass path data type and path name such as <path:our_path>
.
@app.route("/path-route/<path:our_path>")
def Path_Func(our_path):
return "Our path is: " + our_path
Now we will go to the URL and give it the path, then it displays this, but if we put some slashes in and specify another subdirectory, it picks it all and includes the slashes.
The last thing we want to show you is that you can combine more than one parameter in the same route. We will combine string and integer parameters such as <string:name>/<int:num>
.
Let’s define a function and specify both parameters inside the function and return it.
@app.route("/combine-route/<string:name>/<int:num>")
def Combine_Func(name, num):
return "User name is: " + name + " and user id is: " + str(num)
Look at the output so that you can understand it better.
Complete Source Code of the Flask App:
from flask import Flask
app = Flask(__name__)
# This is the default route
@app.route("/default", defaults={"params": "John"})
@app.route("/default/<params>")
def DEFAULT(params):
return "The string parameter is: " + params
# This route holds string data type
@app.route("/str-url/<string:str_params>")
def String_Func(str_params):
return "The string parameter is: " + str_params
# This route holds int data type
@app.route("/int-url/<int:int_params>")
def Int_Func(int_params):
return "The integer parameter is: " + str(int_params)
# This route holds float data type
@app.route("/float-url/<float:float_params>")
def Float_Func(float_params):
return "The float parameter is: " + str(float_params)
# This route holds path data type
@app.route("/path-route/<path:our_path>")
def Path_Func(our_path):
return "Our path is: " + our_path
# This route combine two parameters
@app.route("/combine-route/<string:name>/<int:num>")
def Combine_Func(name, num):
return "User name is: " + name + " and user id is: " + str(num)
if __name__ == "__main__":
app.run(debug=True)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn