How to Return a Valid JSON Response in Flask
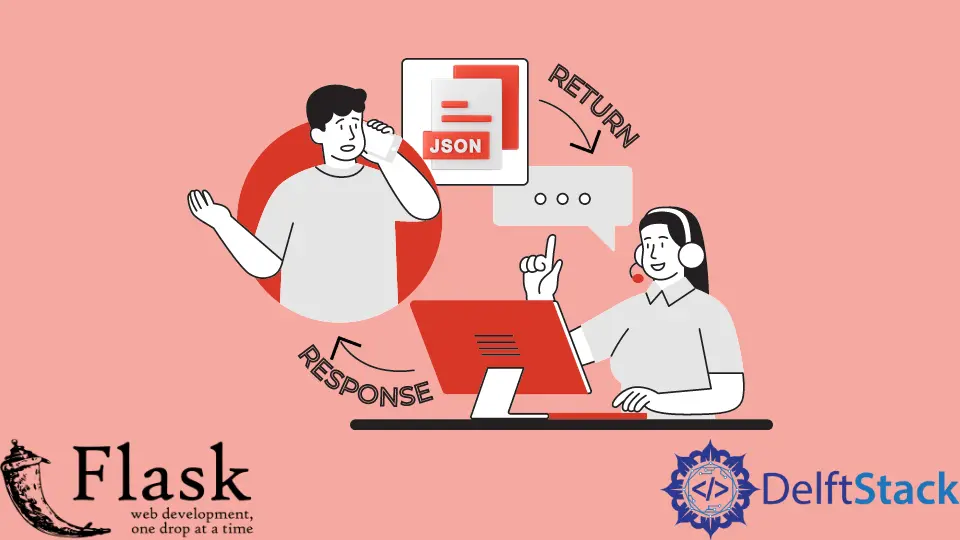
We will learn, with this explanation, about JSON support in Flask and how to create an API and return it in JSON response with the help of jsonify()
in Flask.
Return a Valid JSON Response With the Help of jsonify()
in Flask
While using JSON with Flask is pretty simple because the type of the JSON object can be mapped to Python types, and we can access it like a dictionary or array. If you are familiar with JSON’s basics, then you should be able to understand this.
Now we will need to set up a Flask app. We are just doing some basic things to create an app, and then we will create a single route.
We will specify a root for Get_Trucks()
method that is route('truck/api/',methods=['GET'])
inside the decorator. And inside the Get_Trucks()
method, we will declare a list of dictionaries that is called Trucks
and return it directly.
@app.route("truck/api/", methods=["GET"])
def Get_Trucks():
Trucks = [
{"id": 1, "year": 2017, "model": ""},
{"id": 2, "year": 2019, "model": ""},
{"id": 3, "year": 2020, "model": ""},
{"id": 4, "year": 2016, "model": ""},
]
return Trucks
Now, we will look at two cases: the first is returning an array of objects, and the second is just returning one object by itself or one dictionary.
Let’s go ahead and take a look at the second one. The code will be the same except for a function named Truck_Details()
.
from flask import Flask
app = Flask(__name__)
@app.route("/truck/api/", methods=["GET"])
def Get_Trucks():
Trucks = [
{"id": 1, "year": 2017, "model": ""},
{"id": 2, "year": 2019, "model": ""},
{"id": 3, "year": 2020, "model": ""},
{"id": 4, "year": 2016, "model": ""},
]
return Trucks
@app.route("/truck-details/api/", methods=["GET"])
def Truck_Details():
Details = {"id": 2, "year": 2019, "model": ""}
return Details
if __name__ == "__main__":
app.run(debug=True)
Now, we will run the Flask app and go to the browser. Let’s test this first route.
When we hit Enter, we get the type error where we can read that the view
function did not return a valid response because we’re trying to send a Python list to the browser.
We can fix this very quickly by importing one more thing from Flask: jsonify()
. This is a built-in flask; we do not need to use a third-party module.
We will go to where we are returning our list or array and wrap it in jsonify()
. Let’s save this and test our route again, and now we are getting a valid JSON response.
If we look at this in the network inspector, we can see this has a content type of application is JSON.
Let’s look at returning a single object from the Flask without using jsonify()
. Let’s take a look at it in the browser.
It works because we are using an upgraded version. If you use the Flask version 1.1.0 or higher, it will automatically convert the dictionary to JSON when it is returning it in a view
, but if your version of the Flask is less than 1.1.0, then you can do the same thing as we did to returning multiple objects.
If we look at the right side, we can see that the content type is JSON, and we are just using a Chrome extension called JSON view to prettify the response data.
Full source code:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route("/truck/api/", methods=["GET"])
def Get_Trucks():
Trucks = [
{"id": 1, "year": 2017, "model": ""},
{"id": 2, "year": 2019, "model": ""},
{"id": 3, "year": 2020, "model": ""},
{"id": 4, "year": 2016, "model": ""},
]
return jsonify(Trucks)
@app.route("/truck-details/api/", methods=["GET"])
def Truck_Details():
Details = {"id": 2, "year": 2019, "model": ""}
return Details
if __name__ == "__main__":
app.run(debug=True)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn