How to Handle Request Data in JSON Format in Flask
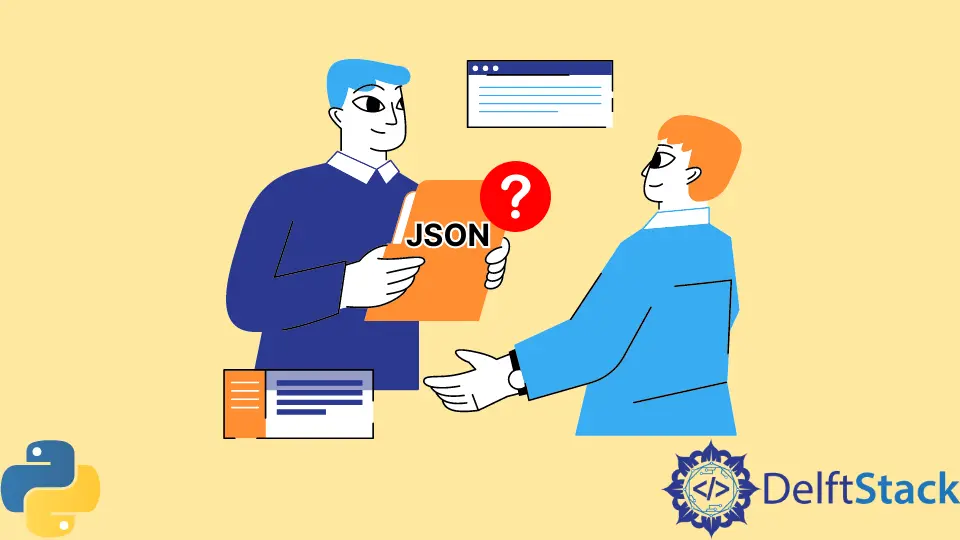
We will learn, with this explanation, what JSON is and how to handle incoming request data in JSON format. We will also learn how to use the Postman Chrome extension to send JSON data.
Handle Request Data in JSON Format in Flask
JSON is a compact, readable text format for structuring data. JSON stands for JavaScript Object Notation; it is a text format that is completely language-independent.
It starts and ends with curly brackets. The JSON format is very easy to read for humans, and it is also easy to parse and generate for computers.
There is one more way to parse request data to your Flask app, and that method will be using a JSON object. To demonstrate this, we will use Postman, a tool in Chrome that allows you to send HTTP requests to particular URLs.
The first thing we must do is create a route for handling JSON. We will call this /post-data
, which will take POST
request only, but it just depends on what you want to use the HTTP method.
Now we will define a method called POST_REQ_DATA()
. We will return a JSON object, jsonify()
, and put a dictionary inside this.
@app.route("/post-data", methods=["POST"])
def POST_REQ_DATA():
return jsonify({"Post_Request": "Sent"})
If we go to Postman and switch to a POST
request, type the /post-data
and send it, we will see the output that we sent through the jsonify()
.
But for now, it is not taking any kind of request data. To access JSON requests, we will go back into our Flask app, and inside the function, we will call the request.get_json()
method.
This method will take the incoming JSON object and convert it to Python data structures. When we pass it to jsonify()
, it will find an object on a JSON object and convert it to Python dictionaries, and arrays in the JSON object will be converted to lists in Python.
Now we will declare an object called P_R
using request.get_json()
, then we will use it as a key to define some values and pass them inside the jsonify()
method. These values should be just regular variables.
def POST_REQ_DATA():
P_R = request.get_json()
company_name = P_R["company_name"]
location = P_R["location"]
userlist = P_R["userlist"]
return jsonify(
{
"Post_Request": "Sent",
"company_name": company_name,
"location": location,
"userlist": userlist[1],
}
)
We will go back to the Postman, click the Body tab, and switch to Raw, then make sure the JSON application is selected in this drop-down list because it is the Jason mime-type. Now we can write JSON queries here and hit the Send
button after feeding our JSON data; the response is shown below.
The complete source code of our Flask app is shown below:
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route("/post-data", methods=["POST"])
def POST_REQ_DATA():
P_R = request.get_json()
company_name = P_R["company_name"]
location = P_R["location"]
userlist = P_R["userlist"]
return jsonify(
{
"Post_Request": "Sent",
"company_name": company_name,
"location": location,
"userlist": userlist[1],
}
)
# We feed the follwing data inside the postman
# {'company_name':'Delftstack','location':'Netherland','userlist':['Harry Parker','Goerge Wilson']}
if __name__ == "__main__":
app.run(debug=True)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn