How to Create a Static Folder in Flask
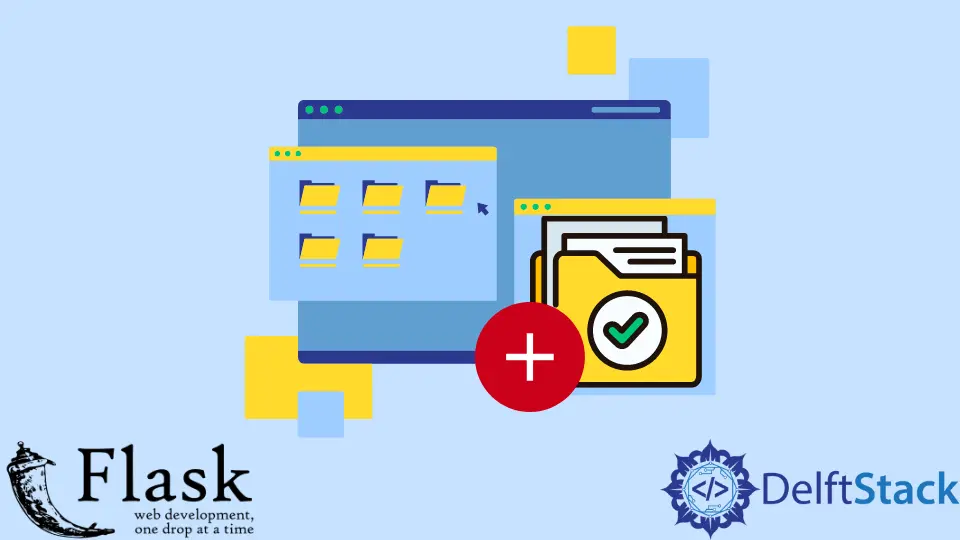
We will learn, with this explanation, how to use static assets or static files inside the static directory. We will also learn how to import our custom CSS file into Flask.
Create a Static Folder in Flask
In a Flask app, there is a weird way of displaying images and loading in your custom CSS classes and using your custom JavaScript. So, let’s go ahead and get started.
Since we are starting from scratch, we will create a brand new project. We will import some classes from Flask, write the module in lowercase, and then create an app object.
from flask import Flask, render_template
app = Flask(__name__)
We will create a basic home page function called index()
. We will set the two roots in individual decorators as '/home'
and '/'
.
The purpose of adding two roots is to show you that we can use more than one route()
in a single function, which means both of these different routes can access this function.
We will use the return
keyword to return the render_template()
and pass the home.html
file.
@app.route("/home")
@app.route("/")
def index():
return render_template("home.html")
To run our app, we need to use the below code.
The run()
method will help run the Flask app. We must pass the debug
equals True
because we want to see the debugging, but you can turn it off by writing False
when you bring your site to the production level.
if __name__ == "__main__":
app.run(debug=True)
Now, we need to set up a template folder where we will put all our HTML files; to do that, we will make a new folder and call it templates
. Then, we will need to make another new folder, which we will call static
.
Now, we might already be able to tell where we will put specific files. We will go inside the templates
folder and create a new file called base.html
, and then we will make a new one called home.html
.
Let’s write a very basic HTML code inside the base.html
file, and in the head
, we will set up a block title, and inside the body
tag, we will add another block content.
Flask application sets a default for all of our static
files, which will be saved in the folder called static
, which means you need to make this folder static. Now, we will reference the specific file name that we want; we will pass {{ url_for('static',filename='style.css') }}
to the href
attribute and put double quotes around it.
In this way, we will use the Python code in the link
tag to grab the URL for the static
folder, which will give us the URL and then specify the exact file name.
<html>
<head>
<title>{% block title %}{% endblock %}</title>
<link rel="stylesheet" type="text/css" href="{{ url_for('static',filename='style.css') }}">
</head>
<body>
{% block content %}{% endblock %}
</body>
</html>
Now, we have our base
template. Let’s extend this base
template using the {% extends 'base.html' %}
jinja code inside our home.html
file and use some blocks to access base.html
elements.
{% extends 'base.html' %}
{% block title %}
Home Page
{% endblock %}
{% block content %}
<h1> Home Page</h1>
{% endblock %}
Now, we will talk about creating a static
file inside of our static
directory and how we can import our custom CSS. We will need to create a new CSS file inside our static
directory and call it style.css
; it does not matter what you call it.
You can change the file name instead of the extension but make sure that you remember the name. We will now write just a basic styling inside the style.css
file to keep it simple.
We will set a CSS body
selector, use the color
property, and then pass the crimson
color value.
body {color: crimson;}
And we are done. Now, we are good to go and run this application.
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/home")
@app.route("/")
def index():
return render_template("home.html")
if __name__ == "__main__":
app.run(debug=True)
Now, open a web browser, and navigate to the homepage with a heading.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn